How to Create a Vertical Slider in Jetpack Compose
Jetpack Compose, the modern toolkit for UI development in Android, offers a diverse range of components to build interactive and visually appealing interfaces. While horizontal sliders are commonly used for adjustments like volume control, there are scenarios where a vertical orientation is more suitable.
This blog post will guide you through creating a vertical slider in Jetpack Compose, enhancing the functionality and aesthetic of your app’s UI.
The Concept of a Vertical Slider
Vertical sliders are an excellent choice for apps with vertical layouts or when you need to save horizontal space. They allow users to input or adjust values through a vertical dragging motion, which can be more intuitive in certain contexts.
Vertical Slider in Jetpack Compose
Creating a vertical slider in Jetpack Compose involves rotating the standard horizontal slider. Here’s how you can implement it:
@Composable
fun MyVerticalSlider() {
var sliderValue by remember { mutableStateOf(0f) }
Box(contentAlignment = Alignment.Center, modifier = Modifier.fillMaxSize()) {
Slider(
value = sliderValue,
onValueChange = { sliderValue = it },
valueRange = 0f..100f,
modifier = Modifier.rotate(-90f)
)
}
}
Breaking Down the Code
Box(contentAlignment = Alignment.Center, modifier = Modifier.fillMaxSize())
: This creates aBox
layout that fills the maximum size available and aligns its children to the center. It acts as the container for our slider.var sliderValue by remember { mutableStateOf(0f) }
: This line sets up the state management for the slider’s value. The slider starts with an initial value of0f
.Slider(...)
: This is the standard horizontal slider composable. It’s configured to represent a range from0f
to100f
.modifier = Modifier.rotate(-90f)
: The critical part of this implementation. This modifier rotates the slider by -90 degrees, effectively changing its orientation from horizontal to vertical.
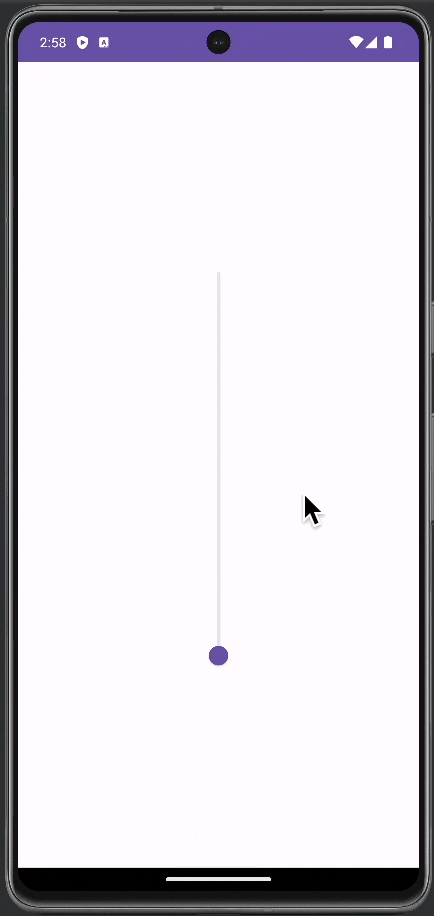
Following is the complete code for reference.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Box
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Slider
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.runtime.getValue
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.setValue
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.draw.rotate
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
MyVerticalSlider()
}
}
}
}
}
@Composable
fun MyVerticalSlider() {
var sliderValue by remember { mutableStateOf(0f) }
Box(contentAlignment = Alignment.Center, modifier = Modifier.fillMaxSize()) {
Slider(
value = sliderValue,
onValueChange = { sliderValue = it },
valueRange = 0f..100f,
modifier = Modifier.rotate(-90f)
)
}
}
Customize the Vertical Slider
Styling and Appearance
You can customize the vertical slider’s thumb size, track color, and more, using Jetpack Compose’s styling parameters. This allows you to make the slider align with your app’s design theme.
User Experience Considerations
- Intuitiveness: Ensure that the purpose and functionality of the vertical slider are intuitive to the user.
- Responsiveness: Test the slider on various devices and orientations to ensure it performs consistently.
A vertical slider in Jetpack Compose can be a valuable addition to your Android app, particularly for apps that require an adaptable approach to UI design. With the flexibility of Jetpack Compose, creating a vertical slider that is both functional and visually appealing is straightforward and effective.