How to change Text Color Opacity in Android Jetpack Compose
Changing the opacity of a UI component can be useful when you want to make it partially transparent, overlaying it on other UI elements. Here, in this Android development tutorial, let’s learn how to change the text opacity in Jetpack Compose.
The Text composable is used to display text in Jetpack Compose. You can change the text opacity in multiple ways.
Changing Text Opacity with Modifier
Using the modifier you can change the alpha of the text color. Thus, you can change the opacity.
See the code snippet given below.
@Composable
fun TextOpacity() {
Text(text = "Text Opacity Example",
fontSize = 30.sp,
color = Color.Red,
modifier = Modifier.alpha(0.5f))
}
The value of Modifier.alpha should be between 0.0f and 1.0f. The value in the code is 0.5f and thus the opacity is 50%. In a similar way, you can also change the opacity of composables like Image.
Following is the Text with 50% alpha.
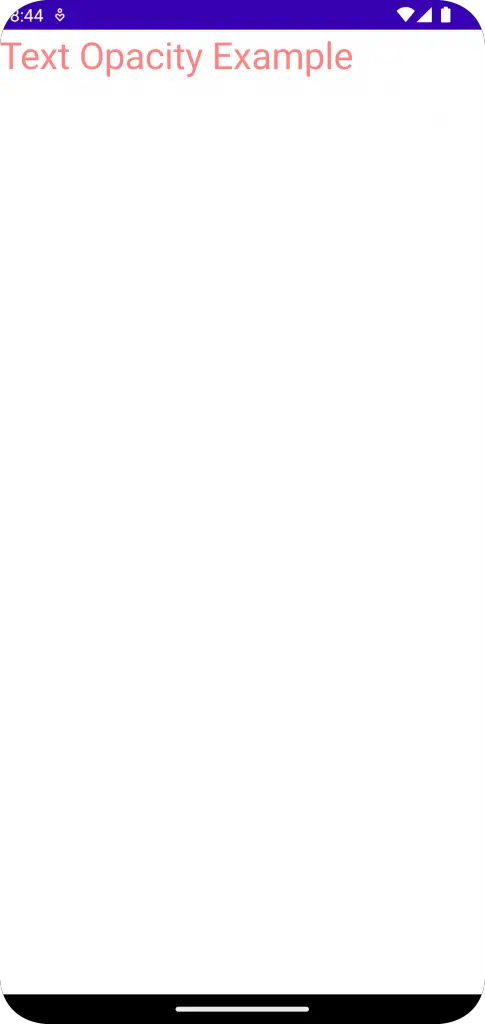
Changing Text Opacity with TextStyle
We can set the alpha value of the color in the TextStyle to change the opacity of the Text. The alpha value ranges from 0 to 1, where 0 is fully transparent and 1 is fully opaque. Let’s see how we can do this in code.
@Composable
fun TextOpacity() {
Text(text = "Text Opacity Example",
fontSize = 30.sp,
style = TextStyle(
color = Color.Red.copy(alpha = 0.5f)
))
}
Following is the complete code for reference.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.*
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.*
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.text.TextStyle
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.sp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
TextOpacity()
}
}
}
}
}
@Composable
fun TextOpacity() {
Text(text = "Text Opacity Example",
fontSize = 30.sp,
style = TextStyle(
color = Color.Red.copy(alpha = 0.5f)
))
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
TextOpacity()
}
}
We have created a composable function TextOpacity that displays the Text with an opacity of 0.5f. We have used the copy method of the Color class to create a new color instance with the same RGB values as the Red color and set the alpha value to 0.5f.
That’s how you change the text opacity in Jetpack Compose.
One Comment