How to make Text Clickable in Android Jetpack Compose
In Android apps, it’s common to have text that responds to user interactions, such as clicking or tapping. In this Android development tutorial, let’s learn how to make text clickable in Jetpack Compose.
Usually, the text components of an app are non-clickable by default. Sometimes, you may want to add clickable text because of UI requirements.
Here, we use the modifier to make the Text composable clickable. The alternate way to make text pressable is to add a text button in Jetpack Compose.
See the code snippet given below.
@Composable
fun ClickableText() {
Text(
modifier = Modifier.clickable { println("Clicked") },
text ="This Text is clickable",
fontSize = 30.sp,
)
}
The modifier parameter is used to specify the behavior of the Text element. In this case, the clickable modifier is used to make the Text clickable. When the Text is clicked, the lambda expression { println(“Clicked”) } is executed, which prints “Clicked” to the console.
You will get the following output.
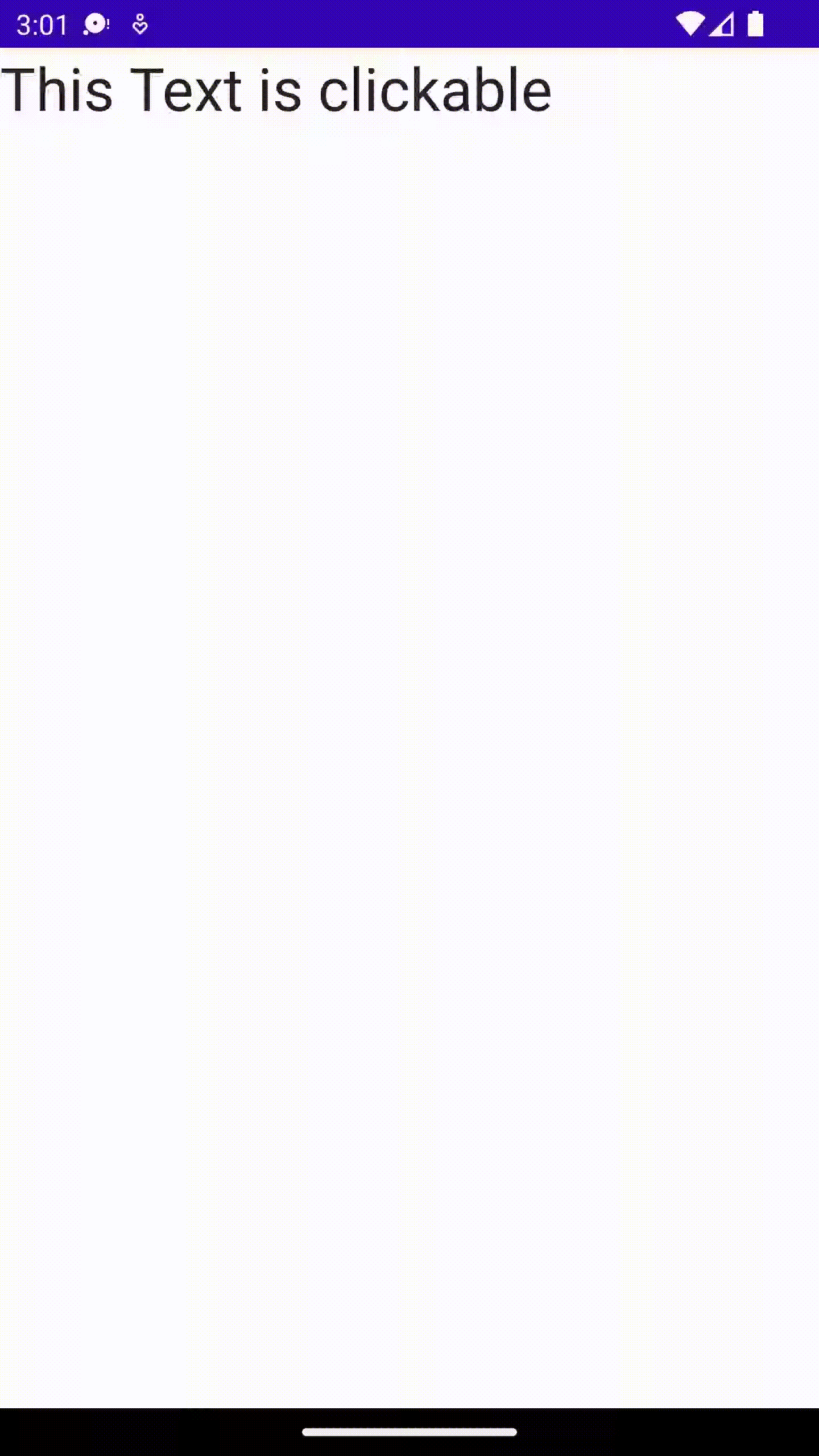
Following is the complete example code for reference.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.clickable
import androidx.compose.foundation.layout.*
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.*
import androidx.compose.ui.Modifier
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.sp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
ClickableText()
}
}
}
}
}
@Composable
fun ClickableText() {
Text(
modifier = Modifier.clickable { println("Clicked") },
text ="This Text is clickable",
fontSize = 30.sp,
)
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
ClickableText()
}
}
In this tutorial, we learned how to make text clickable and respond to user interactions in Android Jetpack Compose.
One Comment