How to set Image Tint Color in Android Jetpack Compose
Jetpack Compose is a modern, reactive, and declarative framework for building efficient and beautiful UI for Android. It is easy to create and customize the look of your app with Jetpack Compose. In this blog post, let’s learn how to change the image tint color in Jetpack Compose.
The Image composable is used to display images with customization. It has a colorFilter parameter that helps to change the tint color. See the code snippet given below.
Image(painter = painterResource(id = R.drawable.tattoo),
contentDescription = null,
colorFilter = ColorFilter.tint(Color.Red)
)
I am using the following image in this Jetpack Compose tutorial. I want to change its tint color to red.
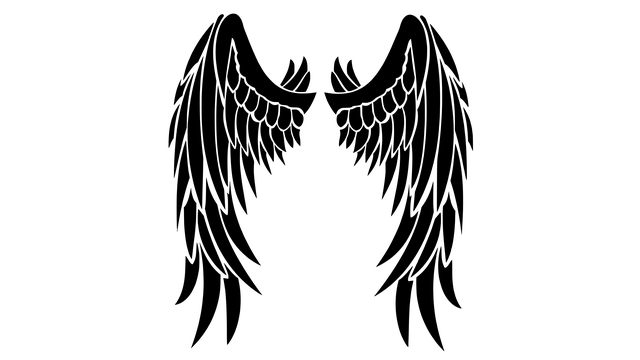
Following is the complete code.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.Image
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.graphics.ColorFilter
import androidx.compose.ui.res.painterResource
import androidx.compose.ui.tooling.preview.Preview
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
ImageExample()
}
}
}
}
}
@Composable
fun ImageExample() {
Image(painter = painterResource(id = R.drawable.tattoo),
contentDescription = null,
colorFilter = ColorFilter.tint(Color.Red),
modifier = Modifier.fillMaxSize()
)
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
ImageExample()
}
}
And you will get the following output with red tint color.
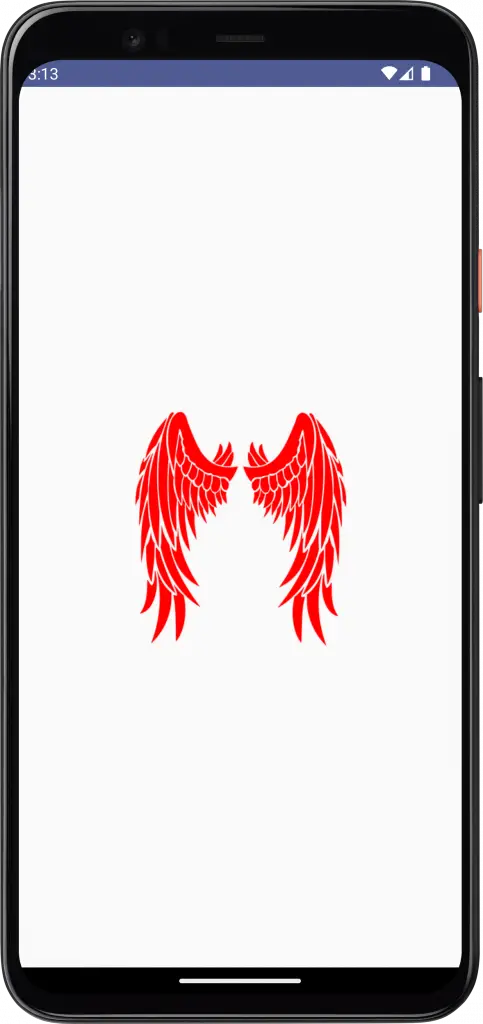
That’s how you change the image tint color in Jetpack Compose. I hope this tutorial is helpful for you.