How to Change TopAppBar Background Color in Android Jetpack Compose
Jetpack Compose, a toolkit for building user interfaces in Android, is transforming the way developers approach app design. In this tutorial, we will focus on a common UI element: the TopAppBar, and specifically, how to change its background color.
Introduction to TopAppBar
TopAppBar, a key component of the Material Design guidelines, is often used for navigation, title display, and action items. It’s located at the top of the screen and provides a consistent interface across your app.
Changing the Background Color of TopAppBar
To change the background color for TopAppBar in Jetpack Compose, you can set the containerColor attribute within the colors parameter of the TopAppBar function. This attribute accepts a color, which will be used as the background color of the TopAppBar.
@Composable
fun AppBarExample() {
Scaffold(
topBar = {
TopAppBar(
title = {
Text("My App")
},
colors = TopAppBarDefaults.smallTopAppBarColors(containerColor = Color.Yellow )
)
}
) { contentPadding ->
// Screen content
Text(text = "Jetpack Compose App Bar Example",
modifier = Modifier.padding(contentPadding))
}
}
You will get the following output.
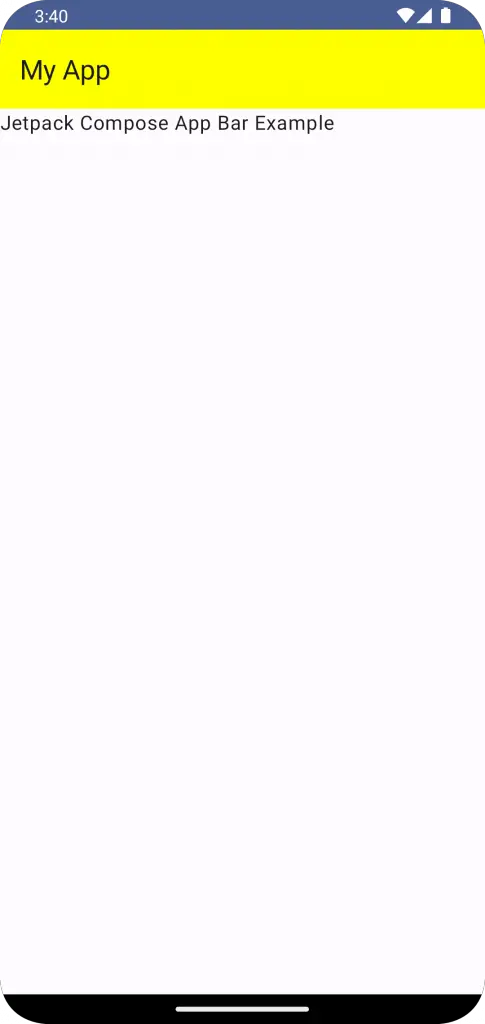
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.padding
import androidx.compose.material3.ExperimentalMaterial3Api
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Scaffold
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.material3.TopAppBar
import androidx.compose.material3.TopAppBarDefaults
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
AppBarExample()
}
}
}
}
}
@OptIn(ExperimentalMaterial3Api::class)
@Composable
fun AppBarExample() {
Scaffold(
topBar = {
TopAppBar(
title = {
Text("My App")
},
colors = TopAppBarDefaults.smallTopAppBarColors(containerColor = Color.Yellow )
)
}
) { contentPadding ->
// Screen content
Text(text = "Jetpack Compose App Bar Example",
modifier = Modifier.padding(contentPadding))
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
AppBarExample()
}
}
In this tutorial, we’ve seen how to change the background color of the TopAppBar in Jetpack Compose. This simple yet powerful feature allows you to create a consistent and appealing visual design across your Android app.
One Comment