How to Add Image in Android Jetpack Compose
Jetpack Compose, Android’s modern, declarative UI toolkit, has been revolutionizing the way we create user interfaces. As a key part of any UI, images need to be handled with care and Jetpack Compose certainly makes it simpler and more intuitive than ever before.
This tutorial will walk you through how to display images in your Jetpack Compose app.
First of all, import an image to your Android project using Android Studio.
In Jetpack Compose, the Image composable is used to display images. It requires a painter which handles the image loading and a contentDescription which is an essential aspect of making your app accessible.
Here’s a simple @Composable function that loads and displays our dog image:
@Composable
fun ImageExample() {
Column {
Image(modifier = Modifier.size(300.dp),
painter = painterResource(id = R.drawable.dog),
contentDescription = "Cartoon of dog")
}
}
Here, painterResource is a handy function that helps us load drawable resources. It takes the resource id of our image (R.drawable.dog) and returns a Painter that we can provide to the Image composable.
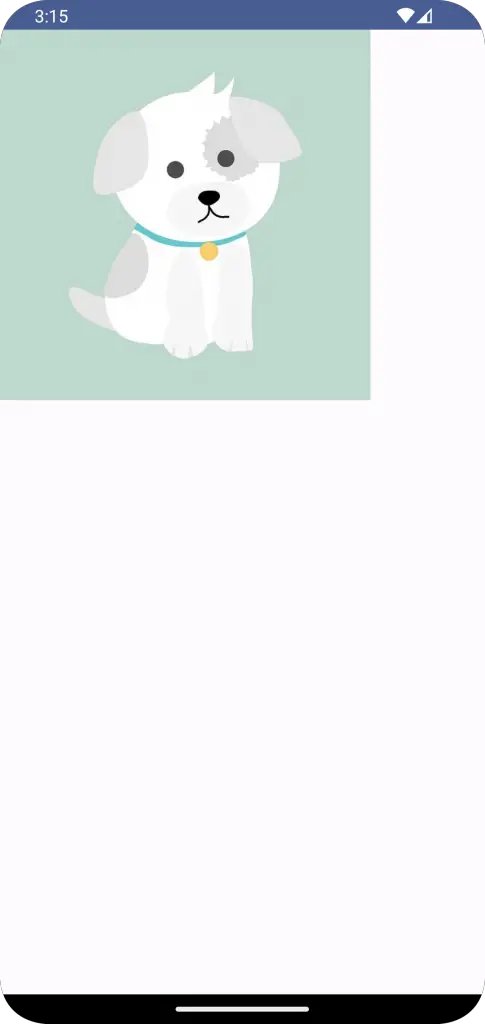
Following is the complete code to show the image.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.Image
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.size
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.res.painterResource
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
ImageExample()
}
}
}
}
}
@Composable
fun ImageExample() {
Column {
Image(modifier = Modifier.size(300.dp),
painter = painterResource(id = R.drawable.dog),
contentDescription = "Cartoon of dog")
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
ImageExample()
}
}
And voila! That’s all there is to displaying images in Jetpack Compose. It’s remarkably simpler and more intuitive compared to traditional methods. Hopefully, this guide has helped you understand how to display images in Jetpack Compose.
10 Comments