How to Animate Content Size in Android Jetpack Compose
You can create dynamic and visually engaging mobile app by using the animations properly. In this Android tutorial, let’s learn how to animate content size in Jetpack Compose.
We use animateContentSize which is a high level animation API in Jetpack Compose. It helps to animate a size change and is used within the modifier.
When you use animateContentSize, have an eye on its order. Ensure that it comes before other size related modifiers for smooth animations.
Following is an example code where we use animateContentSize to animate the expansion and collapse of Text.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.animation.animateColorAsState
import androidx.compose.animation.animateContentSize
import androidx.compose.animation.core.LinearEasing
import androidx.compose.animation.core.tween
import androidx.compose.foundation.background
import androidx.compose.foundation.clickable
import androidx.compose.foundation.layout.*
import androidx.compose.material3.Button
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.getValue
import androidx.compose.runtime.setValue
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import androidx.compose.ui.unit.sp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
AnimationExample()
}
}
}
}
}
@Composable
fun AnimationExample() {
var expanded by remember { mutableStateOf(false) }
Column(Modifier.background(Color.Green).animateContentSize().fillMaxWidth().padding(20.dp))
{
Text(text = "Click to Expand",
modifier = Modifier.clickable { expanded = !expanded },
fontSize = 24.sp
)
if (expanded) {
Text(text = "On the other hand, we denounce with righteous indignation and dislike men who are so beguiled and demoralized by the charms of pleasure of the moment and trouble that are bound to ensue;")
}
}
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
AnimationExample()
}
}
The AnimationExample function creates a column with a green background color that can be expanded or contracted by clicking on a text element.
The column content size is animated using the animateContentSize function. The fillMaxWidth modifier is used to ensure that the column takes up the maximum possible width and the padding of 20dp is applied to give the content some space.
The text inside the column is clickable, and when clicked, it toggles the expanded state. If the state is expanded, then the other text element is displayed.
See the following output.
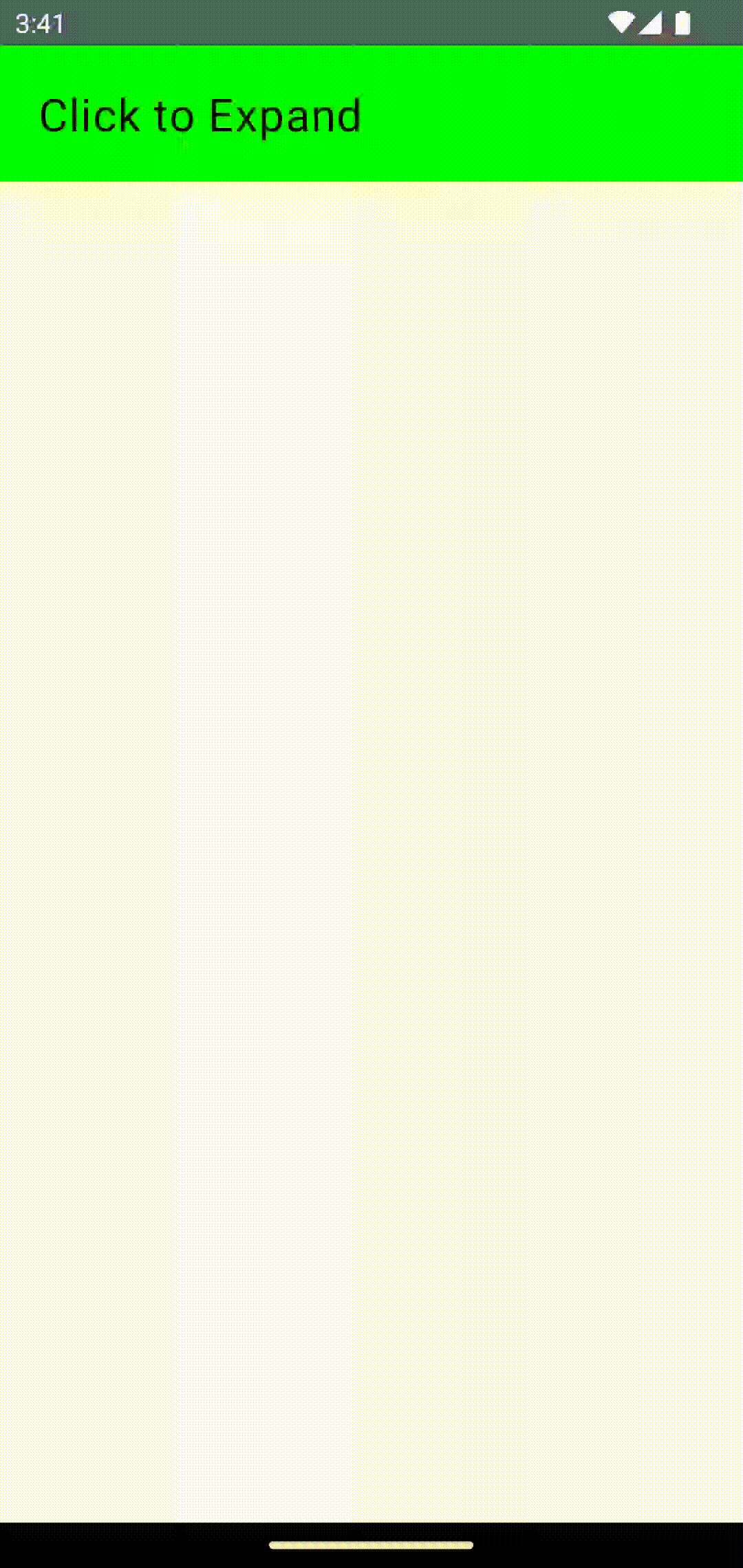
That’s how you animate content size in Jetpack Compose.
One Comment