How to Draw Text on Canvas in Jetpack Compose
Jetpack Compose, Android’s modern UI toolkit, offers extensive capabilities for custom UI design, including drawing text on a canvas. This feature is important for developers looking to create custom text layouts or integrate text within graphical elements.
This blog post provides an in-depth look at how to draw text on a canvas in Jetpack Compose, using a specific example for clarity.
In Jetpack Compose, the Canvas
composable is a powerful tool that provides a surface where you can perform custom drawing, including text. It gives developers the flexibility to create intricate and customized designs that are not possible with standard text composables.
Example: Draw Custom Text on Canvas
Let’s break down an example to understand how to draw text on a canvas in Jetpack Compose.
First, we define a Canvas
composable with the maximum available size for our drawing area.
@Composable
fun Example() {
Canvas(Modifier.fillMaxSize()) {
// Text drawing code will be added here
}
}
Jetpack Compose uses the TextMeasurer
to measure and layout text. buildAnnotatedString
is used to create a custom text layout.
val textMeasurer = rememberTextMeasurer()
The textMeasurer
variable is a tool that helps measure and layout the text within our canvas.
drawText(
textMeasurer = textMeasurer,
text = buildAnnotatedString {
withStyle(ParagraphStyle(textAlign = TextAlign.Start)) {
append("Hello")
}
withStyle(ParagraphStyle()) {
append("World")
}
}
)
In this drawText
function:
textMeasurer
: Specifies the text measurer to use for rendering the text.text
: Defines the actual text to be drawn and its styles.buildAnnotatedString
is used to build a string with different styles.withStyle(ParagraphStyle(textAlign = TextAlign.Start)) { append("Hello") }
: Creates a part of the string “Hello” with a style that aligns the text to the start.withStyle(ParagraphStyle()) { append("World") }
: Adds “World” to the string.
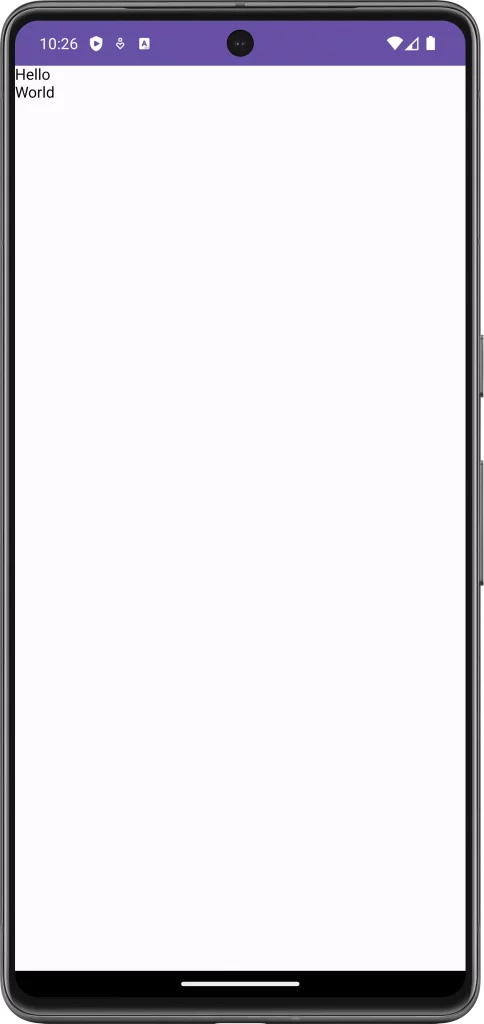
Following is the complete code for reference.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.Canvas
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.text.ParagraphStyle
import androidx.compose.ui.text.buildAnnotatedString
import androidx.compose.ui.text.drawText
import androidx.compose.ui.text.rememberTextMeasurer
import androidx.compose.ui.text.style.TextAlign
import androidx.compose.ui.text.withStyle
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Example()
}
}
}
}
}
@Composable
fun Example() {
val textMeasurer = rememberTextMeasurer()
Canvas(Modifier.fillMaxSize()) {
drawText(
textMeasurer = textMeasurer,
text = buildAnnotatedString {
withStyle(ParagraphStyle(textAlign = TextAlign.Start)) {
append("Hello")
}
withStyle(ParagraphStyle()) {
append("World")
}
}
)
}
}
Drawing text on a canvas in Jetpack Compose allows for creative and flexible text rendering in your Android app. By mastering this technique, you can create custom text layouts and integrate text into your graphical designs, enhancing both the functionality and aesthetic of your app’s UI.