How to Create Gradient TextField in Android Jetpack Compose
If you are developing a fancy app with some cool styles then you may want to apply gradient colors to different UI elements. In this Jetpack Compose tutorial, we are learning how to give gradient color to TextField.
Looking to make your app stand out with vibrant colors? Gradient colors can make your UI elements look dynamic and engaging. In this Jetpack Compose tutorial, you’ll learn how to apply gradient colors to a TextField
.
Why Use Gradients?
Gradients offer a smooth transition between multiple colors. This creates a visually appealing effect that can engage users more than a solid color.
Prerequisites
Before diving in, make sure you’ve set up a Jetpack Compose project. We’re also using the Brush API, which is currently experimental in the Compose library.
How the Brush API Works
The Brush API in Jetpack Compose allows for a range of gradient styles. From linear to radial gradients, you have multiple options. Note that using Brush in TextStyle
is still in the experimental phase.
Brush API helps to create a variety of color gradients in compose. See the following code snippet.
@Composable
fun TextFieldExample() {
var textInput by remember { mutableStateOf("")}
TextField(value = textInput,
onValueChange = {textInput = it},
placeholder = { Text("Sample Text") },
textStyle = TextStyle(brush = Brush.linearGradient(
colors = listOf(Color.Magenta, Color.Cyan))),
modifier = Modifier.fillMaxWidth()
.padding(10.dp))
}
As you see we use Brush.linearGradient to create a linear gradient of two colors namely magenta and cyan.
Complete Code
Below is an example of how to apply gradient colors to a TextField
using Jetpack Compose.
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.padding
import androidx.compose.material3.ExperimentalMaterial3Api
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.material3.TextField
import androidx.compose.runtime.Composable
import androidx.compose.runtime.getValue
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.setValue
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Brush
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.text.ExperimentalTextApi
import androidx.compose.ui.text.TextStyle
import androidx.compose.ui.unit.dp
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
TextFieldExample()
}
}
}
}
}
}
@OptIn(ExperimentalMaterial3Api::class, ExperimentalTextApi::class)
@Composable
fun TextFieldExample() {
var textInput by remember { mutableStateOf("")}
TextField(value = textInput,
onValueChange = {textInput = it},
placeholder = { Text("Sample Text") },
textStyle = TextStyle(brush = Brush.linearGradient(
colors = listOf(Color.Magenta, Color.Cyan))),
modifier = Modifier.fillMaxWidth()
.padding(10.dp))
}
Output
After implementing the above code, you’ll see gradient colors when you type text into the TextField
. The gradient will transition smoothly between Magenta and Cyan.
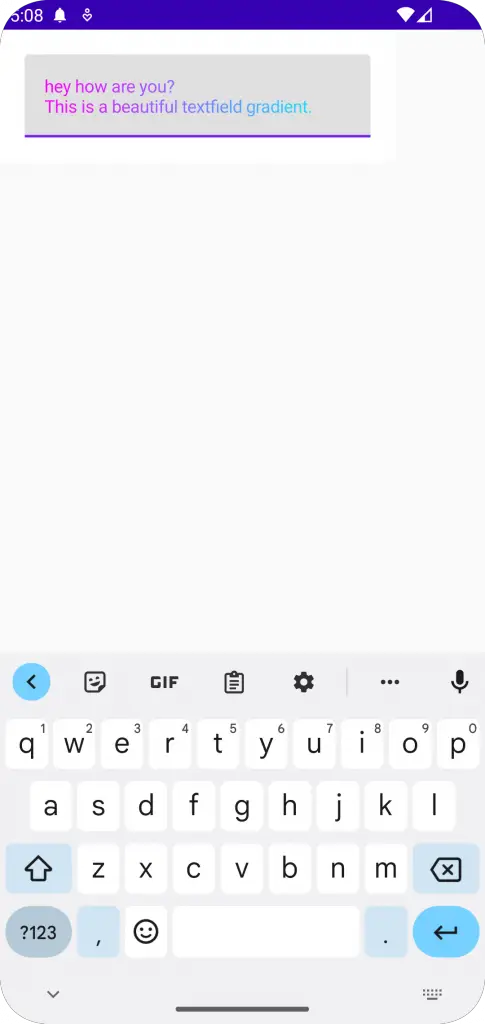
Using gradient colors in your app’s text fields can add an extra flair to your user interface. Although it’s an experimental feature, it’s a nifty one that can elevate your app’s look and feel.