How to Make TextField Single Line in Android Jetpack Compose
Creating user input fields is essential in mobile app development. While the default behavior of a TextField
in Jetpack Compose is to be multiline, sometimes you only need a single line for short inputs like usernames or passwords.
This blog post will guide you on how to implement a single-line TextField
in your Android Jetpack Compose app.
TextField in Jetpack Compose
TextField
is a composable function that lets you create input fields in your app. By default, it is configured to accept multiple lines of text. However, you can easily modify it to be single-line.
Set Up a Single Line TextField
The key parameter to focus on is singleLine
. By setting this parameter to true
, you make the TextField
single-line. Take a look at the following code snippet for reference:
@Composable
fun TextFieldExample() {
var textInput by remember { mutableStateOf("") }
TextField(
value = textInput,
onValueChange = { textInput = it },
placeholder = { Text("Username") },
singleLine = true,
modifier = Modifier.fillMaxWidth()
.padding(10.dp)
)
}
Notice Keyboard Changes
One small but noteworthy feature is that when you set the TextField
to be single-line, the keyboard’s enter button changes to a tick mark. This subtle change enhances user experience by providing a visual cue that the input should be short and concise.
Complete Code Example
For those who like to see the complete code, here it is:
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.padding
import androidx.compose.material3.ExperimentalMaterial3Api
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.material3.TextField
import androidx.compose.runtime.Composable
import androidx.compose.runtime.getValue
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.setValue
import androidx.compose.ui.Modifier
import androidx.compose.ui.unit.dp
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
TextFieldExample()
}
}
}
}
}
}
@OptIn(ExperimentalMaterial3Api::class)
@Composable
fun TextFieldExample() {
var textInput by remember { mutableStateOf("")}
TextField(value = textInput,
onValueChange = {textInput = it},
placeholder = { Text("Username") },
singleLine = true,
modifier = Modifier.fillMaxWidth()
.padding(10.dp))
}
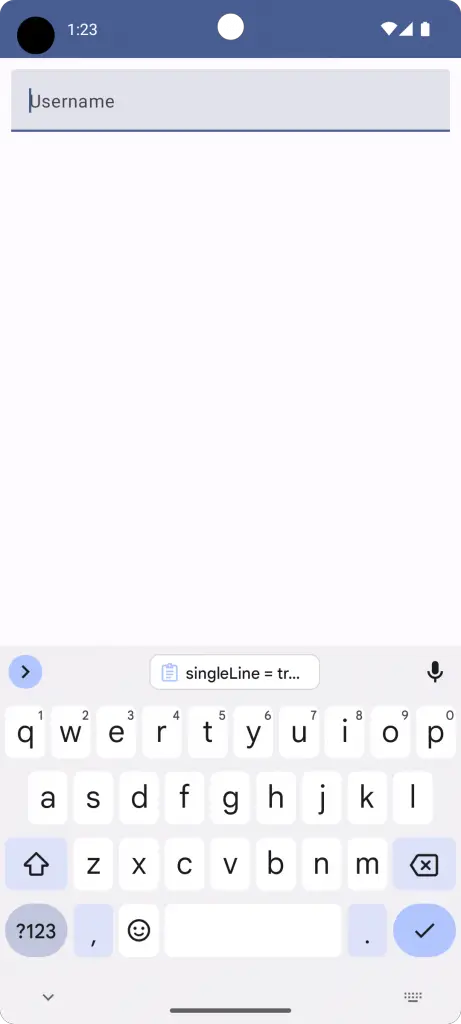
That wraps up our tutorial on how to create a single-line TextField
in Jetpack Compose. You can now successfully implement this feature in your apps for better user experience.
If you want to restrict the number of TextField characters then see this tutorial.