How to Change Switch Size in Jetpack Compose
In Android’s Jetpack Compose, customizing UI components is a common requirement. One such customization is altering the size of a Switch
. This post looks into methods for resizing a Switch
in Jetpack Compose, enhancing its appearance and usability.
Switch in Jetpack Compose
A Switch
in Jetpack Compose is used for toggling between two states. By default, it comes with a predefined size. Customizing its size can be crucial for certain design requirements.
Resize Switch with Modifier.scale
Set Up Your Composable Function:
First, we define a @Composable
function named MySwitch
. This function will include our switch component.
@Composable
fun MySwitch() {
// Switch logic goes here
}
Manage the Switch State:
We use remember
and mutableStateOf
to manage the switch’s state, indicating whether it’s checked (ON) or not (OFF). Here, we initialize it to true
(ON).
var isChecked by remember { mutableStateOf(true) }
Implement the Switch with Modified Size:
The key part is resizing the switch. We achieve this by applying the Modifier.scale
modifier. This modifier adjusts the size of the switch based on the provided scale factor. In this example, we use a scale factor of 1.5f
, which enlarges the switch by 50%.
Switch(
checked = isChecked,
onCheckedChange = { isChecked = it },
modifier = Modifier.scale(1.5f)
)
Full Code Example
Here’s the complete MySwitch
composable function:
@Composable
fun MySwitch() {
var isChecked by remember { mutableStateOf(true) }
Switch(
checked = isChecked,
onCheckedChange = { isChecked = it },
modifier = Modifier.scale(1.5f)
)
}
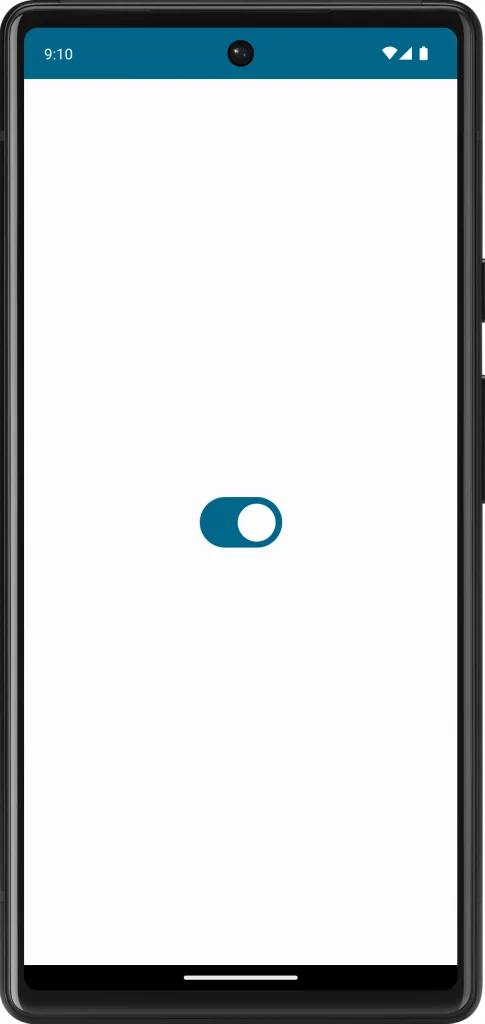
Best Practices and Considerations
- Consistency: Ensure that the size of the switch is consistent with other interactive elements in your app.
- Accessibility: Larger switches can be more accessible, especially for users with motor impairments.
- Testing: Always test on different screen sizes and resolutions to ensure the switch looks and functions as intended.
Customizing the size of a switch in Jetpack Compose is straightforward and enhances the usability and aesthetic of your app. By using the Modifier.scale
modifier, you can easily adjust the switch size to meet your design requirements.