How to Add Checkbox Group in Android Jetpack Compose
Checkboxes are an integral part of UI design in any application. They are especially important in forms where multiple options need to be selected. Grouping these checkboxes can create a much more organized and user-friendly experience.
This blog post will guide you on how to implement a checkbox group in Jetpack Compose. In Jetpack Compose, creating a group of checkboxes can be achieved by combining multiple Checkbox composable functions and managing their checked states together.
Let’s dive into an example to understand this better:
@Composable
fun CheckboxExample() {
val selectedOptions = remember { mutableStateOf(setOf<String>()) }
val options = listOf("Option 1", "Option 2", "Option 3")
Column {
options.forEach { option ->
Row(
Modifier
.fillMaxWidth()
.padding(8.dp),
verticalAlignment = Alignment.CenterVertically
) {
Checkbox(
checked = selectedOptions.value.contains(option),
onCheckedChange = { selected ->
val currentSelected = selectedOptions.value.toMutableSet()
if (selected) {
currentSelected.add(option)
} else {
currentSelected.remove(option)
}
selectedOptions.value = currentSelected
Log.d("value", selectedOptions.value.toString())
}
)
Spacer(Modifier.width(8.dp))
Text(option)
}
}
}
}
In the above code, selectedOptions is a mutable state that keeps track of all selected options in our checkbox group. We loop over each option, and for each one, we create a Row composable containing a Checkbox and a Text composable. The Checkbox is marked as checked if its corresponding option is present in selectedOptions.
When the checked state of a checkbox is changed (i.e., a checkbox is selected or deselected), we update selectedOptions accordingly. If a checkbox is selected, we add its option to selectedOptions; if it’s deselected, we remove its option from selectedOptions.
This way, selectedOptions always contains all and only the options of the selected checkboxes, which effectively implements a checkbox group.
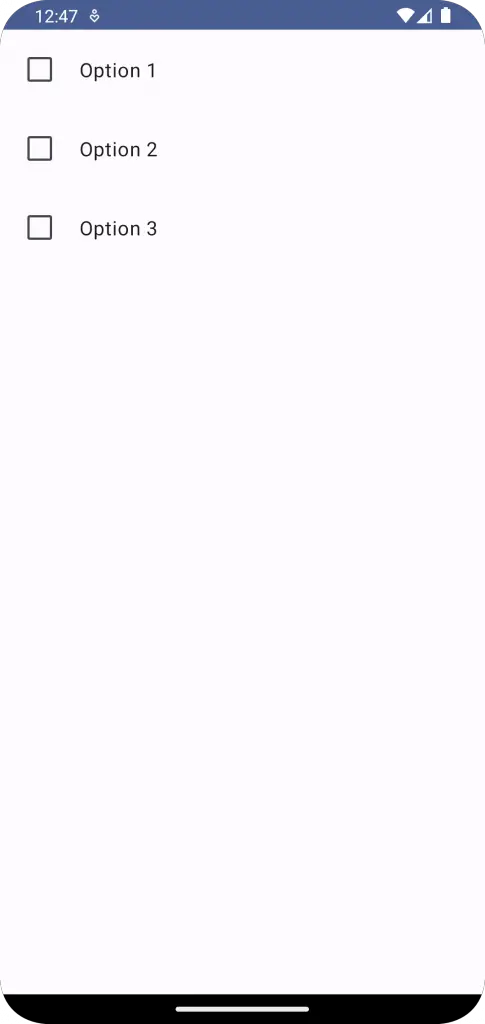
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import android.util.Log
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.Row
import androidx.compose.foundation.layout.Spacer
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.padding
import androidx.compose.foundation.layout.width
import androidx.compose.material3.Checkbox
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
CheckboxExample()
}
}
}
}
}
@Composable
fun CheckboxExample() {
val selectedOptions = remember { mutableStateOf(setOf<String>()) }
val options = listOf("Option 1", "Option 2", "Option 3")
Column {
options.forEach { option ->
Row(
Modifier
.fillMaxWidth()
.padding(8.dp),
verticalAlignment = Alignment.CenterVertically
) {
Checkbox(
checked = selectedOptions.value.contains(option),
onCheckedChange = { selected ->
val currentSelected = selectedOptions.value.toMutableSet()
if (selected) {
currentSelected.add(option)
} else {
currentSelected.remove(option)
}
selectedOptions.value = currentSelected
Log.d("value", selectedOptions.value.toString())
}
)
Spacer(Modifier.width(8.dp))
Text(option)
}
}
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
CheckboxExample()
}
}
As seen above, Jetpack Compose makes it quite straightforward to implement a group of checkboxes, thus enhancing the user experience. Stay tuned for more posts on Jetpack Compose and its powerful capabilities!