How to set Maximum Lines of Text in Android Jetpack Compose
When designing an app, it is important to consider the text content and its visual appearance. Sometimes, the dynamic text content can be lengthy, which may affect the app design. In such cases, restricting the number of lines of text is a useful technique.
In this short Android development tutorial, let’s learn how to set the maximum lines of text in Jetpack Compose.
The Text composable has a property named maxLines that can be used to set maximum lines. By using TextOverflow.Ellipsis you can also add three dots at the end of the exceeded text.
See the code snippet given below.
@Composable
fun TextExample() {
Text( modifier = Modifier.padding(10.dp),
text = "The maximum number of line for this text is 1. It doesn't show the other lines and exceeded text.",
maxLines = 2,
overflow = TextOverflow.Ellipsis, fontSize = 20.sp)
}
The maxLines parameter is used to set the maximum number of lines that the Text element should display. In this case, the maxLines is set to 2.
The overflow parameter is used to specify what should happen if the text exceeds the maximum number of lines. In this case, TextOverflow.Ellipsis is used, which truncates the text and adds an ellipsis at the end of the last visible line.
Following is the output.
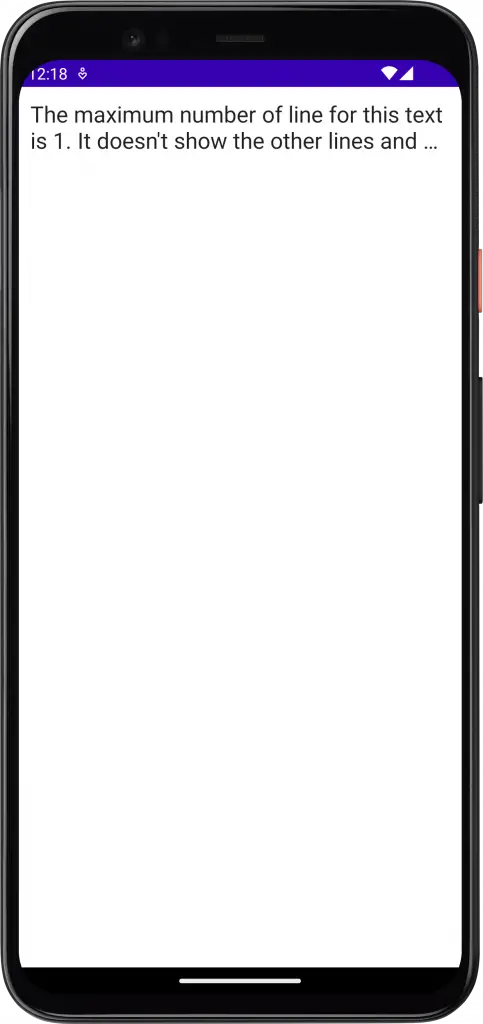
Following is the complete code for reference.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.*
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.*
import androidx.compose.ui.Modifier
import androidx.compose.ui.text.style.TextOverflow
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import androidx.compose.ui.unit.sp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
TextExample()
}
}
}
}
}
@Composable
fun TextExample() {
Text( modifier = Modifier.padding(10.dp),
text = "The maximum number of line for this text is 1. It doesn't show the other lines and exceeded text.",
maxLines = 2,
overflow = TextOverflow.Ellipsis, fontSize = 20.sp)
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
TextExample()
}
}
In this tutorial, we have learned how to set the maximum number of lines of text using Jetpack Compose. We have created a composable function using the Text composable and set the maximum number of lines to 2.
We have also added padding and used the TextOverflow.Ellipsis parameter to truncate the exceeded text with an ellipsis.