How to Center Align UI Elements in Android Jetpack Compose
Alignment is an important aspect of designing a mobile app. In this Jetpack Compose tutorial, let’s learn how to center vertically, center horizontally, and center both ways.
We use Column and its parameter horizontalAlignment to make the children center horizontally to the parent.
@Composable
fun Example() {
Column(horizontalAlignment = Alignment.CenterHorizontally, modifier = Modifier.fillMaxSize()) {
Text(text = "Center Alignment")
}
}
The text will be centered horizontally as seen in the output.
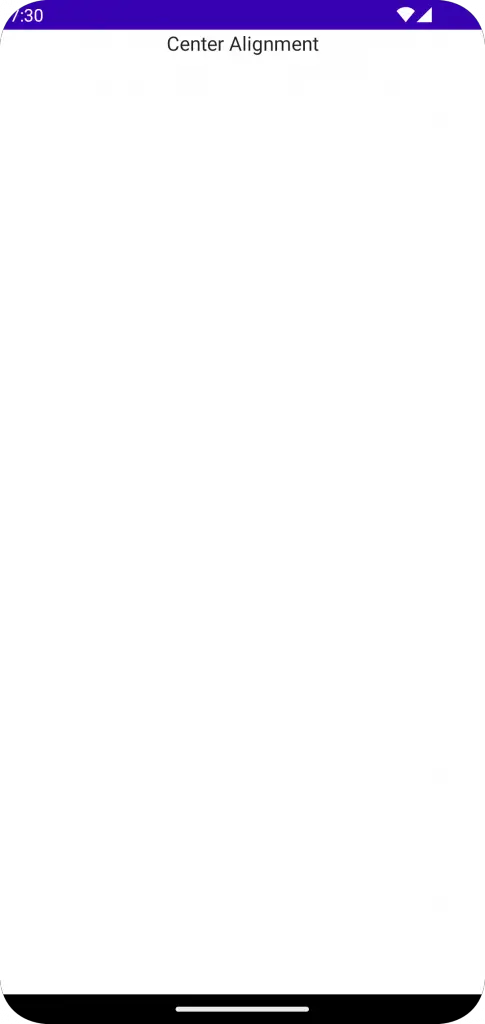
You can align children to the center vertically using verticalAlignment parameter. See the code snippet given below.
@Composable
fun Example() {
Column(verticalArrangement = Arrangement.Center, modifier = Modifier.fillMaxSize()) {
Text(text = "Center Alignment")
}
}
The Text will be center aligned vertically.
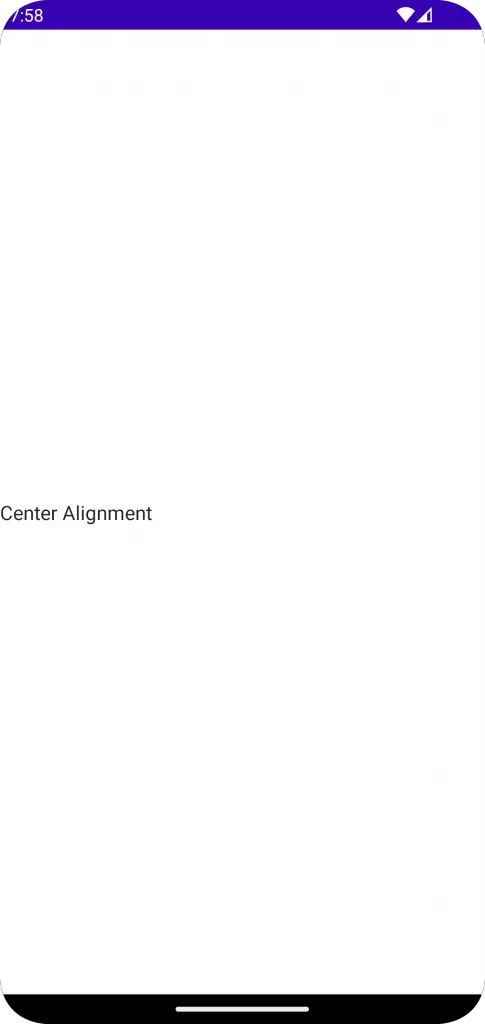
The next thing is to center align elements horizontally and vertically.
@Composable
fun Example() {
Column(horizontalAlignment = Alignment.CenterHorizontally,verticalArrangement = Arrangement.Center,
modifier = Modifier.fillMaxSize()) {
Text(text = "Center Alignment")
}
}
And following is the output.
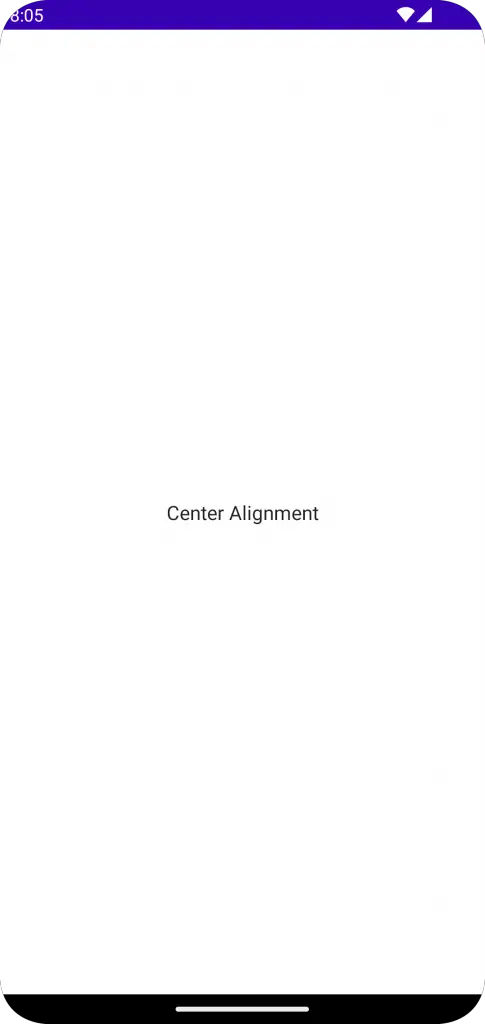
Complete Code
Following is the complete code for reference.
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Arrangement
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
Example()
}
}
}
}
}
}
@Composable
fun Example() {
Column(horizontalAlignment = Alignment.CenterHorizontally,verticalArrangement = Arrangement.Center,
modifier = Modifier.fillMaxSize()) {
Text(text = "Center Alignment")
}
}
I hope this tutorial is helpful for you.