How to Draw Circle Using Canvas in Android Jetpack Compose
Canvas is the fundamental building block for creating custom graphics in Compose. It is added to the layout in the same manner as any other Compose UI component. Inside the Canvas, you can draw elements with exact control over their appearance and positioning.
In this blog post, let’s learn how to draw a color-filled circle using Canvas in Jetpack Compose.
You can make use of the drawCircle() function for this. See the code snippet given below.
Canvas(modifier = Modifier.fillMaxSize()) {
val canvasWidth = size.width
val canvasHeight = size.height
drawCircle(
color = Color.Red,
center = Offset(x = canvasWidth / 2, y = canvasHeight / 2),
radius = size.minDimension / 4
)
}
This creates a Canvas object and sets its modifier property to Modifier.fillMaxSize(), which means the Canvas will take up the maximum available space.
It then defines two variables canvasWidth and canvasHeight that are set to the width and height of the canvas, respectively.
It then uses the drawCircle function to draw a circle on the canvas. This function takes in several parameters, including color, center, and radius.
The color is set to red, the center is set to the center of the canvas by using the canvasWidth / 2 and canvasHeight / 2 for x and y coordinates respectively, and the radius is set to size.minDimension / 4, meaning that the radius of the circle is 1/4th of the minimum dimension of the canvas.
In summary, this code creates a canvas that takes up the maximum available space and draws a red circle in the center of the canvas with a radius that is 1/4th of the minimum dimension of the canvas.
You will get the following red circle as output.
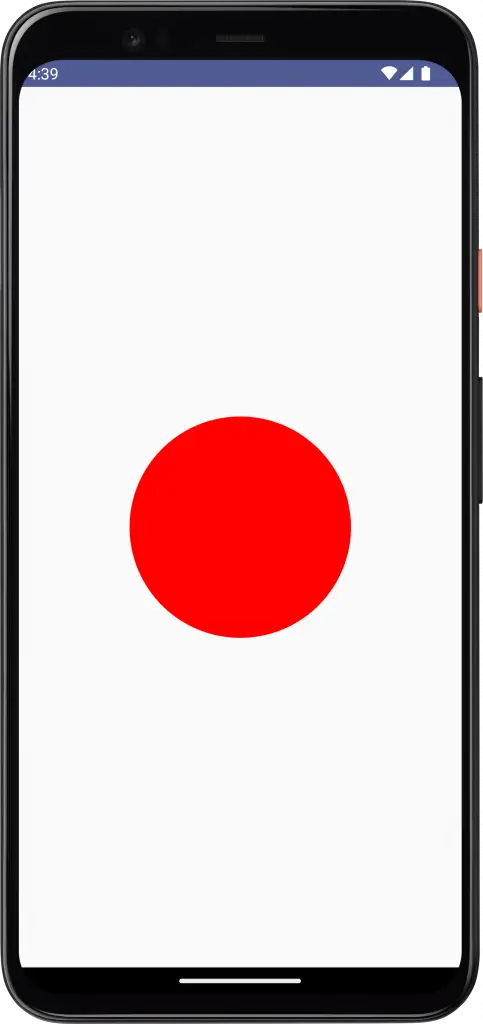
Following is the complete code for reference.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.Canvas
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.geometry.Offset
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
CanvasExample()
}
}
}
}
}
@Composable
fun CanvasExample() {
Canvas(modifier = Modifier.fillMaxSize()) {
val canvasWidth = size.width
val canvasHeight = size.height
drawCircle(
color = Color.Red,
center = Offset(x = canvasWidth / 2, y = canvasHeight / 2),
radius = size.minDimension / 4
)
}
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
CanvasExample()
}
}
That’s how you draw a circle on Canvas in Jetpack Compose.
One Comment