How to Add Scroll to Bottom Button with LazyColumn in Android Jetpack Compose
Navigating through long lists can be cumbersome for users. Fortunately, Jetpack Compose’s LazyColumn component provides us with handy features that can improve this user experience. In this tutorial, we will cover how to add a Scroll to Bottom button to a LazyColumn in Jetpack Compose.
The Basics of LazyColumn
LazyColumn is a component in Jetpack Compose that renders only the visible items in a vertical list. This allows us to efficiently display large sets of data without putting a strain on the device’s resources.
LazyColumn with Scroll to Bottom Button
In order to add a Scroll to Bottom button, we need to get a reference to the state of the LazyColumn. We will use this state to control the scroll position. Then we use the animateScrollToItem function to smoothly scroll to the end of the list. Here is an example:
@Composable
fun ScrollableColumnExample() {
val coroutineScope = rememberCoroutineScope()
val listState = rememberLazyListState()
Box(modifier = Modifier.fillMaxSize()) {
LazyColumn(state = listState) {
items(100) { item ->
Text(text = "Item $item", modifier = Modifier.fillMaxWidth())
}
}
Button(onClick = {
coroutineScope.launch {
listState.animateScrollToItem(index = listState.layoutInfo.totalItemsCount - 1)
}
},
modifier = Modifier
.align(Alignment.BottomCenter)
.padding(16.dp)) {
Text("Scroll to bottom")
}
}
}
n the example above, the Box Composable is used to layer the Button and LazyColumn. Inside the Box, we use the LazyColumn Composable to display a list of items. The “Scroll to bottom” button is then positioned at the bottom center of the Box, above the LazyColumn.
When the button is clicked, we launch a coroutine to call animateScrollToItem, passing the index of the last item (total items count – 1) to smoothly scroll to the end of the list.
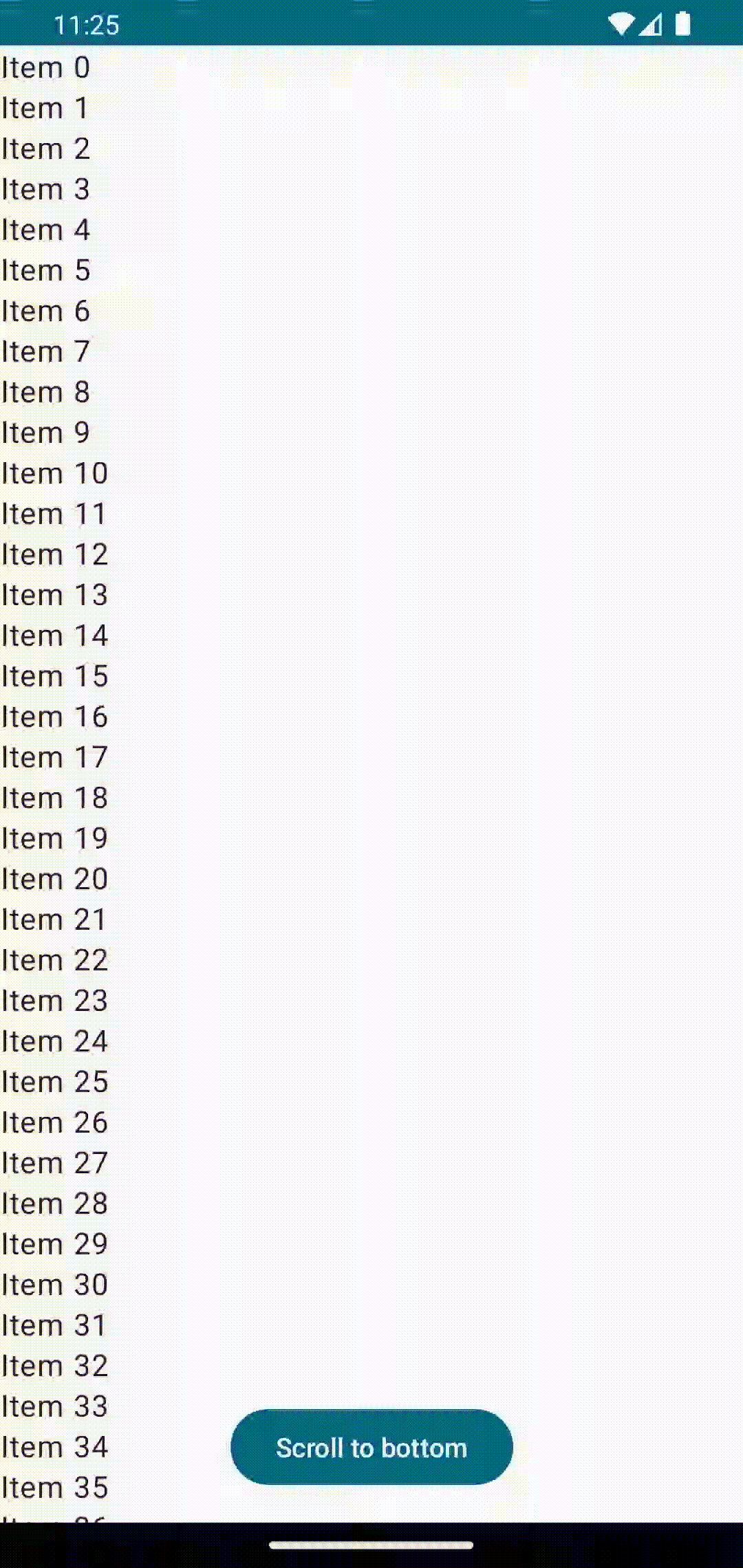
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Box
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.padding
import androidx.compose.foundation.lazy.LazyColumn
import androidx.compose.foundation.lazy.rememberLazyListState
import androidx.compose.material3.Button
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.runtime.rememberCoroutineScope
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import com.example.example.ui.theme.ExampleTheme
import kotlinx.coroutines.launch
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
ScrollableColumnExample()
}
}
}
}
}
@Composable
fun ScrollableColumnExample() {
val coroutineScope = rememberCoroutineScope()
val listState = rememberLazyListState()
Box(modifier = Modifier.fillMaxSize()) {
LazyColumn(state = listState) {
items(100) { item ->
Text(text = "Item $item", modifier = Modifier.fillMaxWidth())
}
}
Button(onClick = {
coroutineScope.launch {
listState.animateScrollToItem(index = listState.layoutInfo.totalItemsCount - 1)
}
},
modifier = Modifier
.align(Alignment.BottomCenter)
.padding(16.dp)) {
Text("Scroll to bottom")
}
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
ScrollableColumnExample()
}
}
Now, you can add a “Scroll to Bottom” button in your Jetpack Compose apps with ease! This will certainly enhance the user experience, especially when dealing with long lists.