How to Create Infinite Animations in Android Jetpack Compose
Most of the time, the animations are finite. But in some scenarios, we may want to repeat animations infinitely. In this Android tutorial, let’s learn how to create infinite animations in Jetpack Compose.
We use the rememberInfiniteTransition API in this tutorial. It is a high level animation API in compose and it can be used to repeat animations infinite times.
In the following example, we animate the background color of the Box composable infinitely.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.animation.animateColor
import androidx.compose.animation.core.*
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.*
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.runtime.getValue
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
AnimationExample()
}
}
}
}
}
@Composable
fun AnimationExample() {
val infiniteTransition = rememberInfiniteTransition()
val bgColor by infiniteTransition.animateColor(
initialValue = Color.Red,
targetValue = Color.Green,
animationSpec = infiniteRepeatable(
animation = tween(1000, easing = LinearEasing),
repeatMode = RepeatMode.Reverse
)
)
Column(Modifier.fillMaxSize(),horizontalAlignment = Alignment.CenterHorizontally,
verticalArrangement = Arrangement.Center)
{
Box(modifier = Modifier.size(100.dp).background(color = bgColor))
}
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
AnimationExample()
}
}
The AnimationExample function is using the rememberInfiniteTransition method to create an infinite animation transition. The animateColor method is then used to animate the background color of a box between red and green.
The animation transition is defined using a tween animation, which has a duration of 1000ms and uses a LinearEasing easing curve. The animation is set to repeat in reverse using the infiniteRepeatable function and the RepeatMode.Reverse repeat mode.
Following is the output.
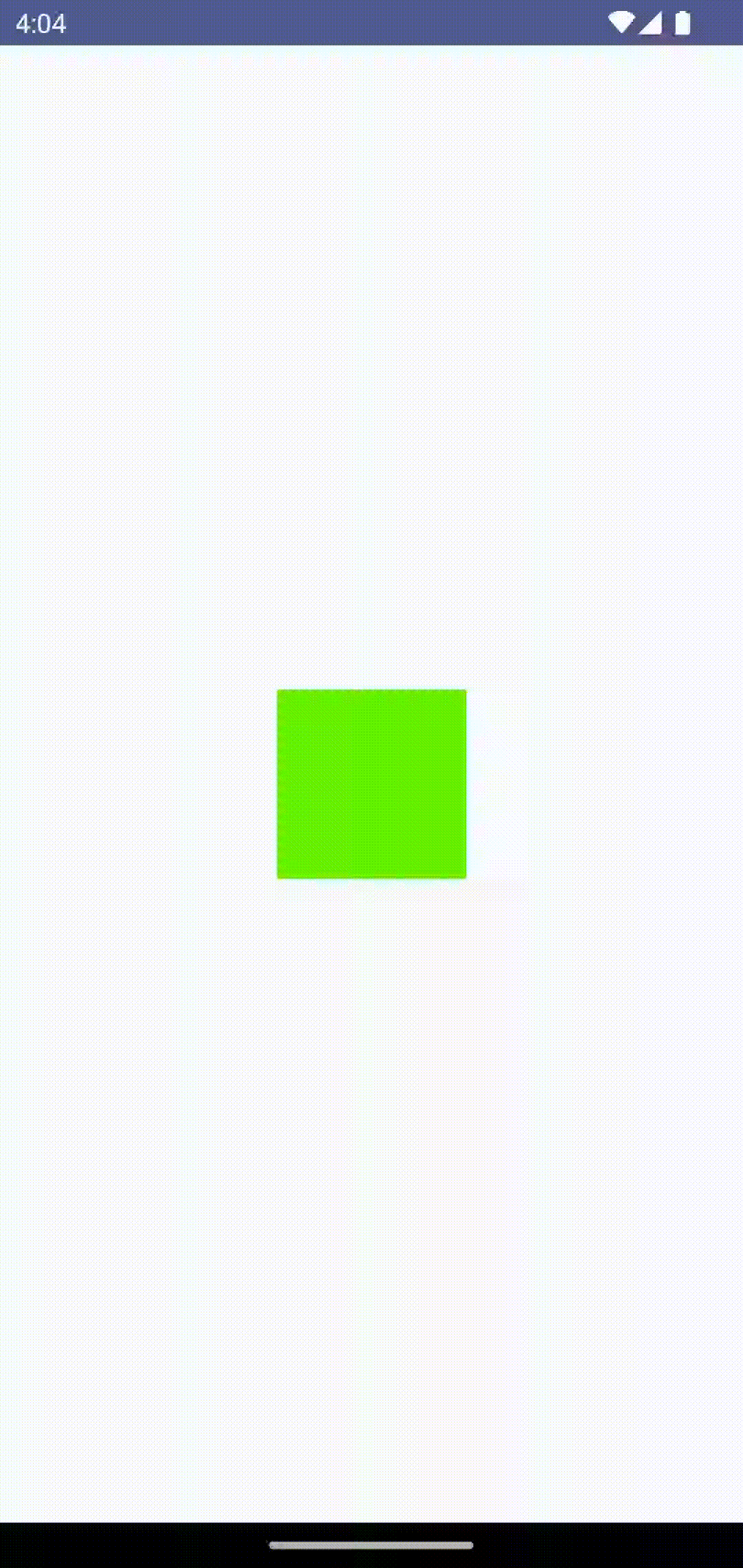
That’s how you add infinite animations in Jetpack Compose.
One Comment