How to Use Modifier Weight in Android Jetpack Compose
Jetpack Compose has given a new perspective to UI development in Android with its declarative programming model. Composable functions are the building blocks of this framework, and modifiers are the essential tools used to design the layout, appearance, and behavior of these Composable functions.
In this blog post, we will focus on the Modifier.weight function and its usage. The Modifier.weight function is a part of the Jetpack Compose foundation layout. It helps distribute the available space among the children of a Row or Column Composable.
What is Modifier.weight?
Modifier.weight is a function that allocates available space along the main axis (horizontal for Row and vertical for Column) to its child elements. The distribution is done in proportion to the weightage assigned to each child. It’s similar to the concept of “weight” in LinearLayout in traditional Android development.
The Modifier.weight function takes a single Float parameter, weight. The space each child occupies is proportional to its weight divided by the total weight of all children.
Modifier Weight Examples
Let’s start with a simple example that uses Modifier.weight in a Row.
@Composable
fun WeightInRowExample() {
Row(Modifier.fillMaxWidth()) {
Box(
Modifier
.fillMaxHeight()
.weight(1f)
.background(Color.Red)) { }
Box(
Modifier
.fillMaxHeight()
.weight(2f)
.background(Color.Green)) { }
}
}
In the above example, a Row composable has two Box children. The available horizontal space is split between them according to their weight, i.e., in a 1:2 ratio. The Box with Modifier.weight(1f) will occupy a third of the horizontal space, and the Box with Modifier.weight(2f) will fill the remaining two-thirds.
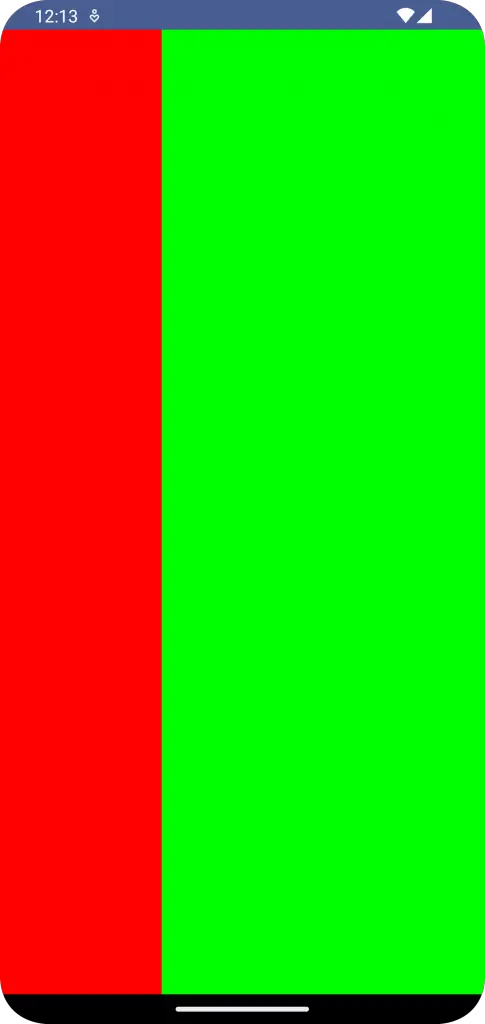
Now, let’s examine a similar example using a Column.
@Composable
fun WeightInColumnExample() {
Column(Modifier.fillMaxHeight()) {
Box(Modifier.fillMaxWidth().weight(1f).background(Color.Yellow)) { }
Box(Modifier.fillMaxWidth().weight(3f).background(Color.Cyan)) { }
}
}
In this example, we distribute the vertical space of a Column between two Box composables. The Box with Modifier.weight(1f) gets 1/4 of the total height, while the Box with Modifier.weight(3f) takes up the remaining 3/4. This allocation reflects the 1:3 ratio specified by the weight values.
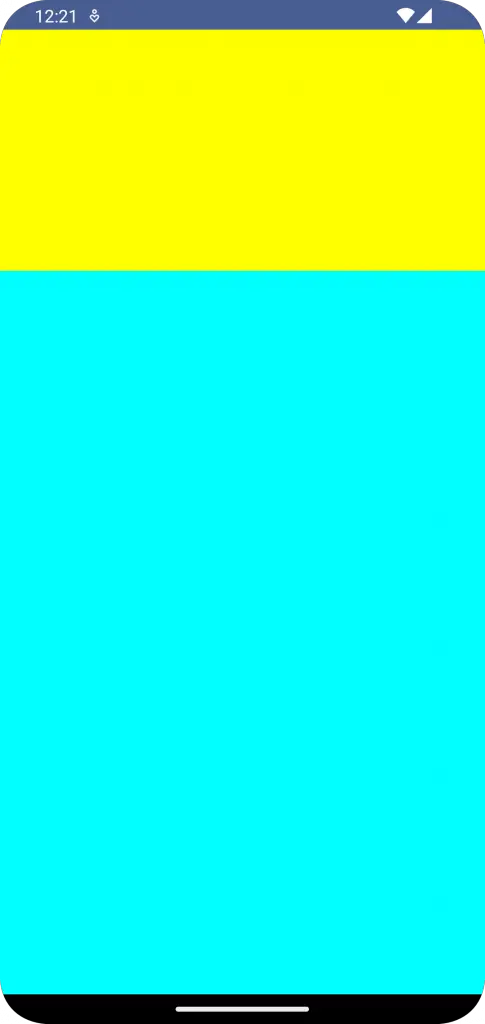
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.Box
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxHeight
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
WeightInColumnExample()
}
}
}
}
}
@Composable
fun WeightInColumnExample() {
Column(Modifier.fillMaxHeight()) {
Box(Modifier.fillMaxWidth().weight(1f).background(Color.Yellow)) { }
Box(Modifier.fillMaxWidth().weight(3f).background(Color.Cyan)) { }
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
WeightInColumnExample()
}
}
Modifier.weight is a powerful and flexible tool for creating responsive and adaptive layouts in Jetpack Compose. With its help, you can allocate available space in a Row or Column efficiently and responsively, ensuring your app’s UI remains fluid and adapts gracefully to different screen sizes and orientations.