How to Draw Paths in Jetpack Compose
Jetpack Compose provides a powerful and flexible API for drawing custom vector graphics and shapes. Using the Canvas
composable and path drawing functions like drawPath()
, you can create anything from simple lines and rectangles to complex polygons and curved shapes.
The Basics of Draw Path
To draw a path in Compose, you need to use the drawPath()
function on a Canvas
. This accepts a Path
instance which defines the actual shape vertices to draw:
Canvas(modifier = Modifier.fillMaxSize()) {
drawPath(
path = myPath,
color = Color.Red
)
}
The Path
is made up of a sequence of lines, curves, and other vector shape commands. For example:
val trianglePath = Path().apply {
moveTo(0f, 0f)
lineTo(100f, 0f)
lineTo(50f, 50f)
close()
}
Here we have defined a simple triangle by specifying the start and end points of each “leg”. The close()
command joins up the last vertex with the first to complete the shape.
You can then draw this with:
Canvas(modifier = Modifier.fillMaxSize()) {
drawPath(
path = trianglePath,
color = Color.Red
)
}
This gives you incredible flexibility over specifying exactly what shapes you wish to draw.
Following is the complete code.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.Canvas
import androidx.compose.ui.graphics.Path
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
TrianglePath()
}
}
}
}
}
@Composable
fun TrianglePath() {
val trianglePath = Path().apply {
moveTo(0f, 0f)
lineTo(500f, 0f)
lineTo(250f, 250f)
close()
}
Canvas(modifier = Modifier.fillMaxSize()) {
drawPath(
path = trianglePath,
color = Color.Red
)
}
}
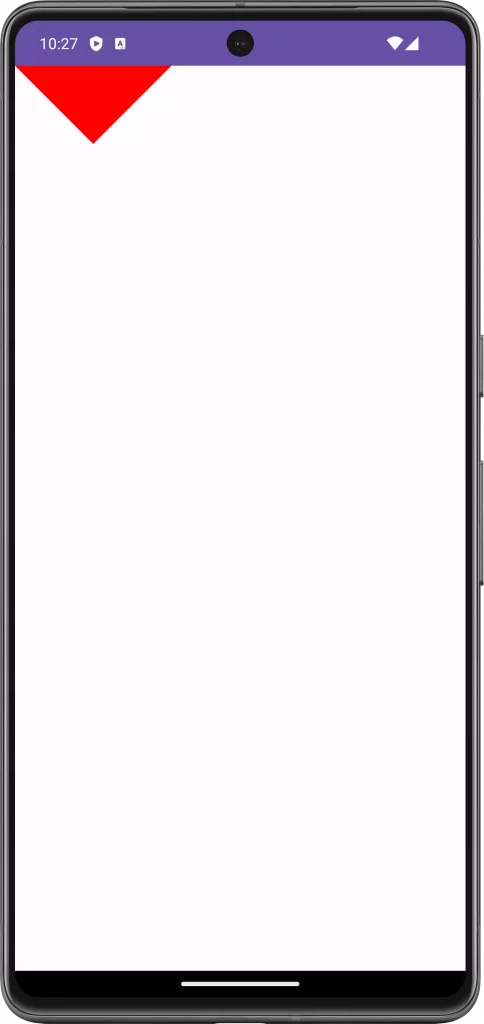
Fill and Stroke
By default drawPath()
will stroke fill the shape using a solid color. Use the style
parameter to fill or add a stroke.
drawPath(
path = myPath,
color = Color.Red,
style = Fill
)
When adding a stroke you can also customize its width.
@Composable
fun TrianglePath() {
val trianglePath = Path().apply {
moveTo(0f, 0f)
lineTo(500f, 0f)
lineTo(250f, 250f)
close()
}
Canvas(modifier = Modifier.fillMaxSize()) {
drawPath(
path = trianglePath,
color = Color.Red,
style = Stroke(width = 5f)
)
}
}
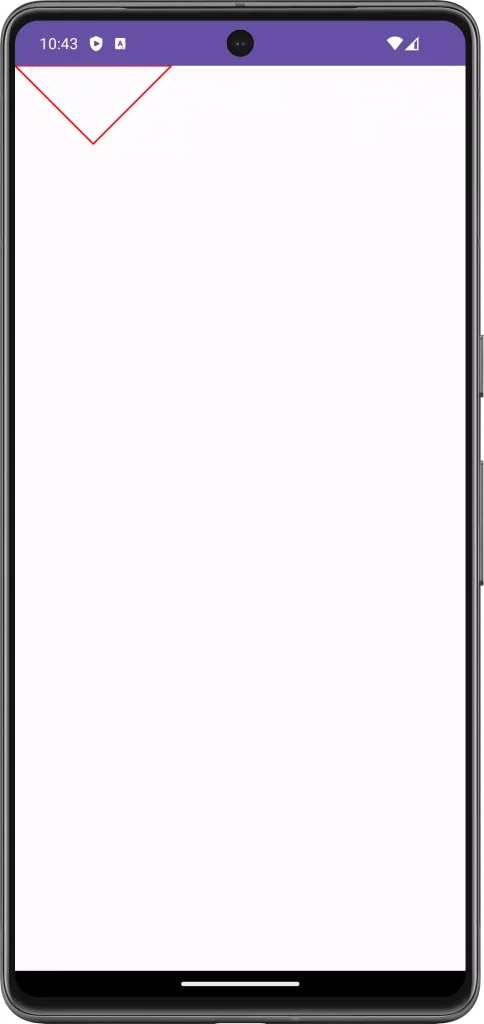
In this post, we’ve explored how to leverage Jetpack Compose’s vector drawing capabilities to render custom paths.
Path drawing is an essential tool for any Compose developer looking to build rich visual apps with dynamic graphic elements. Mastering it unlocks an exciting world of data visualization, animated graphics, interactive diagrams, maps, illustrations, and more.
Let me know in the comments about any cool path drawing examples you have built or want to see tutorials on!
One Comment