How to add Text with all Caps in Android Jetpack Compose
Styling text is an important aspect of designing mobile apps. Sometimes, we display all text in capital letters to show the significance. Let’s learn how to capitalize text in Jetpack Android.
The Text composable doesn’t have a built-in property to change text case. Hence, we use the Kotlin function uppercase() for this purpose. See the code snippet given below.
@Composable
fun TextExample() {
Text(text = ("This is an example of text with caps all").uppercase(),
fontSize = 24.sp)
}
Following is the output.
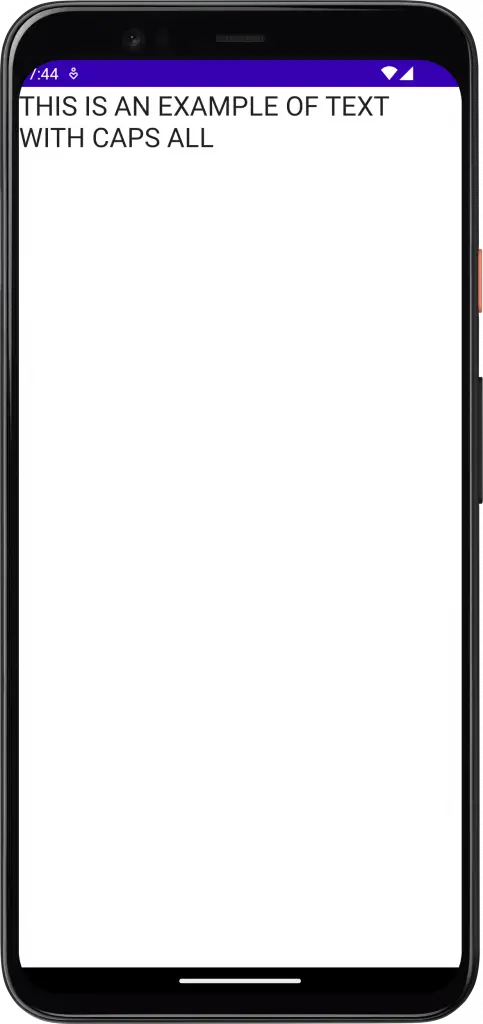
Complete Code
Following is the complete code.
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.unit.sp
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
TextExample()
}
}
}
}
}
}
@Composable
fun TextExample() {
Text(text = ("This is an example of text with caps all").uppercase(),
fontSize = 24.sp)
}
If you want to change text letter spacing then see this tutorial. I hope this Jetpack Compose Text Capitalize tutorial is helpful for you.