How to Create Rotation Animation in Android Jetpack Compose
Animations can enhance the visual interest and interactivity of a mobile app. In this Android tutorial, let’s learn how to create a simple rotation animation in Jetpack Compose.
In this tutorial, we are going to create a rotation animation that rotates an image infinite times. See the following code.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.animation.core.*
import androidx.compose.foundation.Image
import androidx.compose.foundation.layout.*
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.runtime.getValue
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.draw.rotate
import androidx.compose.ui.res.painterResource
import androidx.compose.ui.tooling.preview.Preview
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
AnimationExample()
}
}
}
}
}
@Composable
fun AnimationExample() {
val infiniteTransition = rememberInfiniteTransition()
val angle by infiniteTransition.animateFloat(
initialValue = 0f,
targetValue = 360f,
animationSpec = infiniteRepeatable(
animation = tween(2000, easing = LinearEasing),
repeatMode = RepeatMode.Restart
)
)
Column(Modifier.fillMaxSize(),horizontalAlignment = Alignment.CenterHorizontally,
verticalArrangement = Arrangement.Center)
{
val image = painterResource(id = R.drawable.circle)
Image(painter = image, modifier = Modifier.rotate(angle), contentDescription = null)
}
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
AnimationExample()
}
}
The code defines an AnimationExample composable function that creates an animation of a rotating circle.
The animation is created using the rememberInfiniteTransition and animateFloat functions. The rememberInfiniteTransition creates an instance of InfiniteTransition that is used to keep track of the animation progress over time.
The animateFloat is used to animate a floating-point value over a specified duration and with a specific easing effect. In this case, the target value is set to 360 degrees and the animation repeats indefinitely.
The rotating circle is represented as an image and is displayed in the center of the screen using a Column layout. The rotate modifier is applied to the Image to rotate it by the animated angle value.
Following is the output.
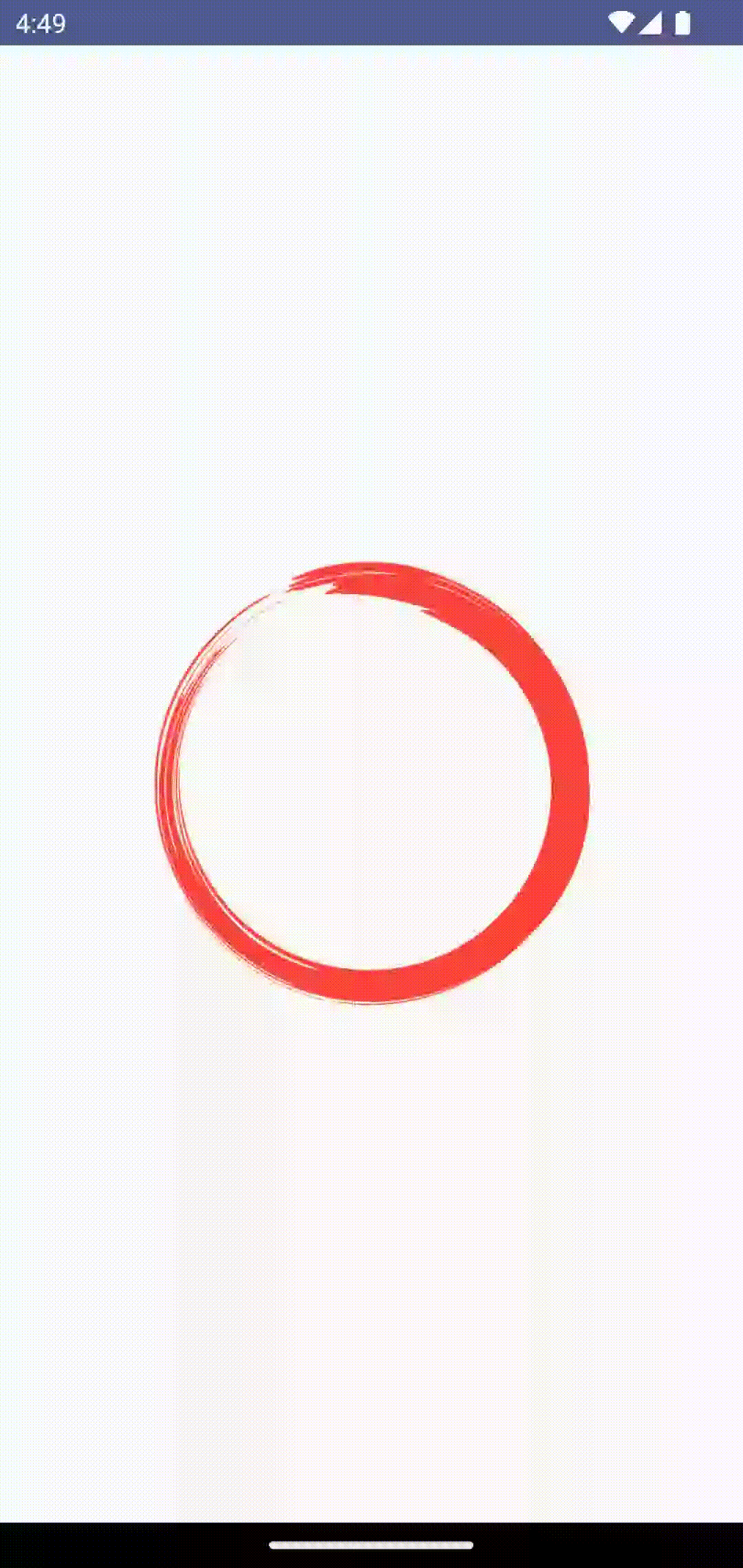
That’s how you create rotation animation in Jetpack Compose.