How to Manage Snackbar Dismissal in Android Jetpack Compose
One of the common use-cases in mobile application development is showing the user a temporary message, and Snackbars are perfect for this. Jetpack Compose, the modern toolkit for building native UIs, allows us to easily use Snackbars in our apps.
In this blog post, we will learn how to manage the dismissal of a Snackbar in Jetpack Compose.
A Snackbar typically comes with two types of dismissal: user-initiated or automatic. User-initiated dismissal occurs when a user swipes the Snackbar away or presses the “dismiss” action. Automatic dismissal happens after a set duration.
Jetpack Compose allows for easy creation and management of snackbars using the SnackbarHostState class.
@Composable
fun SnackbarExample() {
val snackbarHostState = remember { SnackbarHostState() }
val scope = rememberCoroutineScope()
Scaffold(
snackbarHost = { SnackbarHost(snackbarHostState) },
content = { innerPadding ->
Button(onClick = {
scope.launch {
val result = snackbarHostState.showSnackbar(
message = "Snackbar Example",
actionLabel = "Action",
withDismissAction = true,
duration = SnackbarDuration.Indefinite
)
when (result) {
SnackbarResult.ActionPerformed -> {
//Do Something
}
SnackbarResult.Dismissed -> {
//Do Something
}
}
}},
modifier = Modifier
.padding(innerPadding)
.fillMaxSize()
.wrapContentSize()) {
Text(text = "Show Snackbar")
}
}
)
}
The showSnackbar function seen in the previous snippet is called with various parameters, including message, actionLabel, duration, and withDismissAction. The actionLabel parameter becomes the label for our snackbar’s dismissal action, while withDismissAction allows the snackbar to be dismissed with a swipe and with the close icon.
The dismissal of the snackbar returns a SnackbarResult, which can be either ActionPerformed or Dismissed. We can use a when statement to manage actions based on the dismissal result.
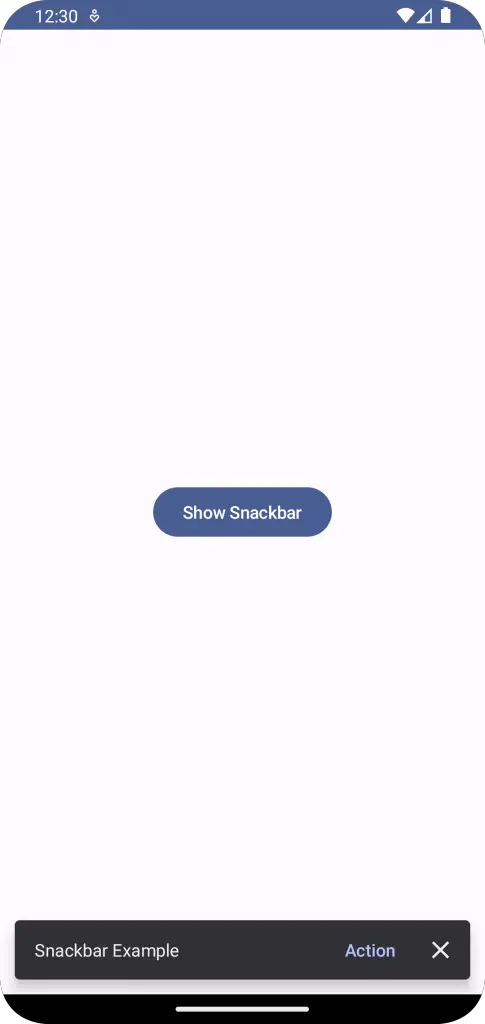
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.padding
import androidx.compose.foundation.layout.wrapContentSize
import androidx.compose.material3.Button
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Scaffold
import androidx.compose.material3.SnackbarDuration
import androidx.compose.material3.SnackbarHost
import androidx.compose.material3.SnackbarHostState
import androidx.compose.material3.SnackbarResult
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.runtime.remember
import androidx.compose.runtime.rememberCoroutineScope
import androidx.compose.ui.Modifier
import androidx.compose.ui.tooling.preview.Preview
import com.example.example.ui.theme.ExampleTheme
import kotlinx.coroutines.launch
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
SnackbarExample()
}
}
}
}
}
@Composable
fun SnackbarExample() {
val snackbarHostState = remember { SnackbarHostState() }
val scope = rememberCoroutineScope()
Scaffold(
snackbarHost = { SnackbarHost(snackbarHostState) },
content = { innerPadding ->
Button(onClick = {
scope.launch {
val result = snackbarHostState.showSnackbar(
message = "Snackbar Example",
actionLabel = "Action",
withDismissAction = true,
duration = SnackbarDuration.Indefinite
)
when (result) {
SnackbarResult.ActionPerformed -> {
//Do Something
}
SnackbarResult.Dismissed -> {
//Do Something
}
}
}},
modifier = Modifier
.padding(innerPadding)
.fillMaxSize()
.wrapContentSize()) {
Text(text = "Show Snackbar")
}
}
)
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
SnackbarExample()
}
}
Now you’re equipped with the knowledge to create and manage the dismissal of snackbars in Jetpack Compose! Remember, snackbars are versatile and can be adapted to fit the specific needs of your application.