How to Set Background Image for Card in Android Jetpack Compose
Jetpack Compose is a powerful UI toolkit that enables Android developers to build beautiful user interfaces more efficiently. Among the wide array of composables it offers is the Card composable, which is used frequently in modern UI design.
One common requirement for cards is the ability to set a background image. In this blog post, we will walk through the steps to set a background image for a Card in Jetpack Compose.
The Card Composable
In Jetpack Compose, the Card composable is a material design implementation that contains content and actions about a single subject. It acts as a container and can hold any other composable.
How to Add Background Image to Card
To add a background image to a card, we use a combination of composables: Card, Box, and Image. Here is how you can do it:
@Composable
fun CardExample() {
Column {
Card(
modifier = Modifier
.fillMaxWidth()
.padding(20.dp)
) {
Box(
Modifier
.height(150.dp)
.fillMaxWidth()
) {
Image(
painter = painterResource(id = R.drawable.nature),
modifier = Modifier.fillMaxSize(),
contentDescription = "Background Image",
contentScale = ContentScale.Crop
)
Text("Hello from Jetpack Compose!", Modifier.padding(16.dp))
}
}
}
}
In the code above, the Card is used as a container for a Box composable, which in turn holds an Image and Text composable. The Box composable is a layout composable that stacks its children, which allows us to place the Text composable over the Image composable.
Following is the output.
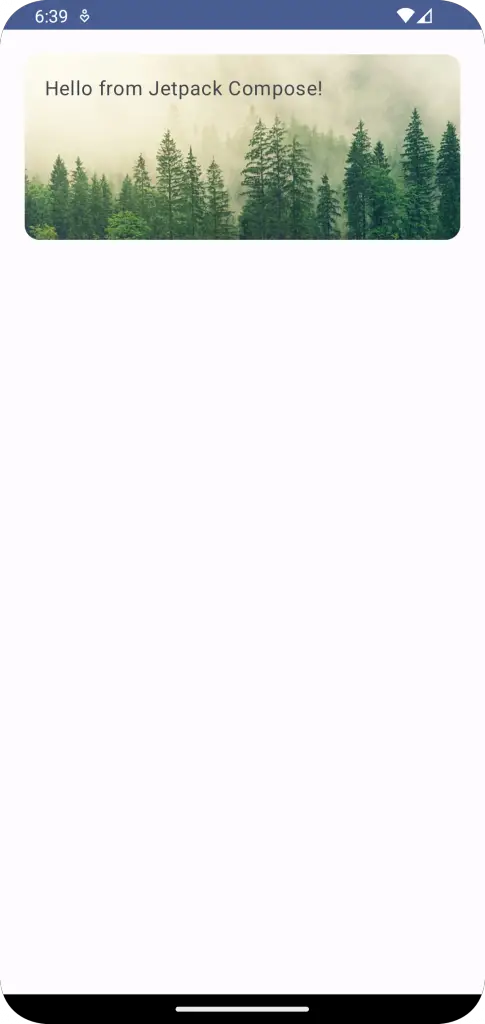
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.Image
import androidx.compose.foundation.layout.Box
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.height
import androidx.compose.foundation.layout.padding
import androidx.compose.material3.Card
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.layout.ContentScale
import androidx.compose.ui.res.painterResource
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
CardExample()
}
}
}
}
}
@Composable
fun CardExample() {
Column {
Card(
modifier = Modifier
.fillMaxWidth()
.padding(20.dp)
) {
Box(
Modifier
.height(150.dp)
.fillMaxWidth()
) {
Image(
painter = painterResource(id = R.drawable.nature),
modifier = Modifier.fillMaxSize(),
contentDescription = "Background Image",
contentScale = ContentScale.Crop
)
Text("Hello from Jetpack Compose!", Modifier.padding(16.dp))
}
}
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
CardExample()
}
}
Jetpack Compose makes it easy to create beautiful UIs with complex requirements. Adding a background image to a Card, for instance, can be accomplished in just a few lines of code.