How to Add Background Image to Box in Android Jetpack Compose
In Jetpack Compose, Box is a layout composable that positions its children relative to its edges. It is very flexible and can be used to create various types of designs, including a box with a background image.
In this tutorial, we are going to learn how to set a background image to a Box in Jetpack Compose.
First, we need to add our image resource to the project. You can add your image to the res/drawable directory of your project.
Once we have our image in place, we can now create a Box with a background image. In the example below, In this case, we’ll use the Image composable to display the image, and the Box will be used to overlay text over this image.
@Composable
fun BoxExample() {
Column {
Box(
modifier = Modifier
.fillMaxWidth().height(200.dp),
contentAlignment = Alignment.Center
) {
Image(
painter = painterResource(id = R.drawable.nature),
modifier = Modifier.fillMaxSize(),
contentDescription = "Background Image",
contentScale = ContentScale.Crop
)
Text(
text = "Coding with Rashid",
style = TextStyle(color= Color.Red,fontSize = 24.sp)
)
}
}
}
In the example above, we’re creating a Box that expands to fill the maximum width and has a height of 200.dp. Inside this Box, we have an Image composable that fills up the entire box. The painterResource function is used to load our image, and ContentScale.Crop is used to adjust the image’s scaling within the Box.
Overlaying the image, we place a Text composable which is centered inside the Box due to the contentAlignment = Alignment.Center parameter. The text has a red color and a font size of 24.sp.
Following is the output.
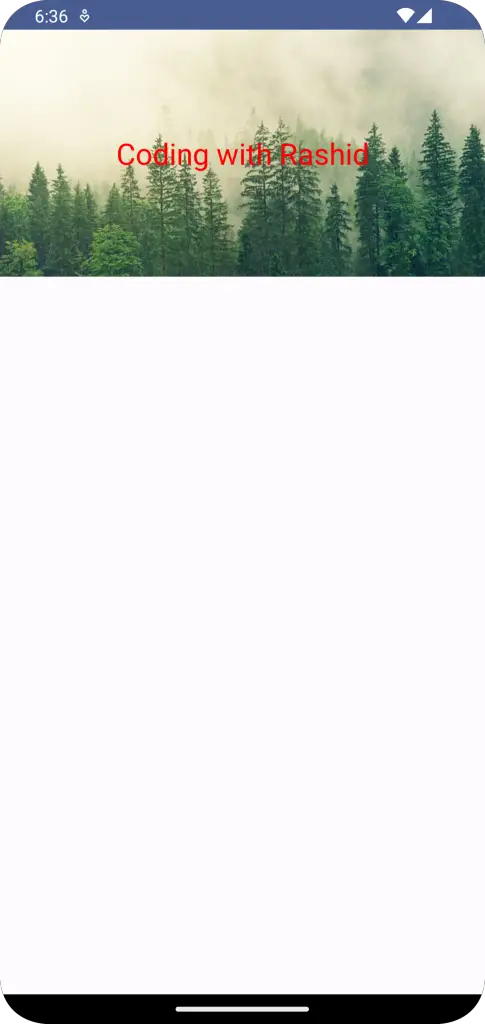
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.Image
import androidx.compose.foundation.layout.Box
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.height
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.layout.ContentScale
import androidx.compose.ui.res.painterResource
import androidx.compose.ui.text.TextStyle
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import androidx.compose.ui.unit.sp
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
BoxExample()
}
}
}
}
}
@Composable
fun BoxExample() {
Column{
Box(
modifier = Modifier
.fillMaxWidth().height(200.dp),
contentAlignment = Alignment.Center
) {
Image(
painter = painterResource(id = R.drawable.nature),
modifier = Modifier.fillMaxSize(),
contentDescription = "Background Image",
contentScale = ContentScale.Crop
)
Text(
text = "Coding with Rashid",
style = TextStyle(color= Color.Red,fontSize = 24.sp)
)
}
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
BoxExample()
}
}
With Jetpack Compose, creating such complex views is now straightforward and intuitive. Box allows for creating overlapping views easily, and it’s just one of the many advantages that Jetpack Compose brings to the table.