How to Add Multiple Transformations on Shapes in Jetpack Compose
In the world of Android development, Jetpack Compose has revolutionized the way developers create and manage UIs. One of its strengths lies in the ability to apply multiple transformations to shapes, allowing for more dynamic and visually appealing designs.
This blog post will delve into how to add multiple transformations to shapes in Jetpack Compose, enhancing the visual creativity of your apps.
Transformations in Jetpack Compose refer to altering the appearance of an object without changing its underlying data. These include translation (moving), rotation, scaling, and more. Applying multiple transformations can create complex and interesting visual effects.
Example: Combine Translation and Rotation
Let’s explore how to apply multiple transformations using Jetpack Compose’s Canvas API.
First, we define a Canvas
composable, which provides a space where we can draw and transform our shapes.
In the Canvas composable, we use the withTransform
block to apply our transformations. Here, we combine translation and rotation to transform a rectangle:
@Composable
fun Example() {
Canvas(modifier = Modifier.fillMaxSize()) {
withTransform({
translate(left = size.width / 5F)
rotate(degrees = 45F)
}) {
drawRect(
color = Color.Red,
topLeft = Offset(x = size.width / 3F, y = size.height / 3F),
size = size / 3F
)
}
}
}
In this code:
translate(left = size.width / 5F)
: Moves the drawing context to the right by one-fifth of the canvas width.rotate(degrees = 45F)
: Rotates the drawing context by 45 degrees.drawRect(...)
: Draws a rectangle with the specified color, position, and size. The transformations applied above affect how this rectangle is rendered.
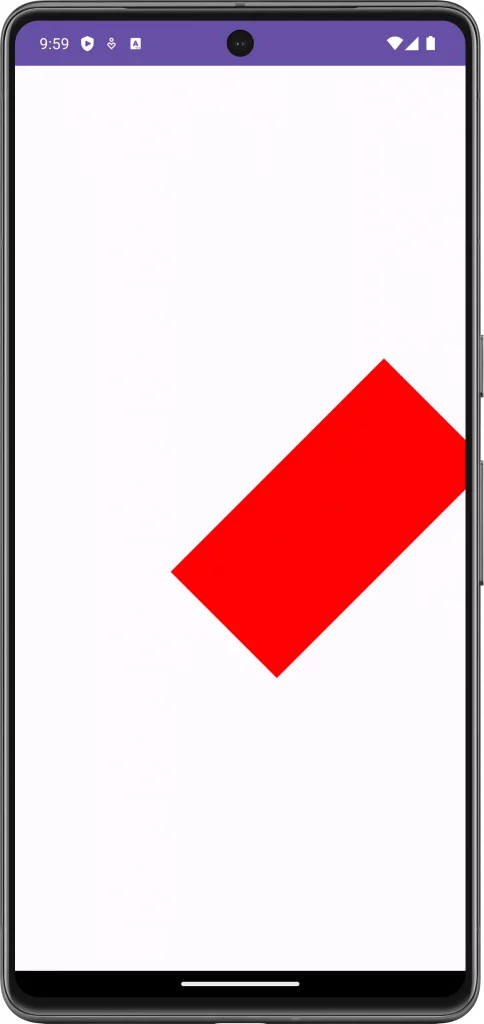
Following is the complete code for reference.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.Canvas
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.geometry.Offset
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.graphics.drawscope.withTransform
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Example()
}
}
}
}
}
@Composable
fun Example() {
Canvas(modifier = Modifier.fillMaxSize()) {
withTransform({
translate(left = size.width / 5F)
rotate(degrees = 45F)
}) {
drawRect(
color = Color.Red,
topLeft = Offset(x = size.width / 3F, y = size.height / 3F),
size = size / 3F
)
}
}
}
Customize Transformations
Adjust Translation and Rotation
You can modify the values passed to translate
and rotate
to change the position and orientation of your shape. Experimenting with different values can lead to interesting designs.
Combine with Other Transformations
Apart from translation and rotation, you can also include scaling or skewing within the withTransform
block to create more complex transformations.
Best Practices
Plan Your Transformations
It’s important to plan the order and values of your transformations. The order in which transformations are applied can significantly affect the final outcome.
Performance Considerations
While transformations allow for creative designs, they can be computationally expensive. Be mindful of performance, especially when dealing with complex transformations or animations.
Test Across Devices
Ensure that your transformed shapes look and function as expected across different devices and screen sizes. This ensures a consistent user experience.
Adding multiple transformations to shapes in Jetpack Compose opens up a world of creative possibilities for your app’s UI. By understanding how to effectively use these transformations, you can create visually striking designs that enhance the user experience of your Android apps.