How to Change Font Size of Text in Android Jetpack Compose
Jetpack Compose is considered as a productive tool to create UI for Android apps. In this Android app development tutorial, let’s see how to change the font size of a Text composable.
The Text composable is a fundamental building block in Jetpack Compose that allows you to render text in your Android app. With Text, you can easily customize the font, color, size, and other styling properties of your text.
The Text composable has many parameters. You have to use the fontSize parameter to change the font size of a given text. See the code snippet given below.
@Composable
fun TextExample() {
Text("Happy Birthday!", fontSize = 32.sp)
}
The Text composable is used to display the text, and it takes two parameters. The first parameter is a string that represents the text to be displayed, which is “Happy Birthday!” in this example.
The second parameter is an optional fontSize parameter that specifies the size of the text. In this case, the fontSize is set to 32.scaledPixels (sp).
You will get the following output.
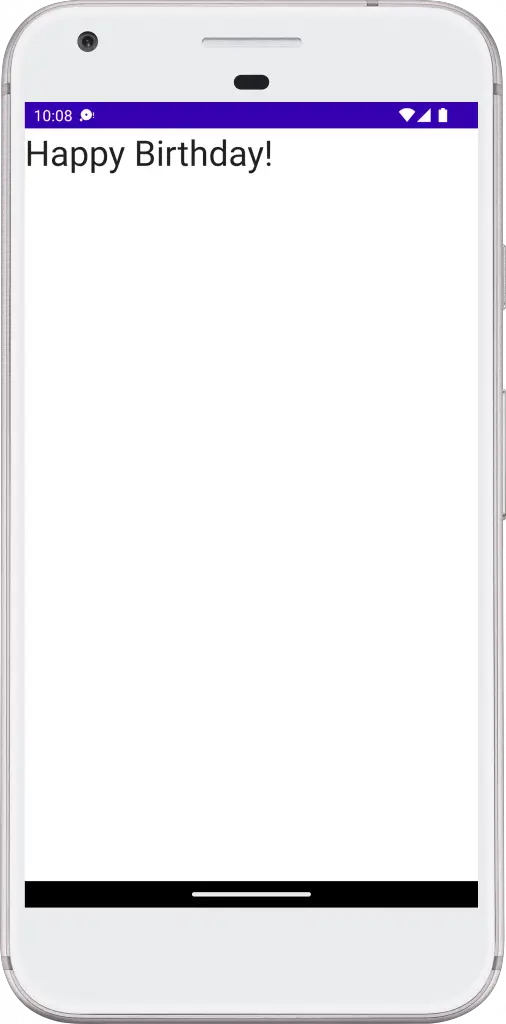
Following is the complete code for reference.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.*
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.*
import androidx.compose.ui.Modifier
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.sp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
TextExample()
}
}
}
}
}
@Composable
fun TextExample() {
Text("Happy Birthday!", fontSize = 32.sp)
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
TextExample()
}
}
That’s how you change Text font size in Jetpack Compose. I hope this Jetpack Compose tutorial for Android app development will be helpful for you.
2 Comments