How to change Text Letter Spacing in Android Jetpack Compose
Sometimes, you want to change text properties such as letter spacing, line height, etc. In this Jetpack Compose tutorial, let’s learn how to add custom letter spacing to Text.
You can alter the letter spacing of Text Composable using TextStyle and its parameter letterSpacing. The value should be given in scale-independent pixels(sp). See the code snippet given below.
@Composable
fun TextExample() {
Text(text = "Letter Spacing Example",
fontSize = 24.sp,
style = TextStyle(letterSpacing = 8.sp)
)
}
8 sp letter spacing is given and you can see the change in the screenshot given below.
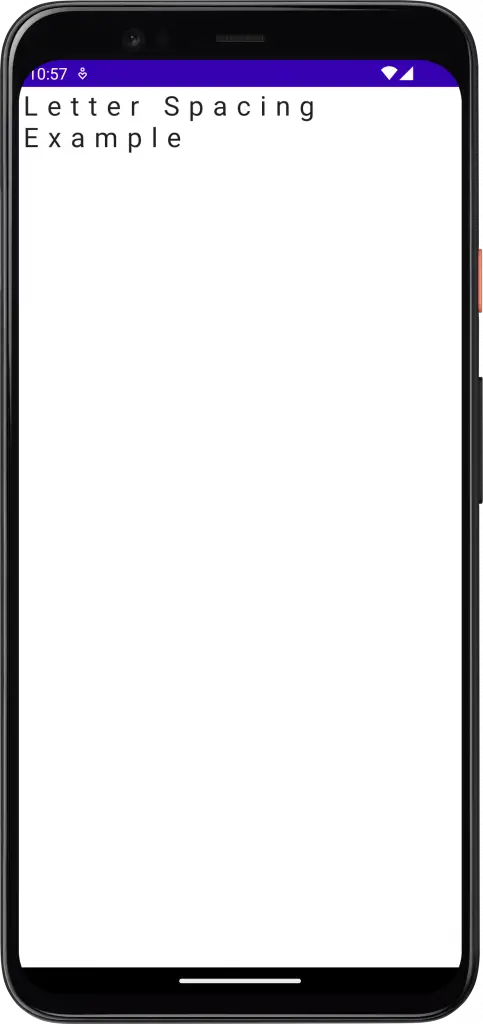
Complete Code
Following is the complete code.
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.text.TextStyle
import androidx.compose.ui.unit.sp
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
TextExample()
}
}
}
}
}
}
@Composable
fun TextExample() {
Text(text = "Letter Spacing Example",
fontSize = 24.sp,
style = TextStyle(letterSpacing = 8.sp)
)
}
That’s how you change letter spacing in Jetpack compose. Click on this tutorial to learn how to make Text italic in Jetpack Compose.
2 Comments