How to change Text Line Height in Android Jetpack Compose
Sometimes mobile app developers want to alter the line height to make the text stand out. Let’s learn how to change the default line height in Jetpack Compose.
You can change the Text line height using TextStyle and its lineHeight parameter. See the code snippet given below.
@Composable
fun TextExample() {
Text(text = "This is custom line height example. Try changing the value of lineHeight and see the difference!",
fontSize = 24.sp,
style = TextStyle(lineHeight = 40.sp)
)
}
The default line height will be changed as seen in the output.
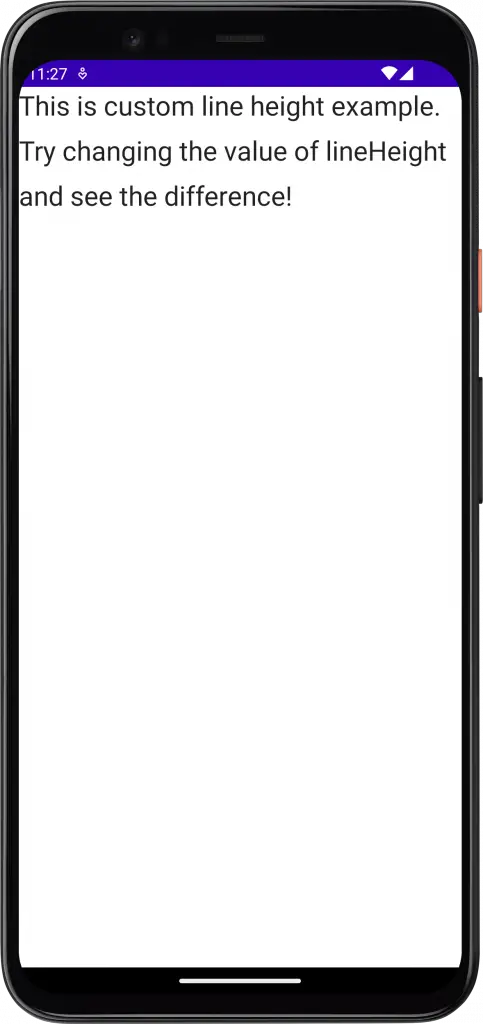
Following is the complete code for reference.
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.text.TextStyle
import androidx.compose.ui.unit.sp
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
TextExample()
}
}
}
}
}
}
@Composable
fun TextExample() {
Text(text = "This is custom line height example. Try changing the value of lineHeight and see the difference!",
fontSize = 24.sp,
style = TextStyle(lineHeight = 40.sp)
)
}
That’s how you change the Text line height in Jetpack Compose. If you want to change the letter spacing you may see this Jetpack Compose letter spacing tutorial.
One Comment