How to Use Background Modifier in Android Jetpack Compose
Jetpack Compose has taken the Android development world by storm with its simplicity, conciseness, and powerful functionality. One such feature of Jetpack Compose is the background modifier, which allows developers to easily manipulate the background aspects of a composable.
What is a Modifier
Before diving into the background modifier, let’s understand what a modifier is in the Jetpack Compose context. A modifier in Jetpack Compose is a series of declarative UI transformations or side effects that you can apply to a composable to alter its behavior or appearance.
What is a Background Modifier
Among the many available modifiers, background is one that you’ll find yourself using quite frequently. As the name suggests, the background modifier lets you apply background colors or shapes to your composables. Let’s explore this in more depth through several practical examples.
How to Apply Background Color
The most straightforward use of the background modifier is to apply a solid color to a composable. Here’s an example:
@Composable
fun ModifierExample() {
Column {
Box(modifier = Modifier.background(Color.Red).height(100.dp).fillMaxWidth()) {
Text("Hello, Jetpack Compose!", color = Color.White)
}
}
}
This block of code creates a Box composable with a Text composable nested within it. The background modifier applies a solid magenta color to the Box composable, which becomes the background color for the text.
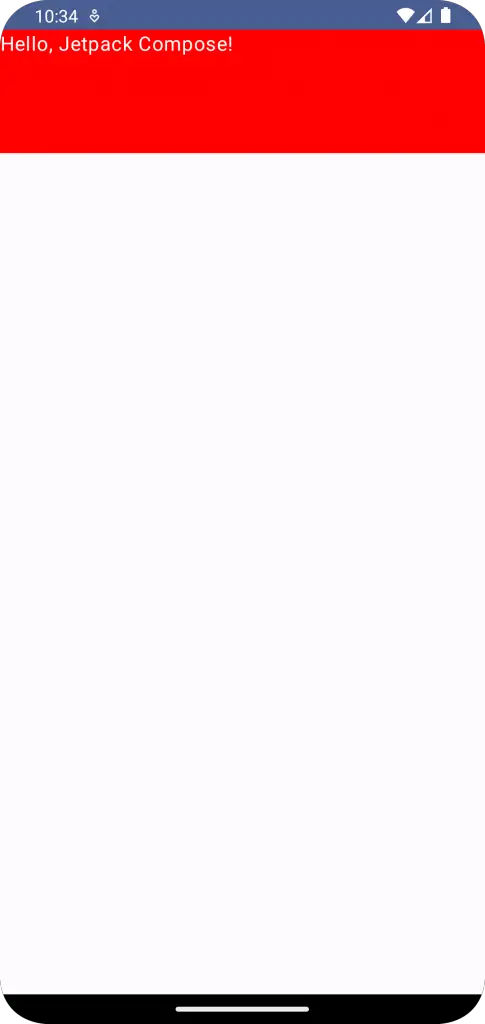
How to Apply Gradient Background
While solid colors are great, what if you want a more dynamic background, like a gradient? With the background modifier, you can achieve this:
@Composable
fun ModifierExample() {
Column {
val gradientBrush = Brush.horizontalGradient(
colors = listOf(Color.Yellow, Color.Blue),
startX = 0f,
endX = Float.POSITIVE_INFINITY
)
Box(modifier = Modifier.background(brush = gradientBrush).height(100.dp).fillMaxWidth()) {
Text(text = "Hello, Gradient!", color = Color.White)
}
}
}
In this example, Brush.horizontalGradient creates a gradient from yellow to blue, which is then used as the brush argument for the background modifier, providing a more visually dynamic background for your composable.
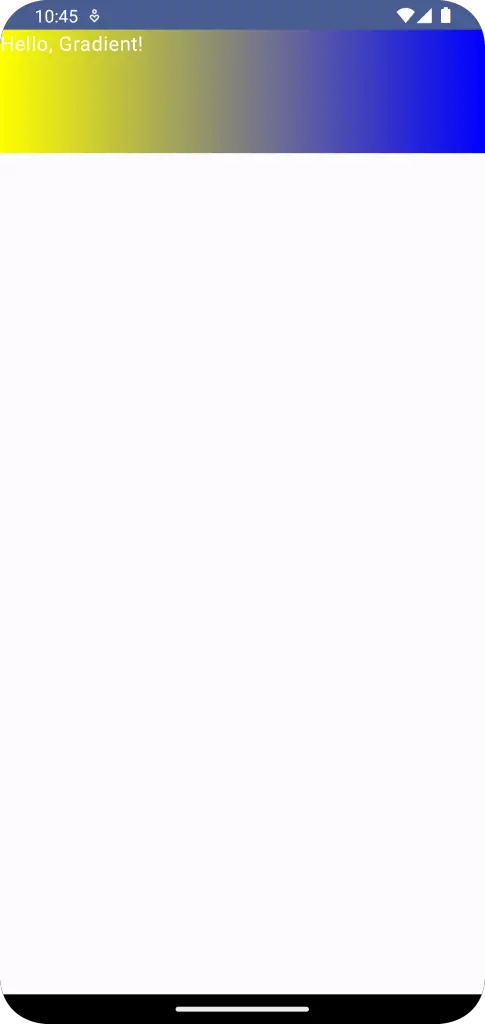
How to Apply Background Shape
Jetpack Compose also supports shapes as backgrounds. Here’s an example of a Box composable with a rounded rectangle background:
@Composable
fun ModifierExample() {
Column {
Box(modifier = Modifier.padding(10.dp).background(Color.Green, shape = RoundedCornerShape(10.dp)).height(100.dp)
.fillMaxWidth()) {
Text(text = "Hello, Shaped Background!")
}
}
}
Here, we pass a RoundedCornerShape object to the shape parameter of the background modifier. This gives our Box composable a rounded rectangle background.
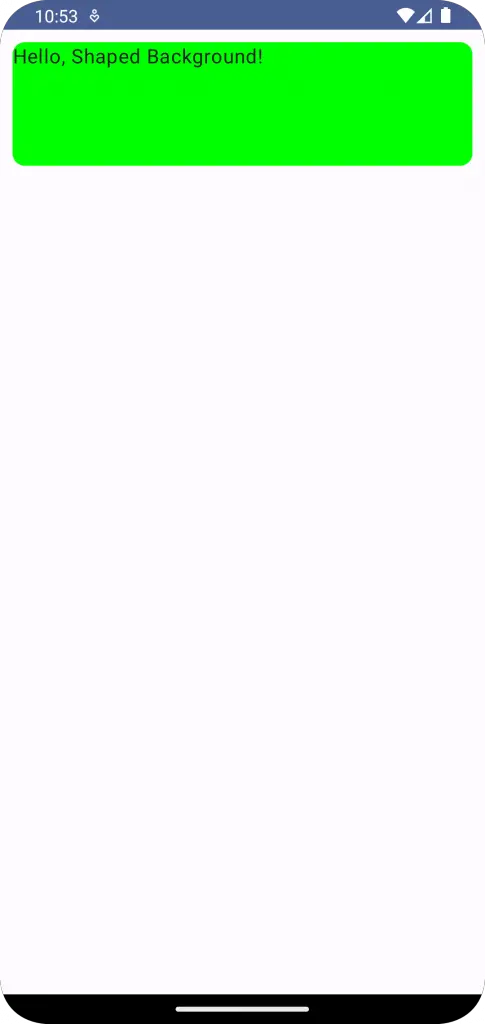
How to Use Image as Background
First, you need to include your image resource in the res/drawable directory. For the purpose of this example, let’s assume you have an image resource named nature.
@Composable
fun ModifierExample() {
Column {
Box(modifier = Modifier.fillMaxSize().paint(
// Replace with your image id
painterResource(id = R.drawable.nature),
contentScale = ContentScale.Crop),
) {
Text(text = "Hello, Image Background!", color = Color.White)
}
}
}
Following is the output.
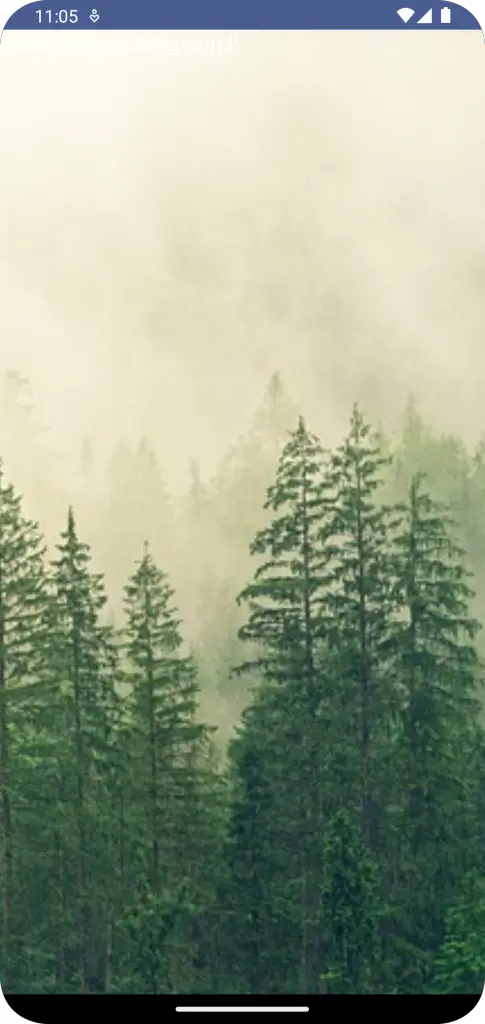
These examples showcase the versatility and simplicity of the background modifier in Jetpack Compose. With this tool in your belt, you can create visually engaging backgrounds for any composable.