How to Use LazyColumn in Android Jetpack Compose
Jetpack Compose, Google’s innovative UI toolkit, has revolutionized the way we build user interfaces in Android. Among the numerous features it offers, the LazyColumn function stands out.
It simplifies the creation of lists, which are an essential part of many apps. This post explores LazyColumn in detail and provides practical examples of its usage.
What is LazyColumn?
LazyColumn is a Composable function used to render large lists efficiently by only rendering the visible items on the screen (also known as ‘lazy loading’ or ‘virtualization’). This makes it ideal for displaying long lists, where performance can be a concern.
How to Use LazyColumn
Let’s start with a basic example. In this case, we want to display a list of 1000 numbers.
@Composable
fun NumbersList() {
LazyColumn {
items(1000) { index ->
Text(text = "Item $index")
}
}
}
In the code snippet above, items function is used to generate a list with 1000 items. The lambda function passed as a parameter to items gets called only for the items that are currently visible on the screen, greatly improving performance.
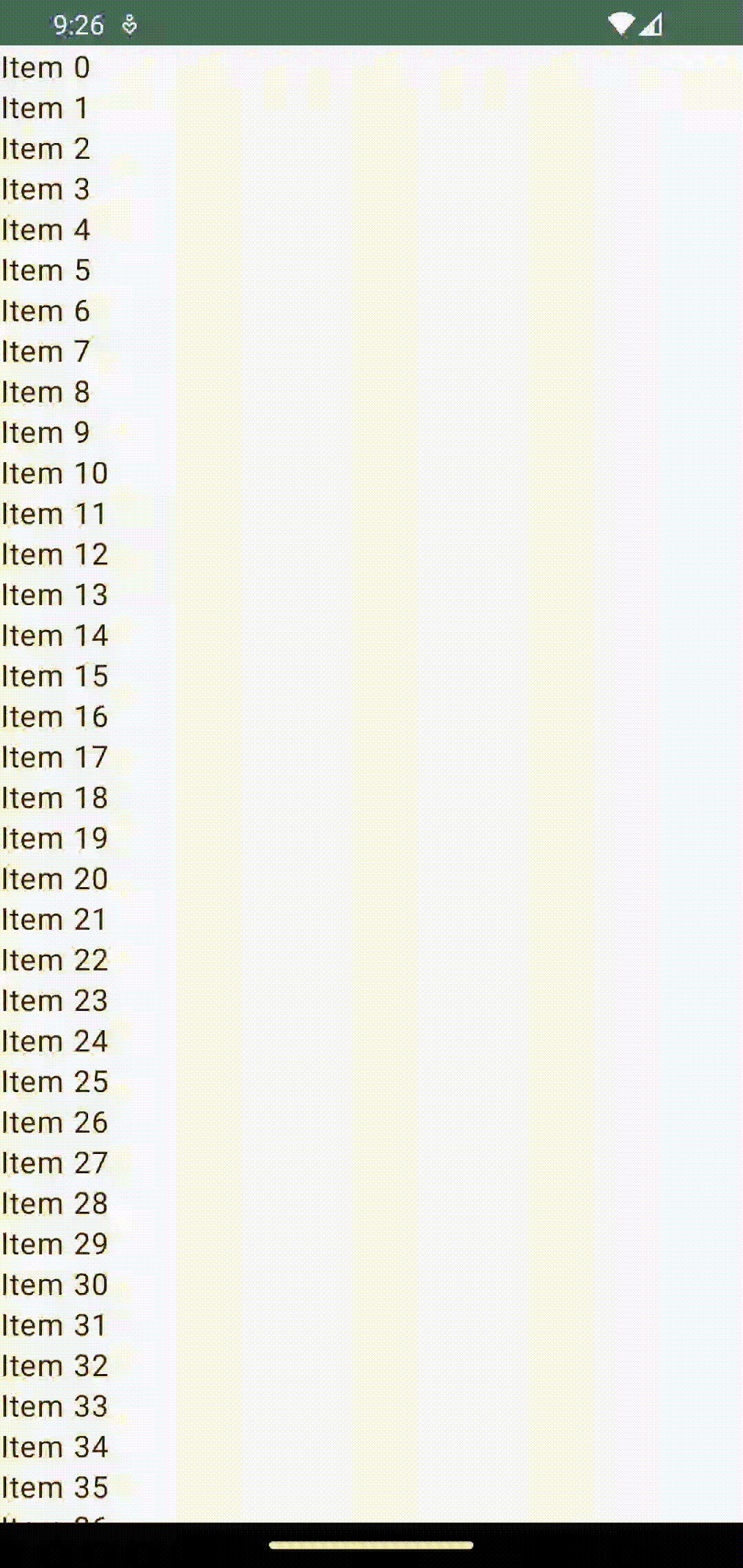
Handle Click Events in LazyColumn
It’s quite common to have click events for items in a list. In LazyColumn, handling click events is straightforward. Here’s how you would implement a click listener on list items:
@Composable
fun NumbersList() {
LazyColumn {
items(1000) { index ->
Text(
text = "Item $index",
modifier = Modifier.clickable {
println("Item $index clicked")
}
)
}
}
}
The Modifier.clickable function is used to listen for click events on the Text Composable.
How to Display a List of Custom Objects
You’re not just limited to displaying a list of primitives such as integers or strings. You can display a list of custom objects too. Let’s take a look at an example where we display a list of Person objects:
data class Person(val name: String, val age: Int)
@Composable
fun MyApp() {
val people = listOf(
Person("John Doe", 25),
Person("Jane Smith", 30),
Person("Alice Johnson", 20),
Person("Bob Williams", 27)
)
PersonList(people)
}
@Composable
fun PersonList(people: List<Person>) {
LazyColumn {
items(people.size) {index ->
val person = people[index]
Text(text = "Name: ${person.name}, Age: ${person.age}")
}
}
}
We declare a data class Person in Kotlin. This class represents a Person entity and contains two properties: name and age. The LazyColumn creates an item for each entry in the people list.
For simplicity, you may use items extension to reduce verbrose.
import androidx.compose.foundation.lazy.LazyColumn
import androidx.compose.foundation.lazy.items
data class Person(val name: String, val age: Int)
@Composable
fun MyApp() {
val people = listOf(
Person("John Doe", 25),
Person("Jane Smith", 30),
Person("Alice Johnson", 20),
Person("Bob Williams", 27)
)
PersonList(people)
}
@Composable
fun PersonList(people: List<Person>) {
LazyColumn {
items(people) { person ->
Text(text = "Name: ${person.name}, Age: ${person.age}")
}
}
}
In this blog post, we’ve covered the basics of using LazyColumn in Jetpack Compose, including how to create a simple list, how to handle click events, and how to display a list of custom objects. With these skills, you’re well on your way to efficiently displaying lists in your Jetpack Compose app.
One Comment