How to Create Scale Animation in Android Jetpack Compose
Using animations instead of static UI can add life to your mobile app. In this Android tutorial, let’s learn how to create scale animation in Jetpack Compose.
By scale animation, we intend to animate the size of a given composable. In this example, we use the animateDpAsState function to animate the size of a Box composable.
See the following code.
var sizeState by remember { mutableStateOf(50.dp) }
val size by animateDpAsState(targetValue = sizeState, tween(durationMillis = 1000,
easing = LinearEasing))
Column(Modifier.fillMaxSize(),
horizontalAlignment = Alignment.CenterHorizontally)
{
Button(onClick = { sizeState = sizeState*2 }) {
Text(text = "Click Here!")
}
Box(modifier = Modifier
.size(size)
.background(color = Color.Blue))
}
This code creates a user interface that consists of a button and a blue box that changes size with a scale animation when the button is clicked.
First, we declare a mutable state variable called sizeState and initialize it to a value of 50 dp. Then declare an immutable state variable called size and uses the animateDpAsState function to animate the size of the box to the value of sizeState.
The animation duration is 1000 milliseconds (1 second) and uses a linear easing function to make the animation smooth and consistent. The Button composable is created with the onClick parameter set to update the sizeState variable to double its current value when the button is clicked.
The Box composable is created with a modifier that sets its size to the value of the size variable. As the sizeState variable is updated with the button click, the size variable will change accordingly, triggering the animation of the box’s size.
Following is the output.
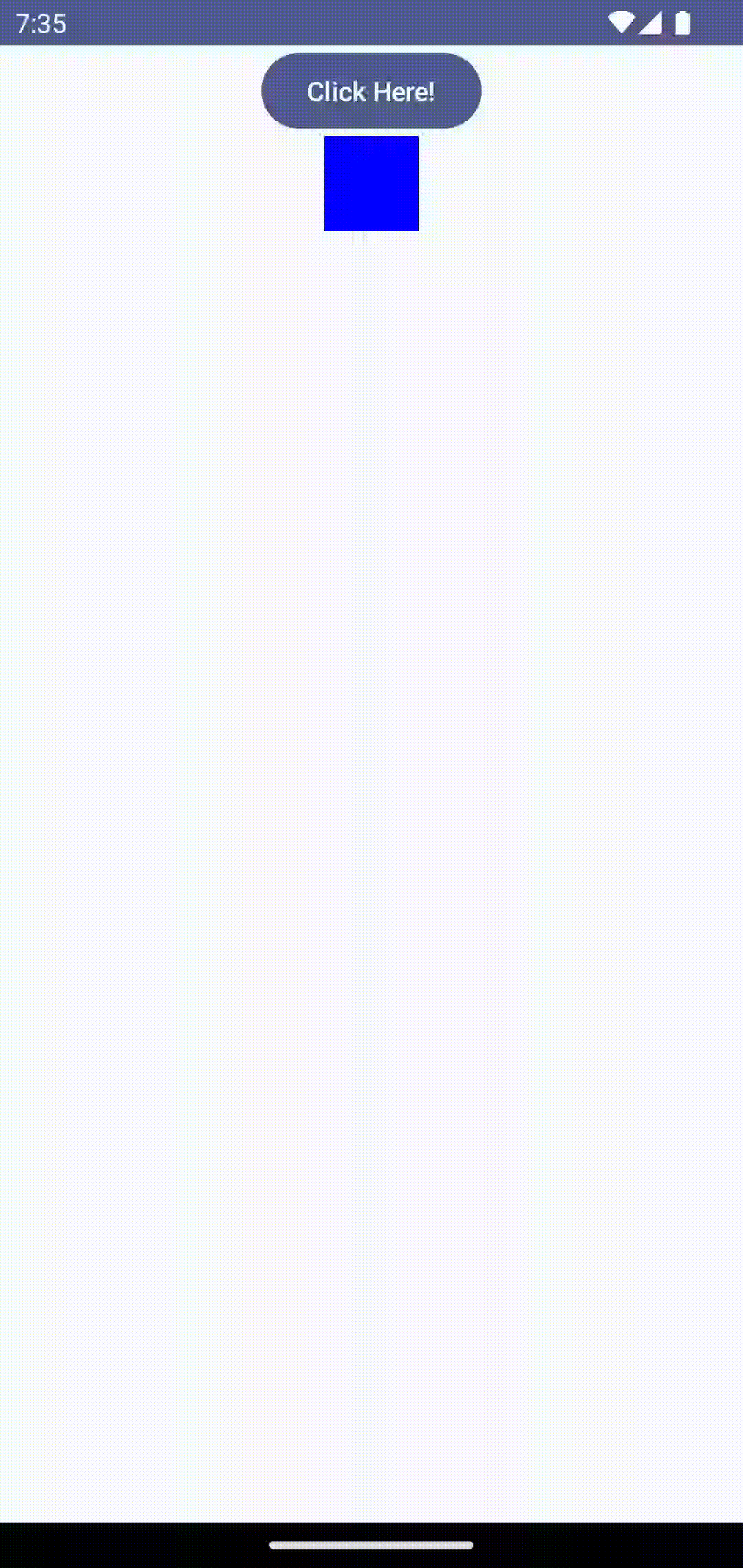
Following is the complete code for reference.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.animation.core.LinearEasing
import androidx.compose.animation.core.animateDpAsState
import androidx.compose.animation.core.tween
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.*
import androidx.compose.material3.Button
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.getValue
import androidx.compose.runtime.setValue
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
AnimationExample()
}
}
}
}
}
@Composable
fun AnimationExample() {
var sizeState by remember { mutableStateOf(50.dp) }
val size by animateDpAsState(targetValue = sizeState, tween(durationMillis = 1000,
easing = LinearEasing))
Column(Modifier.fillMaxSize(),
horizontalAlignment = Alignment.CenterHorizontally)
{
Button(onClick = { sizeState = sizeState*2 }) {
Text(text = "Click Here!")
}
Box(modifier = Modifier
.size(size)
.background(color = Color.Blue))
}
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
AnimationExample()
}
}
That’s how you add scale animation in Jetpack Compose.