How to Rotate Shape in Jetpack Compose
Creating dynamic and interactive UIs in Android applications often requires manipulating shapes. In this context, Jetpack Compose, the modern toolkit for building native UIs on Android, provides straightforward ways to rotate shapes.
This blog post will look into the steps and techniques for rotating a shape in Jetpack Compose.
Rotation in Jetpack Compose is performed using the DrawScope.rotate()
method. This method allows you to rotate your drawing operations around a specified pivot point.
Basic Rotation Example
Let’s consider a simple example: rotating a rectangle by 45 degrees. The steps are as follows:
- Setup a Canvas: First, set up a Canvas using
Canvas(modifier = Modifier.fillMaxSize())
. This canvas acts as your drawing area. - Apply Rotation: Inside the Canvas, use the
rotate
function and specify the degrees of rotation. For a 45-degree rotation, you would writerotate(degrees = 45F)
. - Drawing the Shape: Within the
rotate
block, define your shape. In this case, a rectangle is drawn withdrawRect
function. You can specify the color, size, and position of the rectangle. Example Code:
@Composable
fun Example() {
Canvas(modifier = Modifier.fillMaxSize()) {
rotate(degrees = 45F) {
drawRect(
color = Color.Red,
topLeft = Offset(x = size.width / 3F, y = size.height / 3F),
size = size / 3F
)
}
}
}
This code will rotate the rectangle by 45 degrees around the top-left corner of the rectangle.
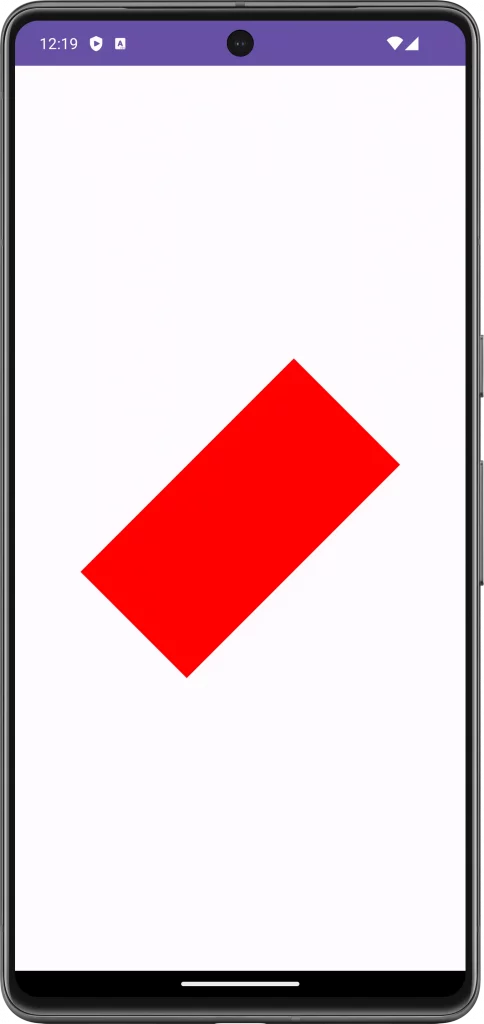
Following is the complete code for reference.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.Canvas
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.geometry.Offset
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.graphics.drawscope.rotate
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Example()
}
}
}
}
}
@Composable
fun Example() {
Canvas(modifier = Modifier.fillMaxSize()) {
rotate(degrees = 45F) {
drawRect(
color = Color.Red,
topLeft = Offset(x = size.width / 3F, y = size.height / 3F),
size = size / 3F
)
}
}
}
Advanced Rotation Techniques
The rotation in Jetpack Compose is around a pivot point. By default, the pivot is at the top-left corner of the canvas. You can change the pivot point as needed for your design.
Rotate Around a Custom Pivot Point
You can specify a custom pivot point for rotation. This allows for more control over how the shape rotates. For instance, rotating a rectangle around its center:
Canvas(modifier = Modifier.fillMaxSize()) {
val pivotX = size.width / 2
val pivotY = size.height / 2
rotate(degrees = 45F, pivot = Offset(pivotX, pivotY)) {
drawRect(
color = Color.Red,
topLeft = Offset(x = size.width / 4F, y = size.height / 4F),
size = size / 2F
)
}
}
Rotating shapes in Jetpack Compose is a straightforward yet powerful tool in your UI development arsenal. By understanding the basics of rotation and experimenting with advanced techniques, you can greatly enhance the visual appeal and interactivity of your Android apps.
One Comment