How to Implement Scrolling in Android Jetpack Compose
Jetpack Compose has revolutionized the way we think about Android UI development. Among its many features, the scrolling capability is a key component that enables smooth user experiences. This article will delve deep into two scroll modifiers available in Jetpack Compose: verticalScroll
and horizontalScroll
.
Jetpack Compose Scroll Modifiers
Jetpack Compose offers two basic types of scroll modifiers. The verticalScroll
modifier lets you scroll content up and down. On the other hand, horizontalScroll
allows for left and right movements.
Basic Code Snippet for Vertical Scrolling
Before diving into a complete example, let’s look at a simple code snippet that shows how to use the verticalScroll
modifier.
@Composable
fun ScrollItems() {
Column(
modifier = Modifier
.verticalScroll(rememberScrollState()).fillMaxSize()
) {
repeat(20) {
Text("Item $it", modifier = Modifier.padding(20.dp))
}
}
}
In this snippet, we create a column of 20 list items that you can scroll through vertically.
A Complete Example with Code
Now, let’s explore a complete example that incorporates everything we’ve learned so far.
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.padding
import androidx.compose.foundation.rememberScrollState
import androidx.compose.foundation.verticalScroll
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.unit.dp
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
ScrollItems()
}
}
}
}
}
}
@Composable
fun ScrollItems() {
Column(
modifier = Modifier.verticalScroll(rememberScrollState()).fillMaxSize()
) {
repeat(20) {
Text("Item $it", modifier = Modifier.padding(20.dp))
}
}
}
In this example, you will see the full code required to create a vertically scrollable list using Jetpack Compose.
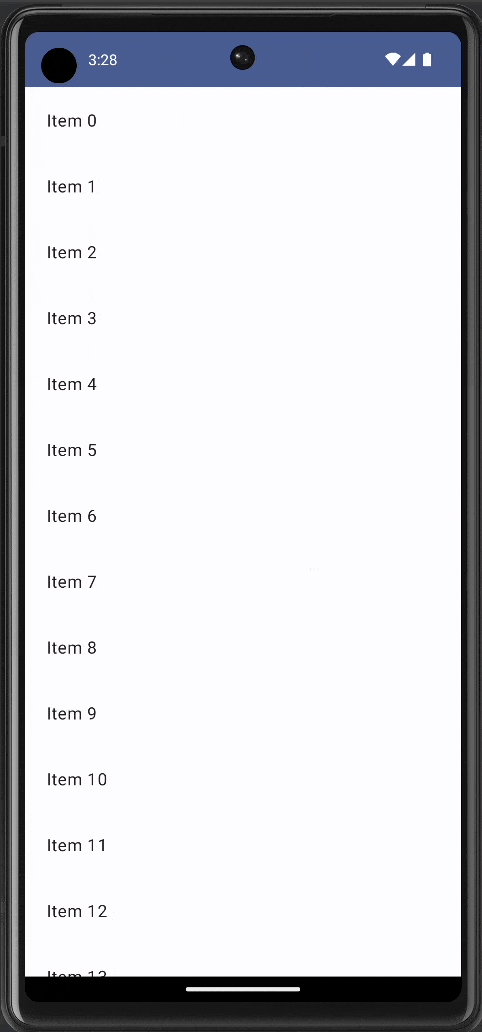
Implementing basic scrolling in Android Jetpack Compose is pretty straightforward. By using the verticalScroll
and horizontalScroll
modifiers, you can enable scrolling in just a few lines of code. This feature makes Jetpack Compose even more flexible and user-friendly.