How to Draw Points on Jetpack Compose Canvas
Jetpack Compose, Google’s modern toolkit for UI development in Android, offers developers a canvas for creative and custom drawings. Among its features is the ability to draw points, a fundamental aspect of graphics programming.
This blog post will look into how to draw points in Jetpack Compose Canvas.
Canvas in Jetpack Compose provides a lower-level API for drawing shapes, text, images, and more. It’s a versatile tool that can be used for custom drawings, like plotting points, which is essential for creating graphics, charts, or custom designs.
Draw Points on Canvas
Set Up the Canvas
To start, we need to create a Canvas composable. This will serve as our drawing surface.
@Composable
fun CanvasExample() {
Canvas(modifier = Modifier.fillMaxSize()) {
// Point drawing code will go here
}
}
Draw Points
In the Canvas, you can draw points using the drawPoints
function. This function provides flexibility in terms of how the points are displayed.
val points = listOf(
Offset(100f, 100f),
Offset(200f, 200f),
Offset(300f, 300f)
)
drawPoints(
points = points,
pointMode = PointMode.Points,
color = Color.Red,
strokeWidth = 50f,
cap = StrokeCap.Round
)
In this example:
points
: A list ofOffset
objects representing the coordinates of each point.drawPoints(...)
: This function draws the points on the canvas.pointMode = PointMode.Points
: Specifies how points should be drawn (as individual points, lines, or a polygon).color = Color.Red
: The color of the points.strokeWidth = 5f
: The size of each point.cap = StrokeCap.Round
: The shape of each point (round in this case).
Following is the complete code for reference.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.Canvas
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.geometry.Offset
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.graphics.PointMode
import androidx.compose.ui.graphics.StrokeCap
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
ExampleCanvas()
}
}
}
}
}
}
@Composable
fun ExampleCanvas() {
Canvas(modifier = Modifier.fillMaxSize()) {
val points = listOf(
Offset(100f, 100f),
Offset(200f, 200f),
Offset(300f, 300f)
)
drawPoints(
points = points,
pointMode = PointMode.Points,
color = Color.Red,
strokeWidth = 50f,
cap = StrokeCap.Round
)
}
}
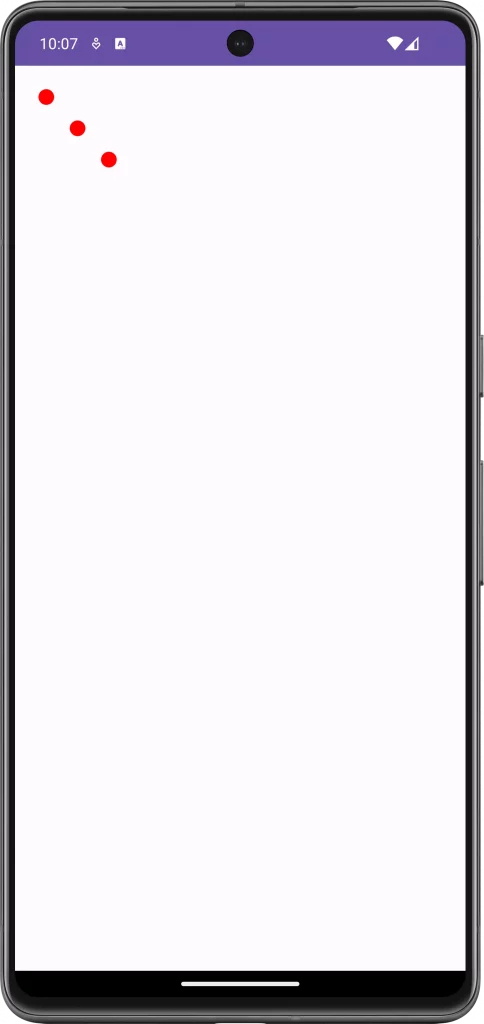
PointMode Variations
PointMode.Points
: Draws individual points.PointMode.Lines
: Connects points with lines.PointMode.Polygon
: Connects all points in a continuous line, forming a polygon.
Customize Point Drawings
Change Point Color and Size
You can customize the color and size of the points by modifying the color
and strokeWidth
parameters, respectively.
Use Advanced Features
drawPoints
also supports other parameters like pathEffect
for creating dashed lines or patterns when using PointMode.Lines
or PointMode.Polygon
.
Drawing points in Jetpack Compose Canvas is a straightforward yet powerful way to add custom graphics to your Android apps. Whether you’re creating simple designs or complex data visualizations, the flexibility of Jetpack Compose Canvas provides the tools you need to bring your creative visions to life.