How to Create Spring Animation in Android Jetpack Compose
Adding subtle animations can make your mobile app more engaging and vibrant. In this Android tutorial, let’s learn how to add spring animation in Jetpack Compose.
In order to create a spring effect in animations you have to use the spring function. It has parameters such as dampingRatio and stiffness to customize the spring effect.
Here, we use animateContentSize to create animations when the column composable is expanded and collapsed.
See the following code snippet.
var expanded by remember { mutableStateOf(false) }
Column(Modifier.background(Color.Green).animateContentSize(animationSpec = spring(
dampingRatio = Spring.DampingRatioMediumBouncy,
stiffness = Spring.StiffnessLow
)).fillMaxWidth().padding(20.dp))
{
Text(text = "Click to Expand",
modifier = Modifier.clickable { expanded = !expanded },
fontSize = 24.sp
)
if (expanded) {
Text(text = "On the other hand, we denounce with righteous indignation and dislike men who are so beguiled and demoralized by the charms of pleasure of the moment and trouble that are bound to ensue;")
}
}
This code creates a user interface with a “Click to Expand” text that toggles the display of additional text when clicked. The additional text expands and collapses with a spring animation.
The first line declares a mutable state variable called “expanded” and initializes it to a value of “false”, which indicates that the additional text is initially hidden.
The animateContentSize function is called with a SpringSpec animation builder to animate the size of the column to adjust to its content, and to create a spring animation with a bouncy feel.
See the output given below.
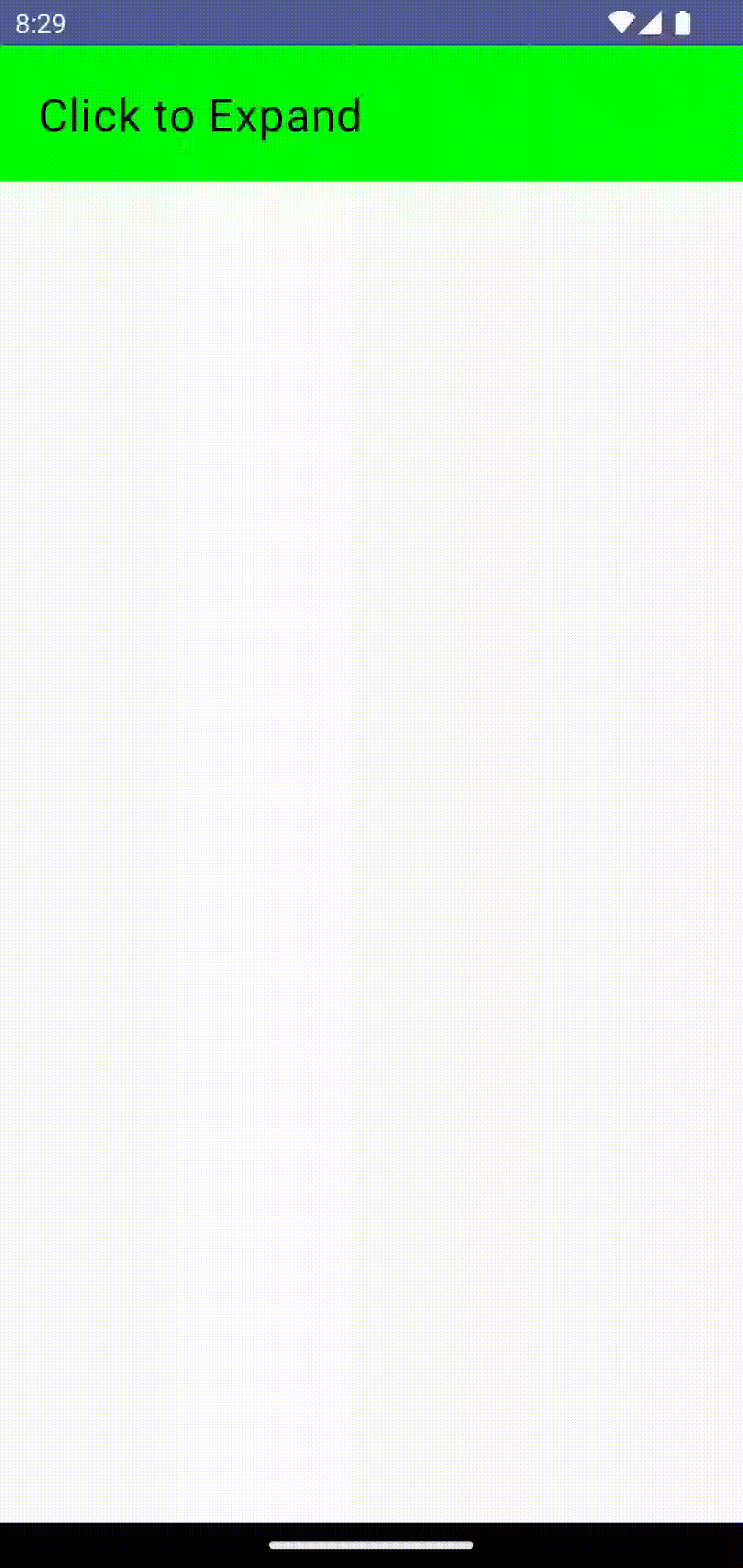
Following is the complete code for reference.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.animation.animateContentSize
import androidx.compose.animation.core.Spring
import androidx.compose.animation.core.spring
import androidx.compose.foundation.background
import androidx.compose.foundation.clickable
import androidx.compose.foundation.layout.*
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.getValue
import androidx.compose.runtime.setValue
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import androidx.compose.ui.unit.sp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
AnimationExample()
}
}
}
}
}
@Composable
fun AnimationExample() {
var expanded by remember { mutableStateOf(false) }
Column(Modifier.background(Color.Green).
animateContentSize(animationSpec = spring(
dampingRatio = Spring.DampingRatioMediumBouncy,
stiffness = Spring.StiffnessLow
)).fillMaxWidth().padding(20.dp))
{
Text(text = "Click to Expand",
modifier = Modifier.clickable { expanded = !expanded },
fontSize = 24.sp
)
if (expanded) {
Text(text = "On the other hand, we denounce with righteous indignation and dislike men who are so beguiled and demoralized by the charms of pleasure of the moment and trouble that are bound to ensue;")
}
}
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
AnimationExample()
}
}
That’s how you create spring animation in Jetpack Compose.