How to add Text Background Color in Android Jetpack Compose
In some rare scenarios, mobile app developers prefer to set a background color for text in their apps. Let’s learn how to add Text with a custom background color in Jetpack Compose.
Jetpack Compose Text Background Color
The TextStyle helps to set a custom background color to the Text composable. See the code snippet given below.
@Composable
fun TextExample() {
Text(
style = TextStyle(background = Color.Yellow),
modifier = Modifier.padding(20.dp),
text = "This is a sample text. This is an example of adding background color to the text.",
fontSize = 24.sp)
}
The output is given below.
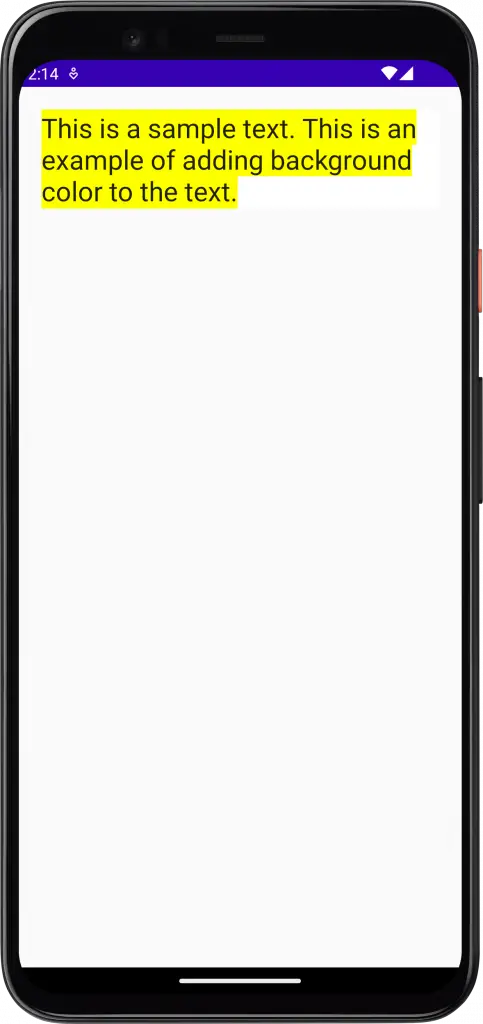
Complete Code
Following is the complete code for reference.
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.padding
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.text.TextStyle
import androidx.compose.ui.unit.dp
import androidx.compose.ui.unit.sp
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
TextExample()
}
}
}
}
}
}
@Composable
fun TextExample() {
Text(
style = TextStyle(background = Color.Yellow),
modifier = Modifier.padding(20.dp),
text = "This is a sample text. This is an example of adding background color to the text.",
fontSize = 24.sp)
}
I hope this Jetpack Compose Text background color tutorial is helpful for you.