How to add Text from strings.xml in Android Jetpack Compose
Jetpack Compose simplifies the UI development process by allowing developers to build UI components declaratively in Kotlin. Even though text can be added directly to a Compose function, it’s recommended to add text in the strings.xml file for localization and translation purposes.
A Text composable is a UI component for displaying text on the screen. It’s similar to a TextView in traditional Android development, but with the added benefits of being customizable and declarative.
With the Text composable, developers can easily style the text by specifying attributes such as font size, font style, color, and alignment. Let’s learn how to read values from the strings.xml file in Jetpack Compose.
Add your text in the strings.xml file and then You should use stringResource to call the reference. See the code snippet given below.
@Composable
fun ResourceText() {
Text(stringResource(R.string.example_text))
}
Following is the complete code for reference.
I added an example text in the strings.xml as given below.
<resources>
<string name="app_name">My Application</string>
<string name="example_text">Example Text</string>
</resources>
Then I called the string from the MainActivity.kt file.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.*
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.*
import androidx.compose.ui.Modifier
import androidx.compose.ui.res.stringResource
import androidx.compose.ui.tooling.preview.Preview
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
ResourceText()
}
}
}
}
}
@Composable
fun ResourceText() {
Text(stringResource(R.string.example_text))
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
ResourceText()
}
}
Then you will get the following output.
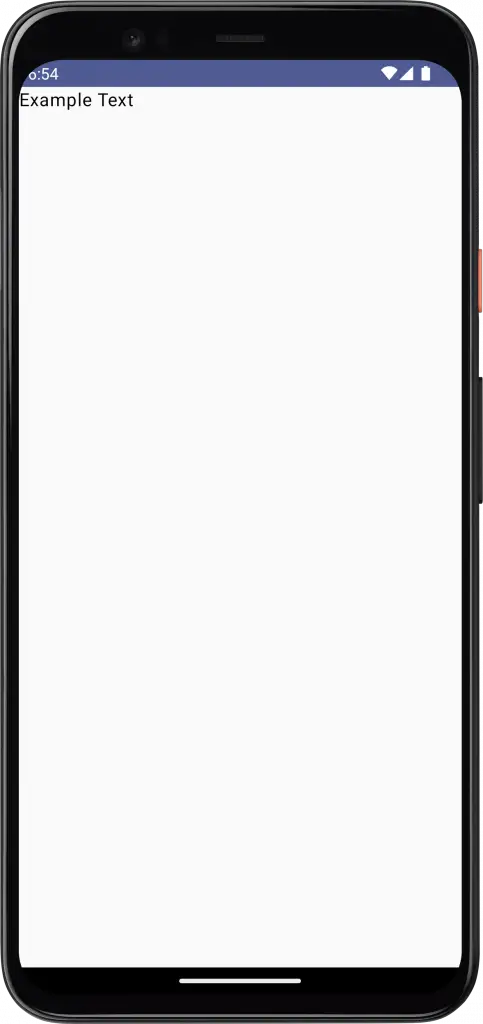
That’s how you show Text by referring to the string resource file in Jetpack Compose. I hope this short tutorial will help you. Thank you for reading!
3 Comments