How to Add Linear Progress Indicator in Android Jetpack Compose
When developing Android apps, providing feedback to users is crucial. One way to indicate progress or loading is by using linear progress indicators. Jetpack Compose simplifies this process with its LinearProgressIndicator
composable.
In this comprehensive guide, we’ll cover how to add both indeterminate and determinate linear progress indicators using Jetpack Compose.
Linear Progress Indicator
Linear progress indicators are horizontal bars that fill up over time. They can be both determinate, indicating a specific amount of progress, or indeterminate, showing that an operation is ongoing without specifying its duration.
Basic Example: Indeterminate Linear Progress Indicator
First, let’s look at a basic example of an indeterminate progress indicator. This type doesn’t show how much of the task has been completed—only that it’s ongoing.
@Composable
fun ProgressIndicator() {
Column {
LinearProgressIndicator(
modifier = Modifier
.fillMaxWidth().padding(10.dp),
color = Color.Red,
trackColor = Color.Gray
)
}
}
In this example, the LinearProgressIndicator
fills the entire width of its container and has padding of 10dp. We set its color to red and the track color to gray.
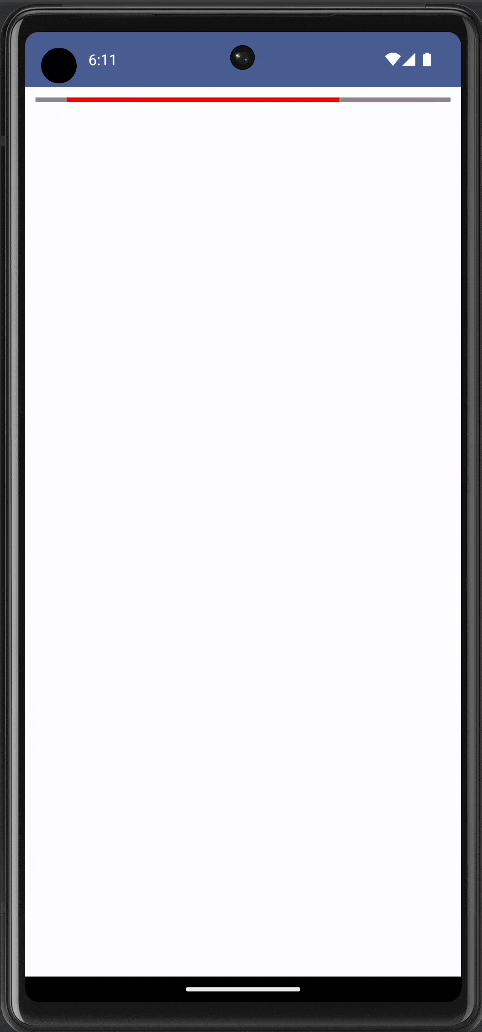
Example: Determinate Linear Progress Indicator
Determinate indicators show how much progress has been made. You need to set the progress
parameter to a float value between 0 and 1.
@Composable
fun ProgressIndicator() {
var progress by remember { mutableStateOf(0.3f) }
Column {
LinearProgressIndicator(
progress = progress,
modifier = Modifier
.fillMaxWidth().padding(10.dp),
color = Color.Green,
trackColor = Color.Gray
)
}
}
In this example, the LinearProgressIndicator
will fill up 30% of the bar. We set the progress
to 0.3f
, indicating 30% completion.
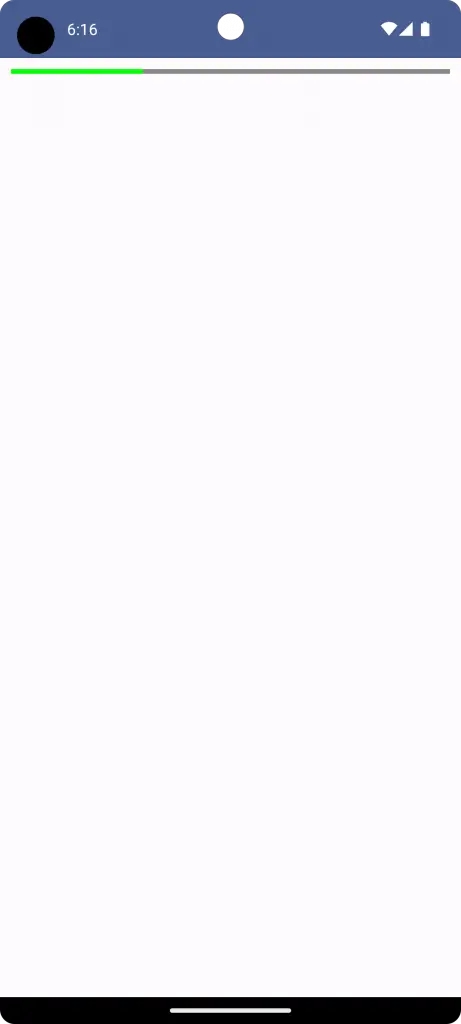
Jetpack Compose offers a highly customizable and straightforward way to implement linear progress indicators. Whether you’re looking for a basic indeterminate indicator or a determinate one, Jetpack Compose has got you covered.
Experiment with the different properties to create the perfect indicator for your application.
One Comment