How to Arrange Elements Horizontally Using Android Jetpack Compose
Jetpack compose helps you to create your Android app’s UI in a more flexible and intuitive way. With Compose, you can create beautiful, responsive user interfaces with less code than traditional XML layouts.
Let’s learn how to arrange your UI components horizontally using Jetpack composable functions in this Android app development tutorial.
Arranging a set of components in a horizontal direction can be attained by using Jetpack composable Row. You just need to wrap your components with Row composable. See the code snippet given below.
@Composable
fun RowExample() {
Row {
Text(text = "Hello ")
Text(text = "World!")
}
}
In this example, we have two Text components that will be arranged horizontally. The result is a row with the text “Hello World!”.
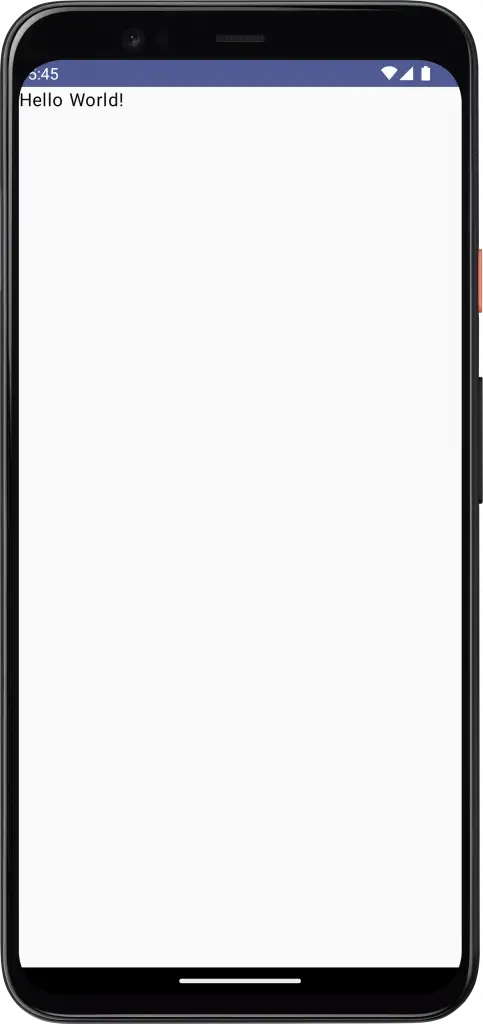
But what if we want to add more elements to the row? We can simply add them as additional child components.
@Composable
fun RowExample() {
Row {
Text(text = "Hello ")
Text(text = "World!")
Button(onClick = { /* Do something */ }) {
Text(text = "Click me!")
}
}
}
In this example, we’ve added a Button component to the row. The result is a row with the text “Hello World!” and a button.
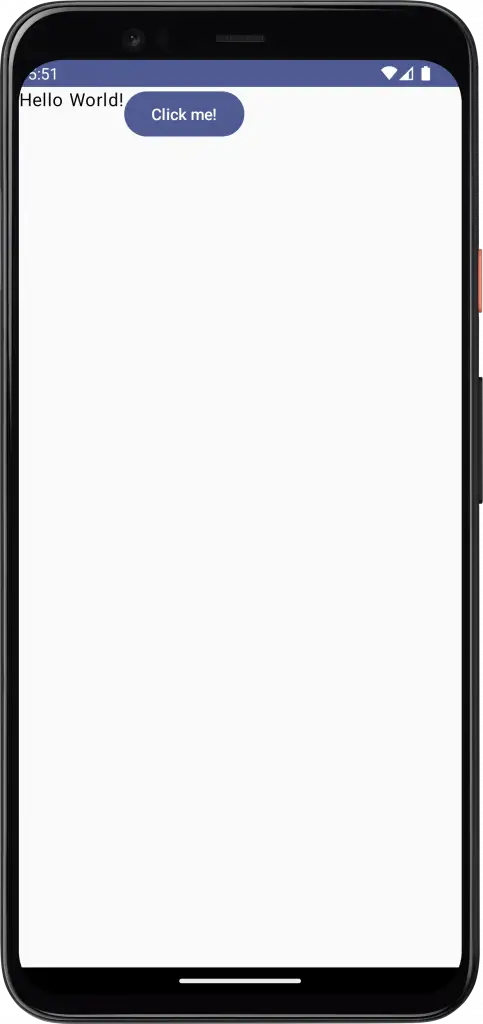
If we want to add spacing between the elements in the row, we can use the horizontalArrangement parameter and apply Arrangement.SpaceBetween.
@Composable
fun RowExample() {
Row(modifier = Modifier.fillMaxWidth(),
horizontalArrangement = Arrangement.SpaceBetween) {
Text(text = "Hello ")
Text(text = "World!")
Button(onClick = { /* Do something */ }) {
Text(text = "Click me!")
}
}
}
Following is the output.
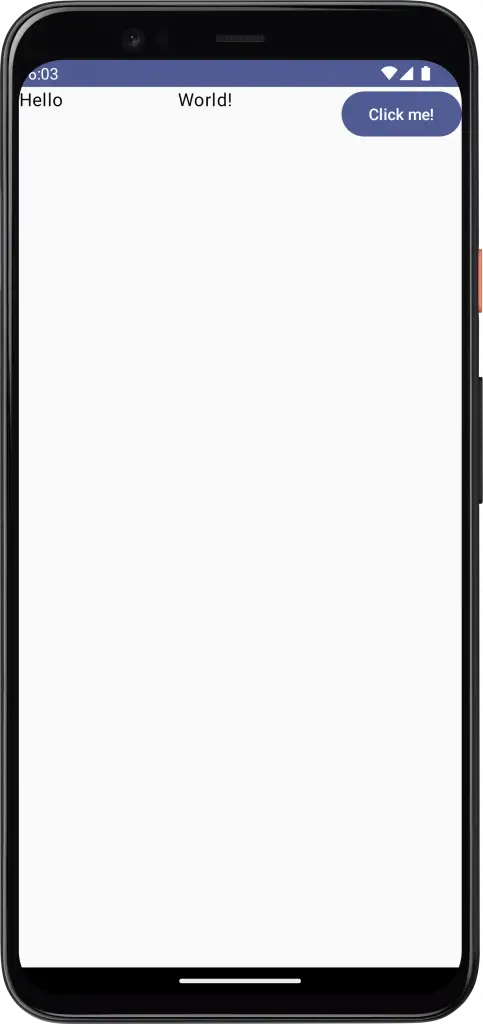
The complete code is given below for reference.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.*
import androidx.compose.material3.Button
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.*
import androidx.compose.ui.Modifier
import androidx.compose.ui.tooling.preview.Preview
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
RowExample()
}
}
}
}
}
@Composable
fun RowExample() {
Row(modifier = Modifier.fillMaxWidth(),
horizontalArrangement = Arrangement.SpaceBetween) {
Text(text = "Hello ")
Text(text = "World!")
Button(onClick = { /* Do something */ }) {
Text(text = "Click me!")
}
}
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
RowExample()
}
}
That’s how you arrange UI elements horizontally using Jetpack Compose. If you are looking to arrange elements vertically then you can use the Column composable.
In this post, we’ve explored how to arrange elements horizontally using Jetpack Compose. By using the Row component, we can easily create responsive, horizontal layouts with less code than traditional XML layouts.
One Comment