How to Show List with Divider in Android Jetpack Compose.
Showing Lists using Jetpack Compose is relatively easy. Having a divider between the items of the list makes the UI neat and clean. Let’s learn how to show lists with a divider between items in Android Jetpack Compose.
LazyColumn composable is used to show lists in a more efficient way. There is no built-in way to show a divider between items. Hence, we have to render a divider for each item. See the code snippet given below.
@Composable
fun TextList() {
val listA = listOf<String>("Example", "Android", "Tutorial", "Jetpack", "Compose", "List", "Example","Simple")
LazyColumn(
modifier = Modifier.fillMaxWidth(),
contentPadding = PaddingValues(16.dp)
) {
items(listA) { item ->
Text(modifier = Modifier.padding(20.dp), text = item)
Divider(color = Black, thickness = 0.5.dp)
}
}
}
The output will be as given below.
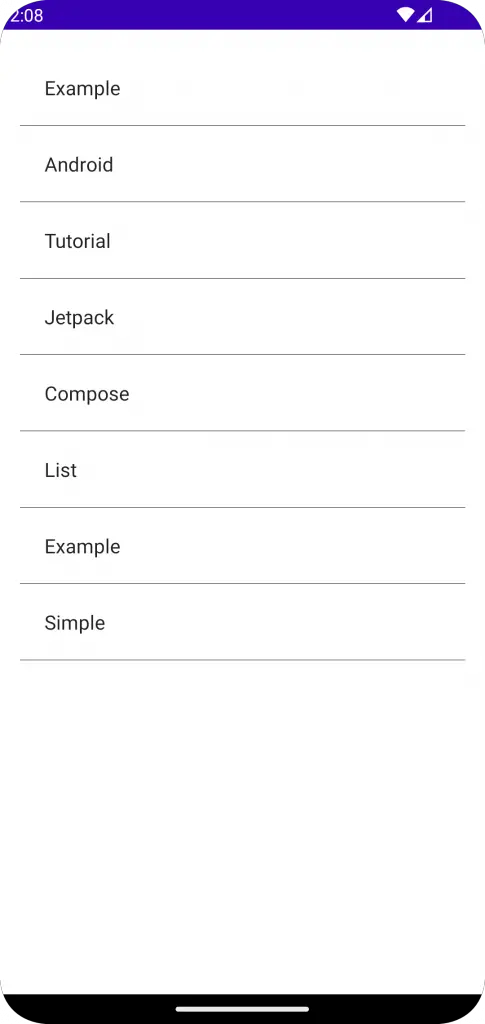
As you see, the last item on the list also has a Divider. You can get rid of the last divider from the list by using itemsIndexed instead of items. See the code snippet given below.
@Composable
fun TextList() {
val listA = listOf<String>("Example", "Android", "Tutorial", "Jetpack", "Compose", "List", "Example","Simple")
LazyColumn(
modifier = Modifier.fillMaxWidth(),
contentPadding = PaddingValues(16.dp)
) {
itemsIndexed(listA) { index,item ->
Text(modifier = Modifier.padding(20.dp), text = item)
if (index < listA.lastIndex)
Divider(color = Black, thickness = 0.5.dp)
}
}
}
Now, you will get the following output.
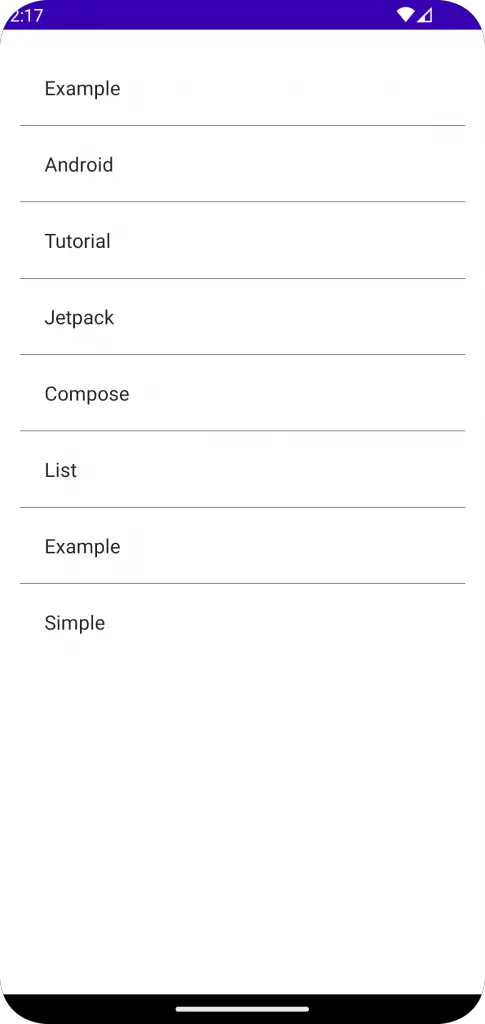
That’s how you remove the last divider from the List in Jetpack Compose.
Following is the complete code for reference.
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.PaddingValues
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.padding
import androidx.compose.foundation.lazy.LazyColumn
import androidx.compose.foundation.lazy.items
import androidx.compose.material3.Divider
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color.Companion.Black
import androidx.compose.ui.unit.dp
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
ListExample()
}
}
}
}
}
}
@Composable
fun ListExample() {
val listA = listOf<String>("Example", "Android", "Tutorial", "Jetpack", "Compose", "List", "Example","Simple")
LazyColumn(
modifier = Modifier.fillMaxWidth(),
contentPadding = PaddingValues(16.dp)
) {
items(listA) { item ->
Text(modifier = Modifier.padding(20.dp), text = item)
Divider(color = Black, thickness = 0.5.dp)
}
}
}
I hope this Jetpack Compose List tutorial is helpful for you. Thank you for reading.