How to Change Snackbar Background Color in Android Jetpack Compose
When building Android applications, you often need to give your users feedback about the outcome of an operation. This is usually done via snackbars – a brief, non-intrusive pop-up that displays a message. Jetpack Compose simplifies the process of creating and customizing these snackbars.
In this blog post, we walk you through the steps of changing the background color of a Snackbar in Jetpack Compose.
How to Customize Snackbar Background Color
You can add Snackbar composable to SnackbarHost. Then you can change the color using the containerColor parameter. See the Snackbar example given below.
@Composable
fun SnackbarExample() {
val snackbarHostState = remember { SnackbarHostState() }
val scope = rememberCoroutineScope()
Box {
Button(onClick = {
scope.launch {
val result = snackbarHostState.showSnackbar(
message = "Snackbar Example",
actionLabel = "Action",
withDismissAction = true,
duration = SnackbarDuration.Indefinite
)
when (result) {
SnackbarResult.ActionPerformed -> {
//Do Something
}
SnackbarResult.Dismissed -> {
//Do Something
}
}
}},
modifier = Modifier
.fillMaxSize()
.wrapContentSize()) {
Text(text = "Show Snackbar")
}
SnackbarHost(
hostState = snackbarHostState,
modifier = Modifier.align(Alignment.BottomCenter)
) {
Snackbar(
snackbarData = it,
containerColor = Color.Red,
)
}
}
}
By adding backgroundColor = Color.Red, the Snackbar’s background color will be changed to red. You can replace Color.Red with any color you want.
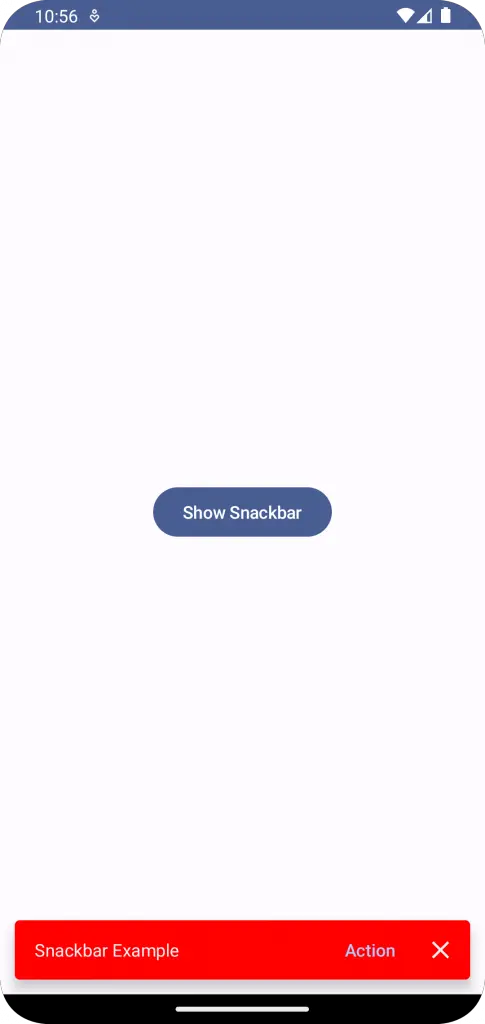
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Box
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.wrapContentSize
import androidx.compose.material3.Button
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Snackbar
import androidx.compose.material3.SnackbarDuration
import androidx.compose.material3.SnackbarHost
import androidx.compose.material3.SnackbarHostState
import androidx.compose.material3.SnackbarResult
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.runtime.remember
import androidx.compose.runtime.rememberCoroutineScope
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import com.example.example.ui.theme.ExampleTheme
import kotlinx.coroutines.launch
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
SnackbarExample()
}
}
}
}
}
@Composable
fun SnackbarExample() {
val snackbarHostState = remember { SnackbarHostState() }
val scope = rememberCoroutineScope()
Box {
Button(onClick = {
scope.launch {
val result = snackbarHostState.showSnackbar(
message = "Snackbar Example",
actionLabel = "Action",
withDismissAction = true,
duration = SnackbarDuration.Indefinite
)
when (result) {
SnackbarResult.ActionPerformed -> {
//Do Something
}
SnackbarResult.Dismissed -> {
//Do Something
}
}
}},
modifier = Modifier
.fillMaxSize()
.wrapContentSize()) {
Text(text = "Show Snackbar")
}
SnackbarHost(
hostState = snackbarHostState,
modifier = Modifier.align(Alignment.BottomCenter)
) {
Snackbar(
snackbarData = it,
containerColor = Color.Red,
)
}
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
SnackbarExample()
}
}
In conclusion, Jetpack Compose offers a simple, flexible way to customize your Snackbar’s look and feel. By using the backgroundColor parameter, you can quickly adjust the background color of your Snackbar to match your application’s theme.