How to Apply Image Background to Text in Android Jetpack Compose
Textual content is a critical component in any app, but who said it has to be plain? Adding an image background to your text can make it pop and catch the user’s attention.
This blog post will guide you through the process of setting an image background to text using Jetpack Compose.
In Jetpack Compose, you can add image backgrounds to text using ImageShader and ShaderBrush. ImageShader allows you to use an image as a shader, while ShaderBrush uses this shader to paint the text.
Let’s look at an example where we apply an image background to a Text composable.
fun TextExample() {
val imageBrush =
ShaderBrush(ImageShader(ImageBitmap.imageResource(id = R.drawable.my_image)))
Text(
text = "Hello!",
style = TextStyle(
brush = imageBrush,
fontWeight = FontWeight.ExtraBold,
fontSize = 118.sp
)
)
}
In this example, we start by creating an ImageShader using ImageBitmap.imageResource, which fetches the image resource (in this case, ‘my_image’) and uses it as the shader. This shader is then wrapped in a ShaderBrush to create imageBrush.
The Text composable takes a string and a TextStyle. In the TextStyle, we pass our imageBrush to the brush parameter. This tells the text to use the image brush for painting. We also set the font weight to ExtraBold and the font size to 36.sp to make the text more noticeable.
Following is the output.
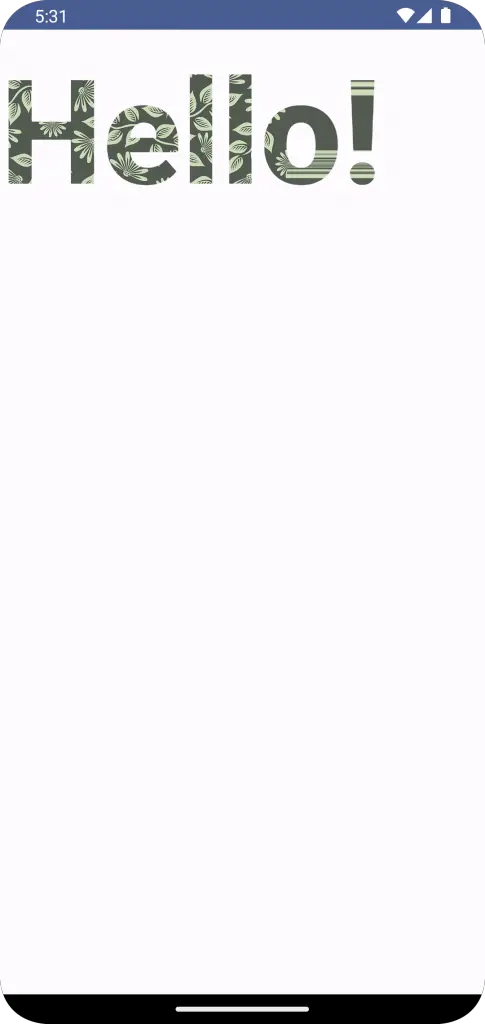
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.ImageBitmap
import androidx.compose.ui.graphics.ImageShader
import androidx.compose.ui.graphics.ShaderBrush
import androidx.compose.ui.res.imageResource
import androidx.compose.ui.text.ExperimentalTextApi
import androidx.compose.ui.text.TextStyle
import androidx.compose.ui.text.font.FontWeight
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.sp
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
TextExample()
}
}
}
}
}
@OptIn(ExperimentalTextApi::class)
@Composable
fun TextExample() {
val imageBrush =
ShaderBrush(ImageShader(ImageBitmap.imageResource(id = R.drawable.my_image)))
Text(
text = "Hello!",
style = TextStyle(
brush = imageBrush,
fontWeight = FontWeight.ExtraBold,
fontSize = 118.sp
)
)
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
TextExample()
}
}
Adding image backgrounds to text can be an excellent way to make your app stand out. It’s particularly useful for titles, headings, and any text that you want to highlight. However, remember to use it sparingly and ensure the text remains readable.