How to Create a Simple List in Android Jetpack Compose
Most mobile apps need a way to display lists. Whether you’re showing a feed of social media posts or a list of settings, knowing how to implement this is crucial. In this Jetpack Compose tutorial, you’ll learn how to create a straightforward list of text items in your Android app.
Why LazyColumn?
LazyColumn
and LazyRow
are two special Jetpack Compose composables that are optimized for displaying lists. What sets them apart is their efficiency. They only render items that are currently visible on the screen, making them highly efficient.
The Basic Code Structure
Here is a simple example using LazyColumn
to display a list of strings:
@Composable
fun ListExample() {
val listA = listOf<String>("Example", "Android", "Tutorial", "Jetpack", "Compose", "List", "Example","Simple", "List")
LazyColumn(
modifier = Modifier.fillMaxWidth(),
contentPadding = PaddingValues(16.dp)
) {
items(listA) { item ->
Spacer(modifier = Modifier.height(100.dp))
Text(text = item)
}
}
}
In this example, I declare a list of strings called listA
. The LazyColumn
composable takes care of displaying each item in this list.
Modifier.fillMaxWidth()
makes sure that theLazyColumn
takes the full width of the parent layout.PaddingValues(16.dp)
adds a 16 dp padding around theLazyColumn
.items(listA) { item -> ... }
iterates through each item in the listlistA
.Spacer
andText
composables render for each item, separated by a height of 100.dp.
Complete Code With MainActivity
If you’d like to see how to use this ListExample
function in your MainActivity
, check out the complete code example below:
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.PaddingValues
import androidx.compose.foundation.layout.Spacer
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.height
import androidx.compose.foundation.lazy.LazyColumn
import androidx.compose.foundation.lazy.items
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.unit.dp
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
ListExample()
}
}
}
}
}
}
@Composable
fun ListExample() {
val listA = listOf<String>("Example", "Android", "Tutorial", "Jetpack", "Compose", "List", "Example","Simple", "List")
LazyColumn(
modifier = Modifier.fillMaxWidth(),
contentPadding = PaddingValues(16.dp)
) {
items(listA) { item ->
Spacer(modifier = Modifier.height(100.dp))
Text(text = item)
}
}
}
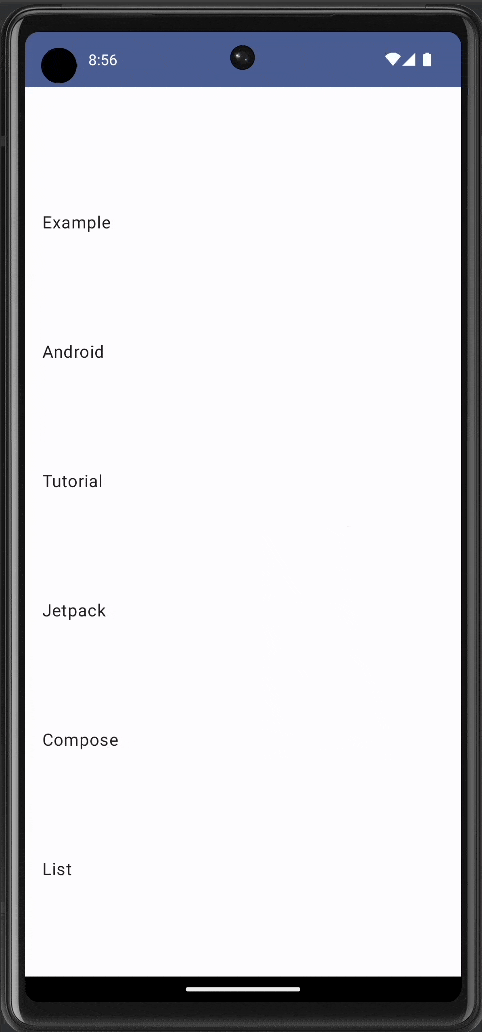
Creating lists in Android has never been easier, thanks to Jetpack Compose. By using LazyColumn
, you can display lists in an efficient and straightforward manner. I hope you find this tutorial helpful for your Android app development journey.
2 Comments