How to Add Drag Functionality using Draggable Modifier in Android Jetpack Compose
Android’s Jetpack Compose library offers a powerful and flexible system for creating UI elements. One such feature is the draggable modifier, which allows you to add drag functionality to your composable functions.
This can be especially handy for creating interactive UI elements like sliders, movable cards, and more. In this blog post, we will take a detailed look at how to use the draggable modifier in Jetpack Compose.
How to Use Draggable Modifier
A simple use case of the draggable modifier is to create a slider. Here is a basic example:
@Composable
fun ModifierExample() {
var offsetX by remember { mutableStateOf(0f) }
Column {
Box(
Modifier
.offset { IntOffset(offsetX.roundToInt(), 0) }
.draggable(
orientation = Orientation.Horizontal,
state = rememberDraggableState { delta -> offsetX += delta },
onDragStopped = { velocity -> println("Drag stopped with velocity: $velocity") }
)
.size(50.dp)
.background(Color.Red),
)
}
}
In this example, we create a Box composable that can be dragged horizontally. The offsetX variable is used to track the horizontal position of the Box composable. We use the draggable modifier and set its orientation to Orientation.Horizontal, allowing the Box to be dragged horizontally.
When the drag is stopped, the onDragStopped function is called. In our example, we print out the velocity at which the Box was being dragged when the drag was stopped.
The rememberDraggableState function is used to create a DraggableState. The DraggableState has a single function dragDeltaConsumer, which is called every time the Box is dragged. In our example, we update offsetX with the delta value, which represents the change in position.
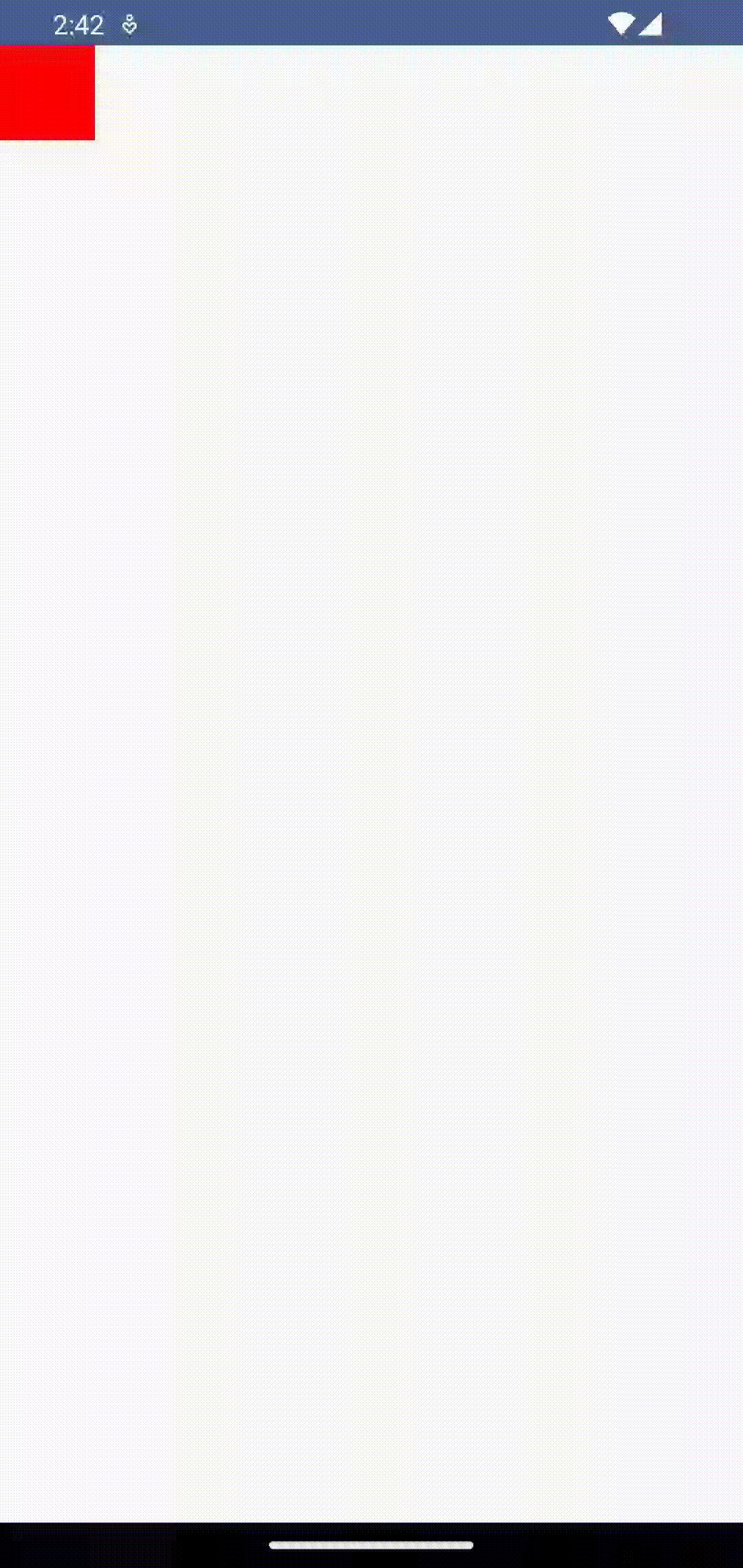
The draggable modifier in Jetpack Compose allows us to add drag functionality to our composables. Whether we need a simple slider or a complex drag-to-rearrange list, the draggable modifier provides the tools we need.