How to Display GIF Image in Android Jetpack Compose
GIFs are a great way to convey emotions and reactions, and they can be used to add visual interest and personality to an app. In this blog post, let’s learn how to show GIF images in Jetpack Compose.
Android doesn’t have default support for GIFs. Hence, we use the Coil library to add GIF support.
First of all, add the following code to the dependencies tag of the app module build.gradle file.
implementation("io.coil-kt:coil-compose:2.2.2")
implementation("io.coil-kt:coil-gif:2.2.2")
You can add gif images as given below.
val imageLoader = ImageLoader.Builder(LocalContext.current)
.components {
if (SDK_INT >= 28) {
add(ImageDecoderDecoder.Factory())
} else {
add(GifDecoder.Factory())
}
}
.build()
Image(
painter = rememberAsyncImagePainter(R.drawable.cat, imageLoader),
contentDescription = null,
modifier = Modifier.fillMaxSize()
)
As you see, first you need to construct the ImageLoader with the decoders. The Coil features two distinct decoders for decoding GIFs, GifDecoder and ImageDecoderDecoder.
GifDecoder is compatible with all API levels, but its performance may not be as fast as ImageDecoderDecoder. ImageDecoderDecoder utilizes the Android ImageDecoder API, which is only available on API 28 and above, but it is faster than GifDecoder.
You will get the following output.
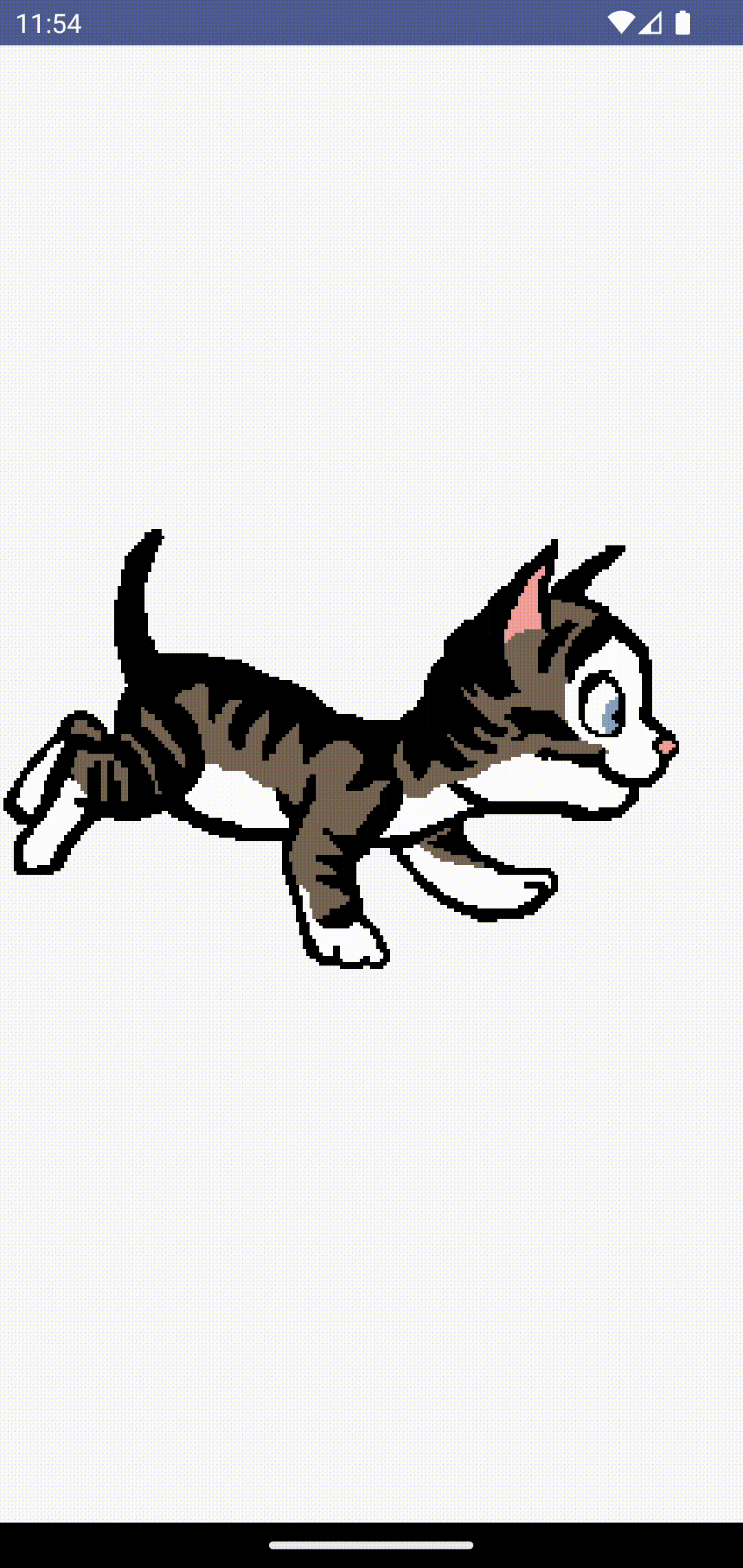
Following is the complete code for reference.
package com.example.myapplication
import android.os.Build.VERSION.SDK_INT
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.*
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.platform.LocalContext
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.foundation.Image
import coil.ImageLoader
import coil.compose.rememberAsyncImagePainter
import coil.decode.GifDecoder
import coil.decode.ImageDecoderDecoder
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
ImageExample()
}
}
}
}
}
@Composable
fun ImageExample() {
val imageLoader = ImageLoader.Builder(LocalContext.current)
.components {
if (SDK_INT >= 28) {
add(ImageDecoderDecoder.Factory())
} else {
add(GifDecoder.Factory())
}
}
.build()
Image(
painter = rememberAsyncImagePainter(R.drawable.cat, imageLoader),
contentDescription = null,
modifier = Modifier.fillMaxSize()
)
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
ImageExample()
}
}
That’s how you show gif images in Jetpack Compose.
One Comment