How to add Toggle Button in Android Jetpack Compose
The toggle button or the switch button is a UI component through which users can turn on or off a particular option. The toggle button is always seen on the settings screen. Let’s learn how to add a simple switch button in Android using Jetpack Compose.
The Switch in compose helps to create a toggle button. It accepts parameters such as checked, onCheckedChange, modifier, enabled, colors, etc. Following is the code snippet to add Switch.
@Composable
fun SwitchExample() {
var checked by remember { mutableStateOf(true) }
Switch(
checked = checked,
onCheckedChange = { checked = it },
modifier = Modifier.padding(10.dp)
)
}
Following is the output.
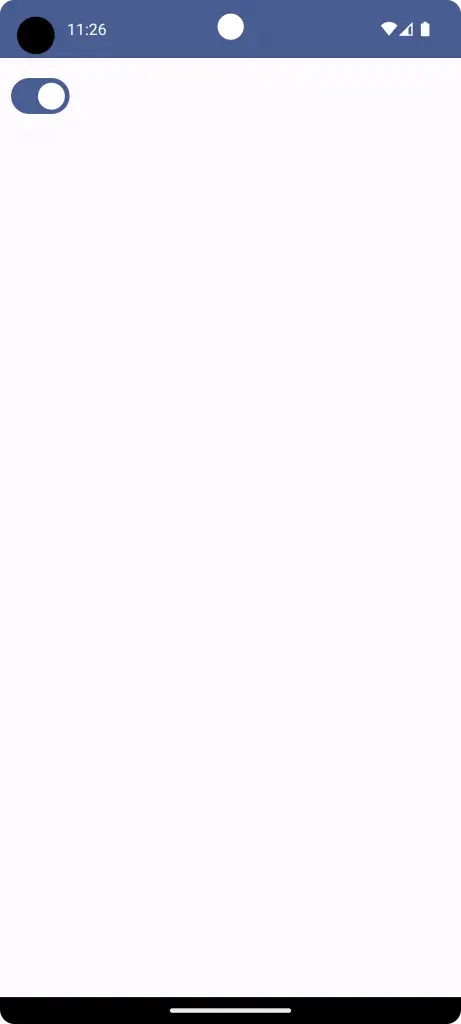
Following is the complete code for reference.
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.padding
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Switch
import androidx.compose.runtime.Composable
import androidx.compose.runtime.getValue
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.setValue
import androidx.compose.ui.Modifier
import androidx.compose.ui.unit.dp
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
SwitchExample()
}
}
}
}
}
}
@Composable
fun SwitchExample() {
var checked by remember { mutableStateOf(true) }
Switch(
checked = checked,
onCheckedChange = { checked = it },
modifier = Modifier.padding(10.dp)
)
}
That’s how you add a switch or toggle in Jetpack Compose.