How to Add Text with Gradient Color in Android Jetpack Compose
When it comes to UI design, using gradient colors can add depth and richness to your app’s look and feel. In this Android development tutorial, we will learn how to create a gradient text in Jetpack Compose using the Brush API.
Note: The usage of Brush in the TextStyle is currently experimental and may be subject to change in the future.
We can create different types of gradients using Brush API. Using the Text composable with Brush will give us the desired result.
See the code snippet given below.
@OptIn (ExperimentalTextApi::class)
@Composable
fun GradientText() {
Text(
fontSize = 30.sp,
text = ("This text has a beautiful gradient color. Just Check it!"),
style = TextStyle(
brush = Brush.linearGradient(
colors = listOf(Color.Magenta, Color.Cyan)
)
)
)
}
Here, we have created a Text composable with a font size of 30sp and added a custom TextStyle with a linear gradient Brush. We have specified the start and end colors for the gradient using a list of Color objects.
You will get the following output.
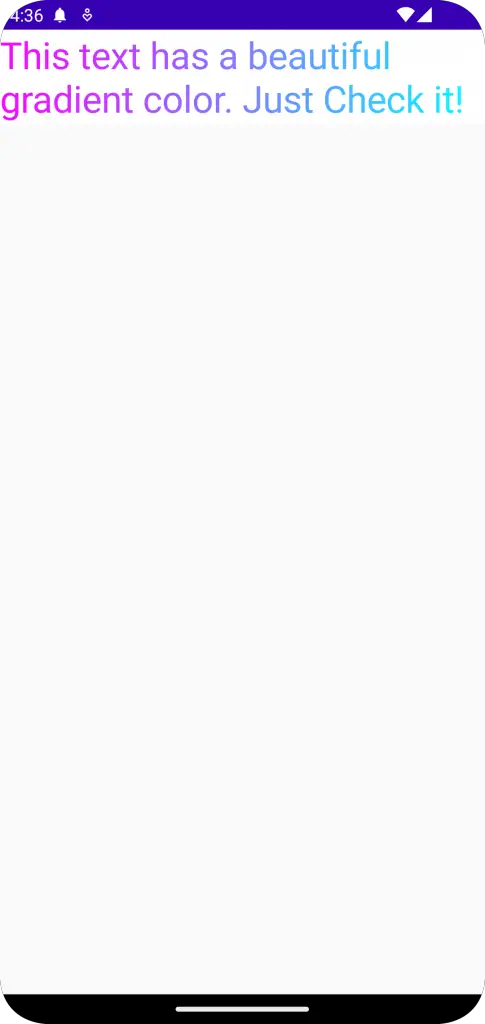
Following is the complete code for gradient text in Jetpack Compose.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.*
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.*
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Brush
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.text.*
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.sp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
GradientText()
}
}
}
}
}
@OptIn (ExperimentalTextApi::class)
@Composable
fun GradientText() {
Text(
fontSize = 30.sp,
text = ("This text has a beautiful gradient color. Just Check it!"),
style = TextStyle(
brush = Brush.linearGradient(
colors = listOf(Color.Magenta, Color.Cyan)
)
)
)
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
GradientText()
}
}
That’s how you add gradient text in Jetpack Compose.
In this tutorial, we have learned how to apply gradient colors to text in Jetpack Compose using the Brush API. While this feature is currently experimental, it allows for some exciting new possibilities in UI design.
One Comment