How to Create Transparent Button in Android Jetpack Compose
Buttons are an essential part of any mobile application. They guide users through actions, leading them from one point to another. However, the traditional button styles may not always fit the aesthetic of your app. In such cases, a transparent button can add a sleek touch.
In this post, we’ll show you how to create a transparent button using Jetpack Compose.
Transparent Button Code Example
Here is an example of how to create a transparent button in Jetpack Compose:
@Composable
fun TransparentButtonExample() {
Button(
onClick = { println("Button Clicked!") },
modifier = Modifier.padding(10.dp),
colors = ButtonDefaults.buttonColors(containerColor = Color.Transparent, contentColor = Color.Black),
border = BorderStroke(width = 1.dp, color = Color.Black)
) {
Text(text = "Transparent Button")
}
}
Code Breakdown
Now let’s take a closer look at the code to understand each part.
The @Composable
Function
- We define a
@Composable
function calledTransparentButtonExample()
that encapsulates our button.
Button Composable
- The
Button
composable is responsible for creating the button. onClick = { println("Button Clicked!") }
specifies the action that occurs when the button is clicked.
Modifier and Padding
modifier = Modifier.padding(10.dp)
adds padding around the button.
Button Colors
- The
colors
parameter defines the visual aspects of the button. containerColor = Color.Transparent
makes the button background transparent.contentColor = Color.Black
sets the text and icon color to black.
Border Configuration
border = BorderStroke(width = 1.dp, color = Color.Black)
adds a border to the button with a width of 1.dp and a color of black.
Text Composable
- Inside the
Button
, we include aText
composable that displays the label “Transparent Button”.
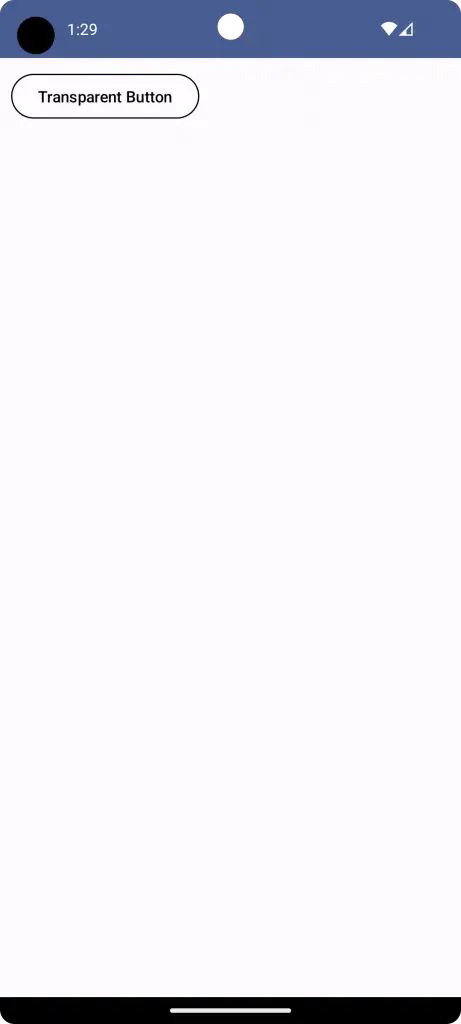
Complete Code
To use this transparent button in your app, add the TransparentButtonExample()
function to your main activity as shown below:
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.BorderStroke
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.padding
import androidx.compose.material3.Button
import androidx.compose.material3.ButtonDefaults
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.unit.dp
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
TransparentButtonExample()
}
}
}
}
}
}
@Composable
fun TransparentButtonExample() {
Button(onClick = { println("Button Clicked!") },
modifier = Modifier.padding(10.dp),
colors = ButtonDefaults.buttonColors(containerColor = Color.Transparent, contentColor = Color.Black),
border = BorderStroke(width = 1.dp, color = Color.Black)
) {
Text(text = "Transparent Button")
}
}
Creating a transparent button in Jetpack Compose is a straightforward task. With just a few lines of code, you can add a stylish transparent button to your Android app that perfectly fits your UI design. The code is clean, modular, and easy to understand, making it a breeze to integrate into any project.