How to Use Clip Modifier in Android Jetpack Compose
Jetpack Compose, the modern toolkit for building native Android UI, provides developers with powerful tools and APIs to create custom and interactive UI elements. One such tool is the clip() modifier.
Clipping allows us to control the shape and layout of a component, giving us the freedom to step beyond traditional rectangular views. In this blog post, we’ll look into the usage of clip() and explore its potential with multiple examples.
What is Clipping
In graphic design, ‘clipping’ refers to hiding certain parts of an image or a view that go beyond the defined boundaries. In the context of Jetpack Compose, the clip() modifier achieves this by changing the shape of a Composable.
You can clip your composable to predefined shapes such as CircleShape, RectangleShape, RoundedCornerShape, or you can define your own custom shapes. Let’s explore this in more detail with some examples.
Circle Clipping
Jetpack Compose comes with a predefined shape for circle. Here is an example of how to clip a box into a circle:
@Composable
fun ModifierExample() {
Column {
Box(
modifier = Modifier
.size(100.dp)
.clip(CircleShape)
.background(Color.Red)
)
}
}
In this example, the box is circular due to the CircleShape parameter passed into the clip() function.
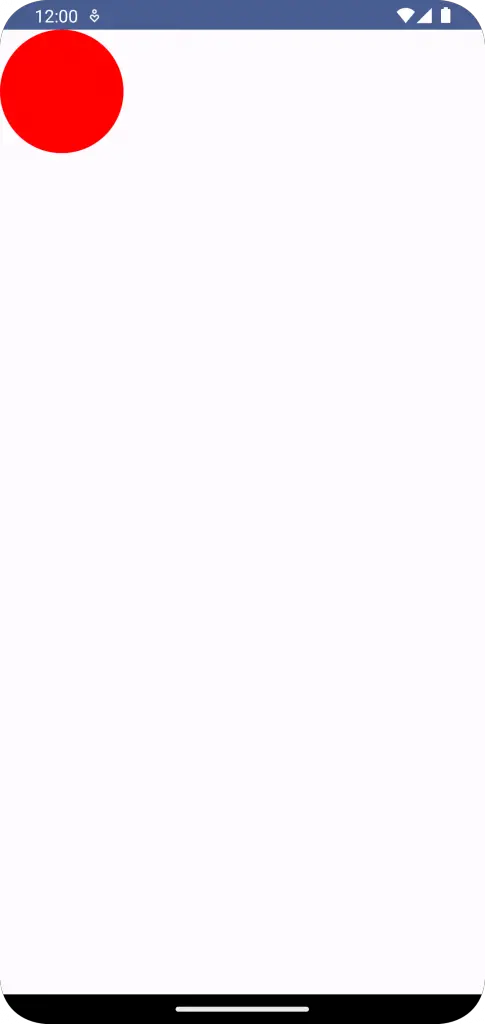
Rounded Rectangle Clipping
If we want the corners of our rectangle to be rounded, we can use RoundedCornerShape(). Here’s how:
@Composable
fun ModifierExample() {
Column {
Box(
modifier = Modifier
.size(100.dp)
.clip(RoundedCornerShape(10.dp))
.background(Color.Red)
)
}
}
In this example, the rectangle has rounded corners with a corner radius of 10.dp.
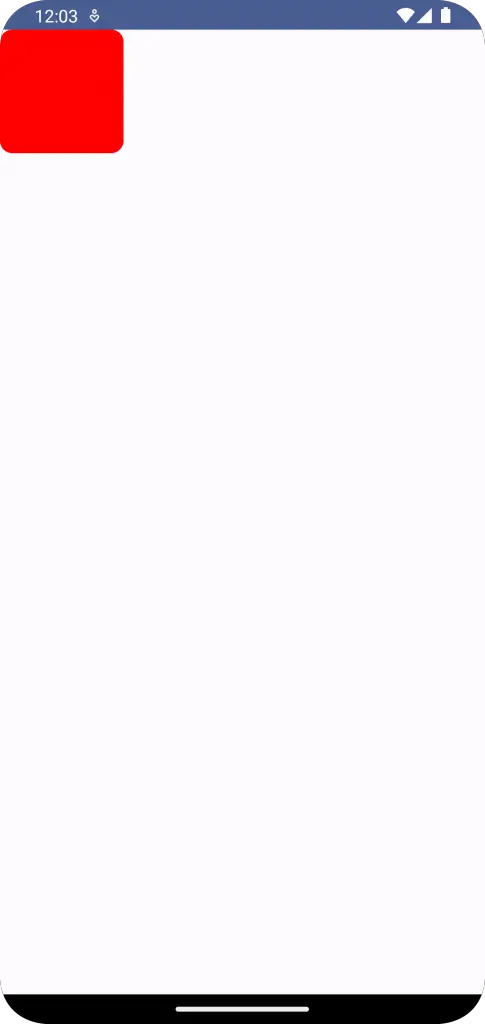
Clipping Images
Images can also be clipped to provide a more aesthetic or unique look. Let’s say you have an image that you want to appear as a circle. Here’s how you would achieve this:
@Composable
fun ModifierExample() {
Column {
Image(
painter = painterResource(id = R.drawable.nature),
contentDescription = "Example Image",
modifier = Modifier
.size(200.dp)
.clip(CircleShape),
contentScale = ContentScale.Crop
)
}
}
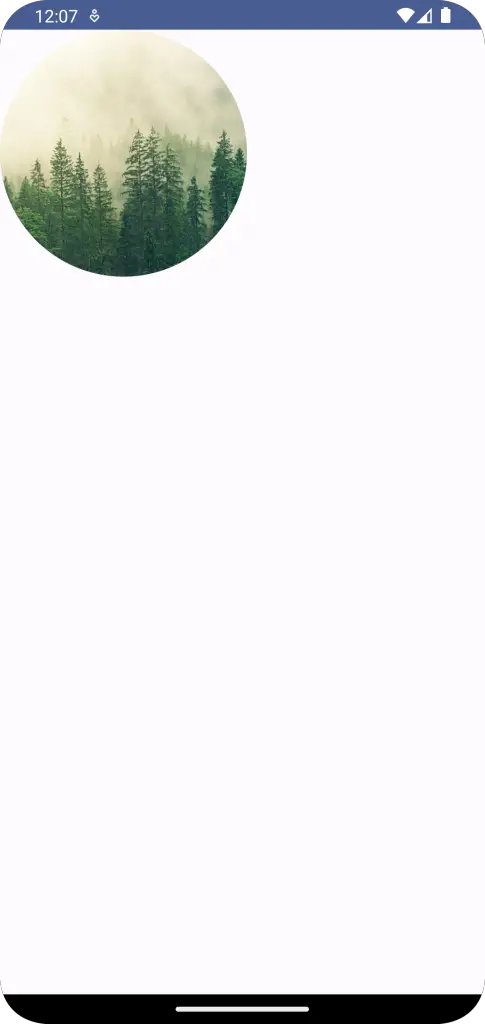
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.Image
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.size
import androidx.compose.foundation.shape.CircleShape
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.draw.clip
import androidx.compose.ui.layout.ContentScale
import androidx.compose.ui.res.painterResource
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
ModifierExample()
}
}
}
}
}
@Composable
fun ModifierExample() {
Column {
Image(
painter = painterResource(id = R.drawable.nature),
contentDescription = "Example Image",
modifier = Modifier
.size(200.dp)
.clip(CircleShape),
contentScale = ContentScale.Crop
)
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
ModifierExample()
}
}
The clip() modifier is an excellent tool in Jetpack Compose’s arsenal, allowing developers to easily modify the shape of any composable. Whether you’re using predefined shapes or creating your own, clip() offers endless possibilities for customizing your UI design.