How to Change Card Background Color in Android Jetpack Compose
Cards are pivotal components of today’s mobile apps, offering a clean, organized user interface for better content presentation. Jetpack Compose provides a robust Card composable to serve this purpose.
In this blog post, we will delve into how to set a background color for cards in Jetpack Compose, keeping in line with the latest updates in the library.
The Card Composable
The Card composable in Jetpack Compose is used to exhibit information on a Material Design card. It allows you to place content within, which could range from text to images or both.
Here’s a basic example:
Card {
Text("Hello from Jetpack Compose!")
}
This snippet will generate a card containing the text “Hello from Jetpack Compose!”.
How to Set Card Background Color
In Jetpack Compose, changing the background color of a card has been made straightforward. You now use the CardDefaults.cardColors() function with the colors parameter of the Card composable.
Here’s the updated way to set the background color of a card:
Card(modifier = Modifier.fillMaxWidth().height(150.dp).padding(20.dp),
colors = CardDefaults.cardColors(
containerColor = Color.Yellow,
)) {
Text("Hello from Jetpack Compose!")
}
This code snippet creates a card with a yellow background color, a width that fills the maximum available width, a height of 150 dp, and a padding of 20 dp around the card.
Following is the output.
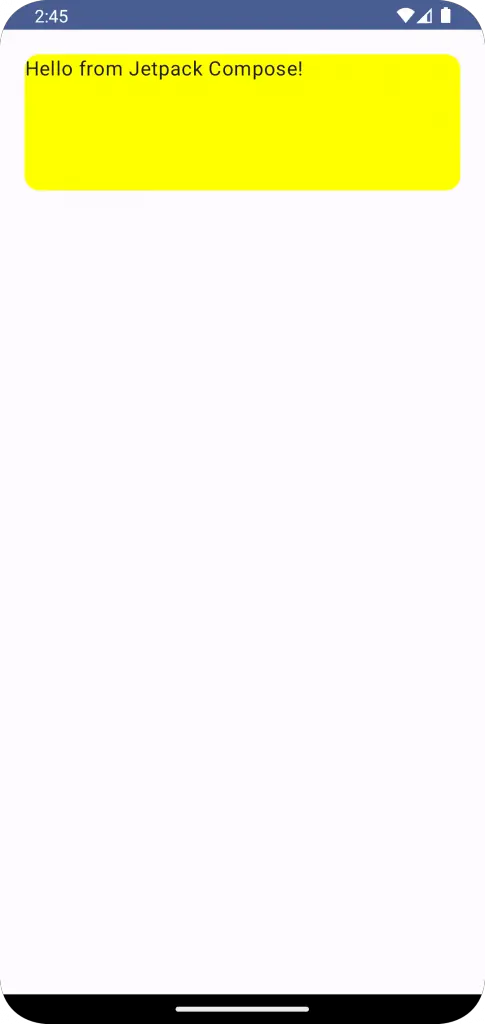
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.height
import androidx.compose.foundation.layout.padding
import androidx.compose.material3.Card
import androidx.compose.material3.CardDefaults
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
IconExample()
}
}
}
}
}
@Composable
fun IconExample() {
Column{
Card(modifier = Modifier.fillMaxWidth().height(150.dp).padding(20.dp),
colors = CardDefaults.cardColors(
containerColor = Color.Yellow,
)) {
Text("Hello from Jetpack Compose!")
}
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
IconExample()
}
}
With Jetpack Compose, you can build and customize cards with various background colors that fit your app’s theme and branding. The Card composable offers a simple, flexible way to present information neatly.
One Comment